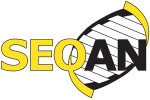 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
31 namespace seqan3::detail
35 template <
typename type>
48 template <
typename u
int_type>
50 requires seqan3::detail::is_uint_adaptation_v<uint_type>
62 static constexpr
auto to_char(uint_type
const intgr) noexcept
65 return static_cast<char>(intgr);
67 return static_cast<char16_t>(intgr);
69 return static_cast<char32_t>(intgr);
76 static constexpr uint_type
to_rank(uint_type
const intgr) noexcept
86 static constexpr uint_type &
assign_char_to(decltype(
to_char(uint_type{}))
const chr, uint_type & intgr) noexcept
96 static constexpr uint_type &
assign_rank_to(uint_type
const intgr2, uint_type & intgr) noexcept
98 return intgr = intgr2;
A type that can be specialised to provide customisation point implementations so that third party typ...
Definition: concept.hpp:46
static constexpr uint_type & assign_rank_to(uint_type const intgr2, uint_type &intgr) noexcept
Assign a rank to to the uint (same as calling =).
Definition: uint.hpp:96
constexpr auto to_char
Return the char representation of an alphabet object.
Definition: concept.hpp:320
static constexpr uint_type to_rank(uint_type const intgr) noexcept
Converting uint to rank is a no-op (it will just return the value you pass in).
Definition: uint.hpp:76
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
static constexpr auto to_char(uint_type const intgr) noexcept
Converting uint to char casts to a character type of same size.
Definition: uint.hpp:62
Provides metaprogramming utilities for integer types.
static constexpr uint_type & assign_char_to(decltype(to_char(uint_type{})) const chr, uint_type &intgr) noexcept
Assign from a character type via implicit or explicit cast.
Definition: uint.hpp:86
constexpr auto alphabet_size
A type trait that holds the size of a (semi-)alphabet.
Definition: concept.hpp:706
Core alphabet concept and free function/type trait wrappers.
A namespace for third party and standard library specialisations of SeqAn customisation points.
Definition: char.hpp:42