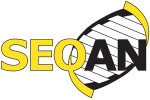 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
55 template <
typename derived_type, writable_semialphabet alphabet_type>
62 alphabet_base<derived_type,
63 alphabet_size<alphabet_type>,
64 detail::valid_template_spec_or_t<void, alphabet_char_t, alphabet_type>>>
72 alphabet_size<alphabet_type>,
73 detail::valid_template_spec_or_t<void, alphabet_char_t, alphabet_type>>>;
79 using char_type = detail::valid_template_spec_or_t<char, alphabet_char_t, alphabet_type>;
82 using phred_type = detail::valid_template_spec_or_t<int8_t, alphabet_phred_t, alphabet_type>;
98 requires std::is_class_v<alphabet_type>
106 requires !std::is_class_v<alphabet_type>
114 constexpr derived_type & operator=(alphabet_type
const & c) noexcept
116 if constexpr (std::is_class_v<alphabet_type>)
121 static_cast<derived_type &>(*this).on_update();
122 return static_cast<derived_type &>(*
this);
126 template <
typename indirect_assignable_type>
127 constexpr derived_type & operator=(indirect_assignable_type
const & c) noexcept
155 return operator=(tmp);
166 return operator=(tmp);
177 return operator=(tmp);
185 constexpr
operator alphabet_type() const noexcept
188 if constexpr (std::is_class_v<alphabet_type>)
209 template <
typename other_t>
213 constexpr
operator other_t() const noexcept
215 return operator alphabet_type();
257 return char_is_valid_for<alphabet_type>(c);
268 template <
typename t>
269 static constexpr
bool is_alphabet_comparable_with = !std::is_same_v<derived_type, t> &&
270 detail::weakly_equality_comparable_with<alphabet_type, t>;
274 template <
typename t>
275 friend constexpr
auto operator==(derived_type
const lhs, t
const rhs) noexcept
278 return (lhs.operator alphabet_type() == rhs);
282 template <
typename t>
283 friend constexpr
auto operator==(t
const lhs, derived_type
const rhs) noexcept
290 template <
typename t>
291 friend constexpr
auto operator!=(derived_type
const lhs, t
const rhs) noexcept
294 return !(lhs == rhs);
298 template <
typename t>
299 friend constexpr
auto operator!=(t
const lhs, derived_type
const rhs) noexcept
static constexpr auto alphabet_size
The alphabet size.
Definition: alphabet_proxy.hpp:143
constexpr friend auto operator==(derived_type const lhs, t const rhs) noexcept -> std::enable_if_t< is_alphabet_comparable_with< t >, bool >
Allow (in-)equality comparison with types that the emulated type is comparable with.
Definition: alphabet_proxy.hpp:275
Provides seqan3::alphabet_base.
constexpr auto assign_rank_to
Assign a rank to an alphabet object.
Definition: concept.hpp:238
Provides seqan3::nucleotide_alphabet.
constexpr derived_type & assign_phred(phred_type const c) noexcept
Assigns a phred.
Definition: alphabet_proxy.hpp:170
A concept that indicates whether an alphabet represents quality scores.
Provides various type traits on generic types.
constexpr auto to_char
Return the char representation of an alphabet object.
Definition: concept.hpp:320
Provides seqan3::type_list and auxiliary type traits.
A CRTP-base that makes defining a custom alphabet easier.
Definition: alphabet_base.hpp:51
constexpr auto to_rank
Return the rank representation of a (semi-)alphabet object.
Definition: concept.hpp:142
decltype(seqan3::to_rank(std::declval< semi_alphabet_type >())) alphabet_rank_t
The rank_type of the semi-alphabet; defined as the return type of seqan3::to_rank.
Definition: concept.hpp:151
constexpr friend auto operator!=(t const lhs, derived_type const rhs) noexcept -> std::enable_if_t< is_alphabet_comparable_with< t >, bool >
Allow (in-)equality comparison with types that the emulated type is comparable with.
Definition: alphabet_proxy.hpp:299
Quality alphabet concept.
constexpr derived_type & assign_rank(alphabet_rank_t< alphabet_type > const r) noexcept
Assigns a rank.
Definition: alphabet_proxy.hpp:151
A concept that indicates whether an alphabet represents nucleotides.
constexpr friend auto operator==(t const lhs, derived_type const rhs) noexcept -> std::enable_if_t< is_alphabet_comparable_with< t >, bool >
Allow (in-)equality comparison with types that the emulated type is comparable with.
Definition: alphabet_proxy.hpp:283
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr auto to_phred() const noexcept
Returns the phred score.
Definition: alphabet_proxy.hpp:234
The concept std::convertible_to<From, To> specifies that an expression of the type and value category...
static constexpr bool char_is_valid(char_type const c) noexcept
Delegate to the emulated type's validator.
Definition: alphabet_proxy.hpp:252
constexpr auto to_phred
The public getter function for the phred representation of a quality score.
Definition: concept.hpp:89
Subsumes std::semiregular and std::equality_comparable.
constexpr auto to_char() const noexcept
Returns the character.
Definition: alphabet_proxy.hpp:225
constexpr auto assign_phred_to
Assign a phred score to a quality alphabet object.
Definition: concept.hpp:189
The generic alphabet concept that covers most data types used in ranges.
Resolves to std::is_assignable_v<t>.
constexpr auto to_rank() const noexcept
Returns the rank.
Definition: alphabet_proxy.hpp:219
constexpr friend auto operator!=(derived_type const lhs, t const rhs) noexcept -> std::enable_if_t< is_alphabet_comparable_with< t >, bool >
Allow (in-)equality comparison with types that the emulated type is comparable with.
Definition: alphabet_proxy.hpp:291
constexpr auto assign_char_to
Assign a character to an alphabet object.
Definition: concept.hpp:416
constexpr auto complement
Return the complement of a nucleotide object.
Definition: concept.hpp:93
constexpr alphabet_type complement() const noexcept
Returns the complement.
Definition: alphabet_proxy.hpp:243
constexpr derived_type & assign_char(char_type const c) noexcept
Assigns a character.
Definition: alphabet_proxy.hpp:159
Refines seqan3::alphabet and adds assignability.
A concept that indicates whether a writable alphabet represents quality scores.
A CRTP-base that eases the definition of proxy types returned in place of regular alphabets.
Definition: alphabet_proxy.hpp:59