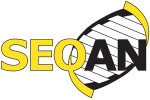 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
141 rank_table[
'.'] = 0u;
142 rank_table[
'('] = 1u;
143 rank_table[
')'] = 2u;
167 for (
size_t idx = 0ul; idx < len; ++idx)
168 vec[idx].assign_char(str[idx]);
constexpr dot_bracket3() noexcept=default
Defaulted.
constexpr bool is_pair_open() const noexcept
Check whether the character represents a rightward interaction in an RNA structure.
Definition: dot_bracket3.hpp:78
Provides seqan3::rna_structure_alphabet.
Provides seqan3::alphabet_base.
constexpr dot_bracket3 & operator=(dot_bracket3 const &) noexcept=default
Defaulted.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
A CRTP-base that makes defining a custom alphabet easier.
Definition: alphabet_base.hpp:51
static constexpr uint8_t max_pseudoknot_depth
The ability of this alphabet to represent pseudoknots, i.e. crossing interactions,...
Definition: dot_bracket3.hpp:103
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
constexpr std::optional< uint8_t > pseudoknot_id() const noexcept
Get an identifier for a pseudoknotted interaction, where opening and closing brackets of the same typ...
Definition: dot_bracket3.hpp:109
detail::min_viable_uint_t< size - 1 > rank_type
The type of the alphabet when represented as a number (e.g. via to_rank()).
Definition: alphabet_base.hpp:62
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
std::conditional_t< std::same_as< char, void >, char, char > char_type
The char representation; conditional needed to make semi alphabet definitions legal.
Definition: alphabet_base.hpp:60
~dot_bracket3() noexcept=default
The three letter RNA structure alphabet of the characters ".()".
Definition: dot_bracket3.hpp:51
constexpr bool is_pair_close() const noexcept
Check whether the character represents a leftward interaction in an RNA structure.
Definition: dot_bracket3.hpp:86
constexpr bool is_unpaired() const noexcept
Check whether the character represents an unpaired position in an RNA structure.
Definition: dot_bracket3.hpp:94
constexpr rank_type to_rank() const noexcept
Return the letter's numeric value (rank in the alphabet).
Definition: alphabet_base.hpp:116