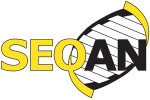 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
73 constexpr
dssp9() noexcept =
default;
74 constexpr
dssp9(
dssp9 const &) noexcept =
default;
78 ~dssp9() noexcept =
default;
87 'H',
'B',
'E',
'G',
'I',
'T',
'S',
'C',
'X'
104 ret[static_cast<rank_type>(rank_to_char[rnk])] = rnk;
129 for (
size_t idx = 0u; idx < len; ++idx)
130 vec[idx].assign_char(str[idx]);
143 constexpr
dssp9 operator""_dssp9(
char const ch) noexcept
Provides seqan3::alphabet_base.
The protein structure alphabet of the characters "HGIEBTSCX".
Definition: dssp9.hpp:60
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
A CRTP-base that makes defining a custom alphabet easier.
Definition: alphabet_base.hpp:51
~dssp9() noexcept=default
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
detail::min_viable_uint_t< size - 1 > rank_type
The type of the alphabet when represented as a number (e.g. via to_rank()).
Definition: alphabet_base.hpp:62
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr dssp9() noexcept=default
Defaulted.
constexpr dssp9 & operator=(dssp9 const &) noexcept=default
Defaulted.
Core alphabet concept and free function/type trait wrappers.