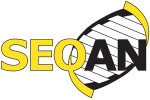 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
16 #include <type_traits>
18 #if SEQAN3_WITH_CEREAL
19 #include <cereal/types/array.hpp>
20 #endif // SEQAN3_WITH_CEREAL
43 template <
typename value_type_,
size_t capacity_>
48 static constexpr
bool is_noexcept = std::is_nothrow_copy_constructible_v<value_type_>;
66 using allocator_type = void;
91 data_{array}, sz{capacity_}
95 template <
size_t capacity2>
99 static_assert(capacity2 <= capacity_,
"You can only initialize from array that has smaller or equal capacity.");
100 std::ranges::copy(array, data_.
begin());
115 template <
size_t capacity2>
119 static_assert(capacity2 <= capacity_,
"You can only initialize from array that has smaller or equal capacity.");
120 std::ranges::copy(array, data_.
begin());
134 template <
typename ...other_value_type>
138 constexpr
small_vector(other_value_type... args) noexcept(is_noexcept) :
139 data_{args...}, sz{
sizeof...(other_value_type)}
141 static_assert(
sizeof...(other_value_type) <= capacity_,
"Value list must not exceed the capacity.");
159 template <std::forward_iterator begin_it_type,
typename end_it_type>
161 requires std::sentinel_for<end_it_type, begin_it_type> &&
164 constexpr
small_vector(begin_it_type begin_it, end_it_type end_it) noexcept(is_noexcept) :
183 template <std::ranges::input_range other_range_t>
185 requires !std::is_same_v<remove_cvref_t<other_range_t>,
small_vector>
188 explicit constexpr
small_vector(other_range_t && range) noexcept(is_noexcept) :
189 small_vector{std::ranges::begin(range), std::ranges::end(range)}
223 assign(std::ranges::begin(ilist), std::ranges::end(ilist));
240 assign(std::ranges::begin(ilist), std::ranges::end(ilist));
259 assign(std::ranges::begin(tmp), std::ranges::end(tmp));
275 template <std::ranges::input_range other_range_t>
279 constexpr
void assign(other_range_t && range) noexcept(is_noexcept)
281 assign(std::ranges::begin(range), std::ranges::end(range));
299 template <std::forward_iterator begin_it_type,
typename end_it_type>
301 requires std::sentinel_for<end_it_type, begin_it_type> &&
304 constexpr
void assign(begin_it_type begin_it, end_it_type end_it) noexcept(is_noexcept)
373 throw std::out_of_range{
"Trying to access element behind the last in small_vector."};
383 throw std::out_of_range{
"Trying to access element behind the last in small_vector."};
458 return (*
this)[
size()-1];
465 return (*
this)[
size()-1];
495 constexpr
bool empty() const noexcept
599 return insert(pos, 1, value);
621 return insert(pos, std::ranges::begin(tmp), std::ranges::end(tmp));
644 template <std::forward_iterator begin_it_type,
typename end_it_type>
646 requires std::sentinel_for<end_it_type, begin_it_type> &&
651 auto const pos_as_num = std::ranges::distance(
cbegin(), pos);
652 auto const length = std::ranges::distance(begin_it, end_it);
654 assert(pos_as_num + length <=
capacity());
659 for (
size_type i = sz + length - 1; i > pos_as_num + length - 1; --i)
660 data_[i] = data_[i - length];
662 std::ranges::copy(begin_it, end_it, &data_[pos_as_num]);
664 return begin() + pos_as_num;
684 return insert(pos, ilist.begin(), ilist.end());
707 if (begin_it >= end_it)
708 return begin() + std::ranges::distance(
cbegin(), end_it);
710 size_type const length = std::ranges::distance(begin_it, end_it);
711 auto out_it =
begin() + std::ranges::distance(
cbegin(), begin_it);
713 while (end_it !=
cend())
714 *(out_it++) = *(end_it++);
717 return begin() + std::ranges::distance(
cbegin(), begin_it);
740 return erase(pos, pos + 1);
758 assert(sz < capacity_);
798 assert(
count <= capacity_);
808 assert(
count <= capacity_);
809 for (
size_t i = sz; i <
count; ++i)
832 rhs.data_ = tmp.data_;
871 template <
size_t cap2>
873 requires cap2 <= capacity_
877 return std::ranges::equal(lhs, rhs);
881 template <
size_t cap2>
883 requires cap2 <= capacity_
887 return !(lhs == rhs);
891 template <
size_t cap2>
893 requires cap2 <= capacity_
897 for (
size_t i = 0; i <
std::min(lhs.size(), rhs.size()); ++i)
900 else if (lhs[i] < rhs[i])
902 return lhs.size() < rhs.size();
906 template <
size_t cap2>
908 requires cap2 <= capacity_
912 for (
size_t i = 0; i <
std::min(lhs.size(), rhs.size()); ++i)
915 else if (lhs[i] > rhs[i])
917 return lhs.size() > rhs.size();
921 template <
size_t cap2>
923 requires cap2 <= capacity_
931 template <
size_t cap2>
933 requires cap2 <= capacity_
957 template <cereal_archive archive_t>
958 void CEREAL_SERIALIZE_FUNCTION_NAME(archive_t & archive)
970 template <
size_t capacity2,
typename value_type>
972 small_vector(
const value_type (&array)[capacity2]) -> small_vector<value_type, capacity2>;
constexpr iterator erase(const_iterator begin_it, const_iterator end_it) noexcept
Removes specified elements from the container.
