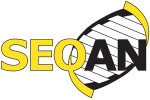 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
66 constexpr
cigar_op() noexcept =
default;
101 ret[rank_to_char[rnk] ] = rnk;
121 inline cigar_op operator""_cigar_op(
char const c) noexcept
constexpr cigar_op() noexcept=default
Defaulted.
Provides seqan3::alphabet_base.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
A CRTP-base that makes defining a custom alphabet easier.
Definition: alphabet_base.hpp:51
constexpr cigar_op & operator=(cigar_op const &) noexcept=default
Defaulted.
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
~cigar_op() noexcept=default
std::conditional_t< std::same_as< char, void >, char, char > char_type
The char representation; conditional needed to make semi alphabet definitions legal.
Definition: alphabet_base.hpp:60
The (extended) cigar operation alphabet of M,D,I,H,N,P,S,X,=.
Definition: cigar_op.hpp:52