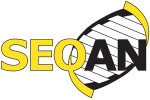 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
16 #include <range/v3/numeric/accumulate.hpp>
37 template <
typename ...errors_t>
39 requires
sizeof...(errors_t) <= 4 &&
52 template <
typename ..._errors_t>
53 static constexpr
bool check_consistency(_errors_t ...errors)
55 if constexpr (
sizeof...(errors) < 2)
61 return [] (
auto head,
auto ...tail) constexpr
63 using head_t = decltype(head);
64 if constexpr (((head_t::_id != decltype(tail)::_id) && ...))
65 return check_consistency(tail...);
72 static_assert(check_consistency(errors_t{}...),
73 "You may not use the same error specifier more than once.");
79 static constexpr detail::search_config_id
id{detail::search_config_id::max_error_rate};
119 requires
sizeof...(errors_t) > 0
123 detail::for_each([
this](
auto e)
126 }, std::forward<errors_t>(errors)...);
131 if (0.0 > error_elem || error_elem > 1.0)
153 max_error_rate() -> max_error_rate<>;
157 template <
typename ...errors_t>
158 max_error_rate(errors_t && ...) -> max_error_rate<remove_cvref_t<errors_t>...>;
constexpr max_error_rate(errors_t &&...errors)
Constructs the object from a set of error specifiers.
Definition: max_error_rate.hpp:117
Provides algorithms for meta programming, parameter packs and seqan3::type_list.
Provides seqan3::detail::configuration and utility functions.
Provides compatibility matrix for search configurations.
Provides seqan3::pipeable_config_element.
Adaptations of algorithms from the Ranges TS.
A special sub namespace for the search configurations.
~max_error_rate() noexcept=default
Destructor.
A configuration element for the maximum number of errors in percent of the query length across all er...
Definition: max_error_rate.hpp:45
Provides seqan3::views::slice.
constexpr max_error_rate() noexcept=default
Default constructor.
Adds pipe interface to configuration elements.
Definition: pipeable_config_element.hpp:30
std::array< double, 4 > value
The stored config value.
Definition: pipeable_config_element.hpp:33
constexpr max_error_rate & operator=(max_error_rate const &) noexcept=default
Copy assignment.
Provides the error types for maximum number of errors.
constexpr auto slice
A view adaptor that returns a half-open interval on the underlying range.
Definition: slice.hpp:141