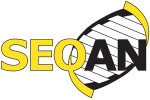 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
50 template <
typename derived_type,
size_t size,
typename char_t =
char>
54 static_assert(
size != 0,
"alphabet size must be >= 1");
99 return derived_type::rank_to_char[rank];
146 rank = derived_type::char_to_rank[static_cast<index_t>(c)];
147 return static_cast<derived_type &>(*
this);
167 assert(static_cast<size_t>(c) < static_cast<size_t>(
alphabet_size));
169 return static_cast<derived_type &>(*
this);
180 friend constexpr
bool operator==(derived_type
const lhs, derived_type
const rhs) noexcept
186 friend constexpr
bool operator!=(derived_type
const lhs, derived_type
const rhs) noexcept
192 friend constexpr
bool operator<(derived_type
const lhs, derived_type
const rhs) noexcept
198 friend constexpr
bool operator>(derived_type
const lhs, derived_type
const rhs) noexcept
204 friend constexpr
bool operator<=(derived_type
const lhs, derived_type
const rhs) noexcept
210 friend constexpr
bool operator>=(derived_type
const lhs, derived_type
const rhs) noexcept
232 template <
typename derived_type,
typename char_t>
266 return derived_type::char_value;
285 return static_cast<derived_type &>(*
this);
291 return static_cast<derived_type &>(*
this);
302 friend constexpr
bool operator==(derived_type
const, derived_type
const) noexcept
308 friend constexpr
bool operator!=(derived_type
const, derived_type
const) noexcept
314 friend constexpr
bool operator<(derived_type
const, derived_type
const) noexcept
320 friend constexpr
bool operator>(derived_type
const, derived_type
const) noexcept
326 friend constexpr
bool operator<=(derived_type
const, derived_type
const) noexcept
332 friend constexpr
bool operator>=(derived_type
const, derived_type
const) noexcept
339 #if SEQAN3_WORKAROUND_GCC_87113
340 bool _bug_workaround{};
constexpr friend bool operator<(derived_type const lhs, derived_type const rhs) noexcept
Checks whether the letter lhs is smaller than rhs.
Definition: alphabet_base.hpp:192
Provides C++20 additions to the type_traits header.
constexpr friend bool operator<=(derived_type const lhs, derived_type const rhs) noexcept
Checks whether the letter lhs is smaller than or equal to rhs.
Definition: alphabet_base.hpp:204
constexpr derived_type & assign_rank(rank_type const) noexcept
Assign from a numeric value.
Definition: alphabet_base.hpp:289
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
constexpr friend bool operator>(derived_type const lhs, derived_type const rhs) noexcept
Checks whether the letter lhs is greater than rhs.
Definition: alphabet_base.hpp:198
constexpr char_type to_char() const noexcept
Return the letter as a character of char_type.
Definition: alphabet_base.hpp:94
A CRTP-base that makes defining a custom alphabet easier.
Definition: alphabet_base.hpp:51
constexpr auto to_rank
Return the rank representation of a (semi-)alphabet object.
Definition: concept.hpp:142
constexpr friend bool operator<=(derived_type const, derived_type const) noexcept
Letters are always equal.
Definition: alphabet_base.hpp:326
constexpr friend bool operator!=(derived_type const, derived_type const) noexcept
Letters are never unequal.
Definition: alphabet_base.hpp:308
constexpr friend bool operator<(derived_type const, derived_type const) noexcept
One letter cannot be smaller than another.
Definition: alphabet_base.hpp:314
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
constexpr friend bool operator==(derived_type const lhs, derived_type const rhs) noexcept
Checks whether the letters lhs and rhs are equal.
Definition: alphabet_base.hpp:180
constexpr friend bool operator>(derived_type const, derived_type const) noexcept
One letter cannot be bigger than another.
Definition: alphabet_base.hpp:320
constexpr rank_type to_rank() const noexcept
Return the letter's numeric value (rank in the alphabet).
Definition: alphabet_base.hpp:270
constexpr friend bool operator!=(derived_type const lhs, derived_type const rhs) noexcept
Checks whether the letters lhs and rhs are unequal.
Definition: alphabet_base.hpp:186
detail::min_viable_uint_t< size - 1 > rank_type
The type of the alphabet when represented as a number (e.g. via to_rank()).
Definition: alphabet_base.hpp:62
constexpr alphabet_base() noexcept=default
Defaulted.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr friend bool operator>=(derived_type const, derived_type const) noexcept
Letters are always equal.
Definition: alphabet_base.hpp:332
constexpr friend bool operator==(derived_type const, derived_type const) noexcept
Letters are always equal.
Definition: alphabet_base.hpp:302
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
std::conditional_t< std::same_as< char_t, void >, char, char_t > char_type
The char representation; conditional needed to make semi alphabet definitions legal.
Definition: alphabet_base.hpp:60
Provides metaprogramming utilities for integer types.
SeqAn specific customisations in the standard namespace.
bool rank_type
The type of the alphabet when represented as a number (e.g. via to_rank()).
Definition: alphabet_base.hpp:242
constexpr friend bool operator>=(derived_type const lhs, derived_type const rhs) noexcept
Checks whether the letter lhs is bigger than or equal to rhs.
Definition: alphabet_base.hpp:210
constexpr derived_type & assign_rank(rank_type const c) noexcept
Assign from a numeric value.
Definition: alphabet_base.hpp:165
constexpr rank_type to_rank() const noexcept
Return the letter's numeric value (rank in the alphabet).
Definition: alphabet_base.hpp:116
Core alphabet concept and free function/type trait wrappers.