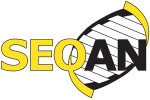 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
82 template <
typename val
idator_type>
128 if (!((cmp <= max) && (cmp >= min)))
138 template <std::ranges::forward_range range_type>
144 std::for_each(range.begin(), range.end(), [&] (
auto cmp) { (*this)(cmp); });
150 return detail::to_string(
"Value must be in range [", min,
",", max,
"].");
178 template <
typename option_value_t>
200 template <std::ranges::forward_range range_type>
215 template <
typename ...option_types>
221 (values.
emplace_back(std::forward<option_types>(opts)), ...);
240 template <std::ranges::forward_range range_type>
246 std::for_each(std::ranges::begin(range), std::ranges::end(range), [&] (
auto cmp) { (*this)(cmp); });
252 return detail::to_string(
"Value must be one of ",
std::views::all(values),
".");
267 value_list_validator(option_types...) -> value_list_validator<double>;
270 template <std::ranges::forward_range range_type>
274 value_list_validator(range_type && rng) -> value_list_validator<double>;
277 template <
typename ...option_types>
281 value_list_validator(option_types...) -> value_list_validator<
std::
string>;
284 template <
std::ranges::forward_range range_type>
288 value_list_validator(range_type && rng) -> value_list_validator<
std::
string>;
291 template <typename ...option_types>
292 value_list_validator(option_types...) -> value_list_validator<
seqan3::pack_traits::
front<option_types...>>;
295 template <
std::ranges::forward_range range_type>
296 value_list_validator(range_type && rng) -> value_list_validator<
std::ranges::range_value_t<range_type>>;
345 template <std::ranges::forward_range range_type>
351 std::for_each(v.begin(), v.end(), [&] (
auto cmp) { this->operator()(cmp); });
364 if (extensions.empty())
370 " has no extension. Expected one of the following valid"
371 " extensions:", extensions,
"!")};
387 extensions,
"! Got ", drop_less_ext,
" instead!")};
404 if (static_cast<bool>(ec))
414 if (!file.is_open() || !file.good())
428 detail::safe_filesystem_entry file_guard{path};
430 bool is_open = file.is_open();
431 bool is_good = file.good();
434 if (!is_good || !is_open)
466 template <
typename file_t =
void>
472 "Expected either a template type with a static member called valid_formats (a file type) or void.");
523 using file_validator_base::operator();
556 return detail::to_string(
"Valid input file formats: [",
584 template <
typename file_t =
void>
590 "Expected either a template type with a static member called valid_formats (a file type) or void.");
627 using file_validator_base::operator();
659 return detail::to_string(
"Valid output file formats: [",
700 using file_validator_base::operator();
733 return detail::to_string(
"An existing, readable path for the input directory.");
772 using file_validator_base::operator();
786 if (static_cast<bool>(ec))
793 detail::safe_filesystem_entry dir_guard{dir};
795 dir_guard.remove_all();
815 return detail::to_string(
"A valid path for the output directory.");
866 template <std::ranges::forward_range range_type>
872 std::for_each(v.begin(), v.end(), [&] (
auto cmp) { (*this)(cmp); });
878 return detail::to_string(
"Value must match the pattern '", pattern,
"'.");
899 template <
typename option_value_t>
900 struct default_validator
903 using option_value_type = option_value_t;
906 void operator()(option_value_t
const & )
const noexcept
927 template <val
idator val
idator1_type, val
idator val
idator2_type>
931 class validator_chain_adaptor
940 validator_chain_adaptor() =
delete;
941 validator_chain_adaptor(validator_chain_adaptor
const & pf) =
default;
942 validator_chain_adaptor & operator=(validator_chain_adaptor
const & pf) =
default;
943 validator_chain_adaptor(validator_chain_adaptor &&) =
default;
944 validator_chain_adaptor & operator=(validator_chain_adaptor &&) =
default;
950 validator_chain_adaptor(validator1_type vali1_, validator2_type vali2_) :
955 ~validator_chain_adaptor() =
default;
966 template <
typename cmp_type>
979 return detail::to_string(vali1.get_help_page_message(),
" ", vali2.get_help_page_message());
984 validator1_type vali1;
986 validator2_type vali2;
1018 template <val
idator val
idator1_type, val
idator val
idator2_type>
1023 auto operator|(validator1_type && vali1, validator2_type && vali2)
1025 return detail::validator_chain_adaptor{std::forward<validator1_type>(vali1),
1026 std::forward<validator2_type>(vali2)};
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:250
virtual void operator()(std::filesystem::path const &file) const override
Tests whether path is does not already exists and is writable.
