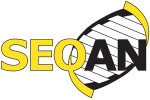 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
77 constexpr
aa20() noexcept =
default;
78 constexpr
aa20(
aa20 const &) noexcept =
default;
79 constexpr
aa20(
aa20 &&) noexcept =
default;
82 ~aa20() noexcept =
default;
131 ret[
'B'] = ret[
'D']; ret[
'b'] = ret[
'D'];
132 ret[
'J'] = ret[
'L']; ret[
'j'] = ret[
'L'];
133 ret[
'O'] = ret[
'L']; ret[
'o'] = ret[
'L'];
134 ret[
'U'] = ret[
'C']; ret[
'u'] = ret[
'C'];
135 ret[
'X'] = ret[
'S']; ret[
'x'] = ret[
'S'];
136 ret[
'Z'] = ret[
'E']; ret[
'z'] = ret[
'E'];
176 constexpr
aa20 operator""_aa20(
char const c) noexcept
200 for (
size_t i = 0; i < n; ++i)
201 r[i].assign_char(s[i]);
Provides seqan3::aminoacid_alphabet.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
static constexpr std::array< rank_type, 256 > char_to_rank
Char to value conversion table.
Definition: aa20.hpp:115
Provides seqan3::aminoacid_base.
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
static constexpr char_type rank_to_char[alphabet_size]
Value to char conversion table.
Definition: aa20.hpp:90
std::conditional_t< std::same_as< char, void >, char, char > char_type
The char representation; conditional needed to make semi alphabet definitions legal.
Definition: alphabet_base.hpp:60
~aa20() noexcept=default
Defaulted.
A CRTP-base that refines seqan3::alphabet_base and is used by the amino acids.
Definition: aminoacid_base.hpp:29
constexpr char_type to_lower(char_type const c) noexcept
Converts 'A'-'Z' to 'a'-'z' respectively; other characters are returned as is.
Definition: transform.hpp:81
The canonical amino acid alphabet.
Definition: aa20.hpp:61
constexpr aa20 & operator=(aa20 const &) noexcept=default
Defaulted.
constexpr aa20() noexcept=default
Defaulted.