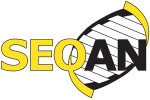 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
109 ret[ rank_to_char[rnk] ] = rnk;
110 ret[
to_lower(rank_to_char[rnk])] = rnk;
114 ret[
'U'] = ret[
'T']; ret[
'u'] = ret[
't'];
145 constexpr
sam_dna16 operator""_sam_dna16(
char const c) noexcept
165 for (
size_t i = 0; i < n; ++i)
166 r[i].assign_char(s[i]);
~sam_dna16() noexcept=default
Defaulted.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
constexpr sam_dna16() noexcept=default
Defaulted.
constexpr sam_dna16 & operator=(sam_dna16 const &) noexcept=default
Defaulted.
A CRTP-base that refines seqan3::alphabet_base and is used by the nucleotides.
Definition: nucleotide_base.hpp:40
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:140
A 16 letter DNA alphabet, containing all IUPAC symbols minus the gap and plus an equality sign ('=').
Definition: sam_dna16.hpp:45
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
Provides seqan3::nucleotide_base.
constexpr char_type to_lower(char_type const c) noexcept
Converts 'A'-'Z' to 'a'-'z' respectively; other characters are returned as is.
Definition: transform.hpp:81