Definition: small_vector.hpp:705
constexpr size_type size() const noexcept
Returns the number of elements in the container, i.e. std::distance(begin(), end()).
Definition: small_vector.hpp:511
A constexpr vector implementation with dynamic size at compile time.
Definition: small_vector.hpp:44
constexpr const_reference operator[](size_type const i) const noexcept
Return the i-th element.
Definition: small_vector.hpp:410
constexpr iterator insert(const_iterator pos, value_type const value) noexcept(is_noexcept)
Inserts value before position in the container.
Definition: small_vector.hpp:597
small_vector(const value_type(&array)[capacity2]) -> small_vector< value_type, capacity2 >
Deducts the size and value type from an built-in array on construction.
constexpr const_iterator begin() const noexcept
Returns the begin to the string.
Definition: small_vector.hpp:321
constexpr small_vector & operator=(std::initializer_list< value_type > ilist) noexcept(is_noexcept)
Assign from std::initializer_list.
Definition: small_vector.hpp:221
constexpr void pop_back() noexcept
Removes the last element of the container.
Definition: small_vector.hpp:777
The std::constructible_from concept specifies that a variable of type T can be initialized with the g...
constexpr friend bool operator==(small_vector const &lhs, small_vector< value_type, cap2 > const &rhs) noexcept
Performs element-wise comparison.
Definition: small_vector.hpp:875
constexpr const_iterator end() const noexcept
Returns iterator past the end of the vector.
Definition: small_vector.hpp:339
typename reference< t >::type reference_t
Shortcut for seqan3::reference (transformation_trait shortcut).
Definition: pre.hpp:77
constexpr const_iterator cend() const noexcept
Returns iterator past the end of the vector.
Definition: small_vector.hpp:345
constexpr friend bool operator<(small_vector const &lhs, small_vector< value_type, cap2 > const &rhs) noexcept
Performs element-wise comparison.
Definition: small_vector.hpp:895
const_reference at(size_type const i) const
Return the i-th element.
Definition: small_vector.hpp:379
Provides seqan3::type_list and auxiliary type traits.
value_type * iterator
The iterator type.
Definition: small_vector.hpp:57
constexpr value_type * data() noexcept
Direct access to the underlying array.
Definition: small_vector.hpp:469
constexpr void swap(small_vector &rhs) noexcept(is_noexcept)
Swap contents with another instance.
Definition: small_vector.hpp:825
constexpr void resize(size_type const count) noexcept
Resizes the container to contain count elements.
Definition: small_vector.hpp:796
constexpr void resize(size_type const count, value_type const value) noexcept
Resizes the container to contain count elements.
Definition: small_vector.hpp:806
constexpr iterator insert(const_iterator pos, std::initializer_list< value_type > const &ilist) noexcept(is_noexcept)
Inserts elements from initializer list before position in the container.
Definition: small_vector.hpp:682
constexpr void push_back(value_type const value) noexcept
Appends the given element value to the end of the container.
Definition: small_vector.hpp:756
constexpr void assign(other_range_t &&range) noexcept(is_noexcept)
Assign from a different range.
Definition: small_vector.hpp:279
constexpr iterator end() noexcept
Returns iterator past the end of the vector.
Definition: small_vector.hpp:333
constexpr small_vector() noexcept=default
Defaulted.
constexpr size_type capacity() const noexcept
Returns the number of elements that the container is able to hold and resolves to capacity_.
Definition: small_vector.hpp:546
constexpr void reserve(size_type) const noexcept
Since the capacity is fixed on compile time, this is a no-op.
Definition: small_vector.hpp:552
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
constexpr friend bool operator!=(small_vector const &lhs, small_vector< value_type, cap2 > const &rhs) noexcept
Performs element-wise comparison.
Definition: small_vector.hpp:885
constexpr small_vector(value_type const (&array)[capacity2]) noexcept(is_noexcept)
Construct from a (smaller or equally sized) built in array over the same value type.
