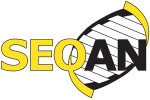 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
71 constexpr
dna3bs() noexcept =
default;
102 ret[ rank_to_char[rnk] ] = rnk;
103 ret[
to_lower(rank_to_char[rnk])] = rnk;
107 ret[
'C'] = ret[
'T']; ret[
'c'] = ret[
't'];
108 ret[
'U'] = ret[
'T']; ret[
'u'] = ret[
't'];
111 ret[
'R'] = ret[
'A']; ret[
'r'] = ret[
'A'];
112 ret[
'Y'] = ret[
'T']; ret[
'y'] = ret[
'T'];
113 ret[
'S'] = ret[
'T']; ret[
's'] = ret[
'T'];
114 ret[
'W'] = ret[
'A']; ret[
'w'] = ret[
'A'];
115 ret[
'K'] = ret[
'G']; ret[
'k'] = ret[
'G'];
116 ret[
'M'] = ret[
'A']; ret[
'm'] = ret[
'A'];
117 ret[
'B'] = ret[
'T']; ret[
'b'] = ret[
'T'];
118 ret[
'D'] = ret[
'A']; ret[
'd'] = ret[
'A'];
119 ret[
'H'] = ret[
'A']; ret[
'h'] = ret[
'A'];
120 ret[
'V'] = ret[
'A']; ret[
'v'] = ret[
'A'];
150 constexpr
dna3bs operator""_dna3bs(
char const c) noexcept
169 for (
size_t i = 0; i < n; ++i)
170 r[i].assign_char(s[i]);
constexpr dna3bs() noexcept=default
Defaulted.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
A CRTP-base that refines seqan3::alphabet_base and is used by the nucleotides.
Definition: nucleotide_base.hpp:40
The three letter reduced DNA alphabet for bisulfite sequencing mode (A,G,T(=C)).
Definition: dna3bs.hpp:55
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
Provides seqan3::nucleotide_base.
~dna3bs() noexcept=default
Defaulted.
constexpr char_type to_lower(char_type const c) noexcept
Converts 'A'-'Z' to 'a'-'z' respectively; other characters are returned as is.
Definition: transform.hpp:81
constexpr dna3bs & operator=(dna3bs const &) noexcept=default
Defaulted.