Definition: small_vector.hpp:116
constexpr void swap(small_vector &&rhs) noexcept(is_noexcept)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: small_vector.hpp:837
Provides seqan3::views::repeat_n.
constexpr iterator begin() noexcept
Returns the begin to the string.
Definition: small_vector.hpp:315
constexpr iterator insert(const_iterator pos, begin_it_type begin_it, end_it_type end_it) noexcept(is_noexcept)
Inserts elements from range [begin_it, end_it) before position in the container.
Definition: small_vector.hpp:649
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr small_vector(begin_it_type begin_it, end_it_type end_it) noexcept(is_noexcept)
Construct from two iterators.
Definition: small_vector.hpp:164
constexpr friend void swap(small_vector &lhs, small_vector &rhs) noexcept(is_noexcept)
Swap contents with another instance.
Definition: small_vector.hpp:856
constexpr reference operator[](size_type const i) noexcept
Return the i-th element.
Definition: small_vector.hpp:403
constexpr small_vector(size_type n, value_type value) noexcept(is_noexcept)
Construct with n times value.
Definition: small_vector.hpp:204
ptrdiff_t difference_type
The difference_type type.
Definition: small_vector.hpp:59
constexpr const_reference back() const noexcept
Return the last element.
Definition: small_vector.hpp:462
constexpr const_iterator cbegin() const noexcept
Returns the begin to the string.
Definition: small_vector.hpp:327
constexpr void assign(size_type const count, value_type const value) noexcept(is_noexcept)
Assign with count times value.
Definition: small_vector.hpp:255
constexpr void shrink_to_fit() const noexcept
Since the capacity is fixed on compile time, this is a no-op.
Definition: small_vector.hpp:558
constexpr friend bool operator>(small_vector const &lhs, small_vector< value_type, cap2 > const &rhs) noexcept
Performs element-wise comparison.
Definition: small_vector.hpp:910
reference at(size_type const i)
Return the i-th element.
Definition: small_vector.hpp:369
const value_type & const_reference
The const_reference type.
Definition: small_vector.hpp:56
constexpr friend void swap(small_vector &&lhs, small_vector &&rhs) noexcept(is_noexcept)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: small_vector.hpp:862
constexpr iterator erase(const_iterator pos) noexcept
Removes specified elements from the container.
Definition: small_vector.hpp:738
detail::min_viable_uint_t< capacity_ > size_type
The size_type type.
Definition: small_vector.hpp:60
Provides metaprogramming utilities for integer types.
constexpr bool empty() const noexcept
Checks whether the container is empty.
Definition: small_vector.hpp:495
SeqAn specific customisations in the standard namespace.
constexpr void assign(std::initializer_list< value_type > ilist) noexcept(is_noexcept)
Assign from std::initializer_list.
Definition: small_vector.hpp:238
constexpr const_reference front() const noexcept
Return the first element. Calling front on an empty container is undefined.
Definition: small_vector.hpp:436
constexpr size_type max_size() const noexcept
Returns the maximum number of elements the container is able to hold and resolves to capacity_.
Definition: small_vector.hpp:530
Adaptions of concepts from the Cereal library.
value_type & reference
The reference type.
Definition: small_vector.hpp:55
constexpr auto repeat_n
A view factory that repeats a given value n times.
Definition: repeat_n.hpp:94
char value_type
The value_type type.
Definition: small_vector.hpp:54
constexpr void assign(begin_it_type begin_it, end_it_type end_it) noexcept(is_noexcept)
Assign from pair of iterators.
Definition: small_vector.hpp:304
constexpr iterator insert(const_iterator pos, size_type const count, value_type const value) noexcept(is_noexcept)
Inserts count copies of value before position in the container.
Definition: small_vector.hpp:618
constexpr friend bool operator>=(small_vector const &lhs, small_vector< value_type, cap2 > const &rhs) noexcept
Performs element-wise comparison.
Definition: small_vector.hpp:935
constexpr const value_type * data() const noexcept
Direct access to the underlying array.
Definition: small_vector.hpp:475
constexpr reference back() noexcept
Return the last element.
Definition: small_vector.hpp:455
constexpr friend bool operator<=(small_vector const &lhs, small_vector< value_type, cap2 > const &rhs) noexcept
Performs element-wise comparison.
Definition: small_vector.hpp:925
constexpr small_vector(other_range_t &&range) noexcept(is_noexcept)
Construct from a different range.
Definition: small_vector.hpp:188
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134
constexpr reference front() noexcept
Return the first element. Calling front on an empty container is undefined.
Definition: small_vector.hpp:429
constexpr void clear() noexcept
Removes all elements from the container.
Definition: small_vector.hpp:577
value_type const * const_iterator
The const_iterator type.
Definition: small_vector.hpp:58