Definition: validators.hpp:634
Provides parser related exceptions.
std::string option_value_type
Type of values that are tested by validator.
Definition: validators.hpp:840
Provides seqan3::detail::safe_filesystem_entry.
Provides various transformation trait base templates and shortcuts.
Provides seqan3::views::drop.
void operator()(range_type const &range) const
Tests whether every element in range lies inside values.
Definition: validators.hpp:244
A validator that checks if a matches a regular expression pattern.
Definition: validators.hpp:836
The std::constructible_from concept specifies that a variable of type T can be initialized with the g...
Provides various type traits on generic types.
const auto move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
Adaptations of concepts from the standard library.
This header includes C++17 filesystem support and imports it into namespace seqan3::filesystem (indep...
T is_regular_file(T... args)
A validator that checks whether a number is inside a given range.
Definition: validators.hpp:108
regex_validator(std::string const &pattern_)
Constructing from a vector.
Definition: validators.hpp:845
A validator that checks if a given path is a valid output directory.
Definition: validators.hpp:751
Adaptations of algorithms from the Ranges TS.
auto operator|(validator1_type &&vali1, validator2_type &&vali2)
Enables the chaining of validators.
Definition: validators.hpp:1023
~value_list_validator()=default
Defaulted.
T current_exception(T... args)
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:876
std::vector< std::string > extensions
Stores the extensions.
Definition: validators.hpp:441
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
using option_value_type
The type of value on which the validator is called on.
A validator that checks whether a value is inside a list of valid values.
Definition: validators.hpp:179
value_list_validator()=default
Defaulted.
An abstract base class for the file and directory validators.
Definition: validators.hpp:311
T has_extension(T... args)
const auto to_lower
A view that calls seqan3::to_lower() on each element in the input range.
Definition: to_lower.hpp:66
T is_directory(T... args)
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
T throw_with_nested(T... args)
void operator()(option_value_type const &cmp) const
Tests whether cmp lies inside values.
Definition: validators.hpp:229
file_validator_base()=default
Defaulted.
value_list_validator & operator=(value_list_validator const &)=default
Defaulted.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
arithmetic_range_validator(option_value_type const min_, option_value_type const max_)
The constructor.
Definition: validators.hpp:118
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
constexpr ptrdiff_t find_if
Get the index of the first type in a pack that satisfies the given predicate.
Definition: traits.hpp:175
Subsumes std::movable, std::copy_constructible, and requires that the type be std::assignable_from bo...
virtual ~output_directory_validator()=default
Virtual Destructor.
The concept std::convertible_to<From, To> specifies that an expression of the type and value category...
Provides seqan3::views::to_lower.
typename decltype(detail::front< pack_t... >())::type front
Return the first type from the type pack.
Definition: traits.hpp:240
virtual void operator()(std::filesystem::path const &dir) const override
Tests whether path is writable.
Definition: validators.hpp:779
constexpr detail::search_mode_all all
Configuration element to receive all hits within the error bounds.
Definition: mode.hpp:43
output_file_validator()
Default constructor.
Definition: validators.hpp:600
option_value_t option_value_type
Type of values that are tested by validator.
Definition: validators.hpp:183
Provides seqan3::views::join.
output_directory_validator()=default
Defaulted.
Adaptations of concepts from the Ranges TS.
T emplace_back(T... args)
constexpr auto drop
A view adaptor that returns all elements after n from the underlying range (or an empty range if the ...
Definition: drop.hpp:168
void operator()(range_type const &v) const
Tests whether every filename in list v matches the pattern.
Definition: validators.hpp:870
virtual ~output_file_validator()=default
Virtual Destructor.
SeqAn specific customisations in the standard namespace.
Argument parser exception that is thrown whenever there is an error while parsing the command line ar...
Definition: exceptions.hpp:37
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:148
output_directory_validator & operator=(output_directory_validator const &)=default
Defaulted.
The concept for option validators passed to add_option/positional_option.
T create_directory(T... args)
void operator()(option_value_type const &cmp) const
Tests whether cmp lies inside [min, max].
Definition: validators.hpp:126
Auxiliary for pretty printing of exception messages.
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:657
A type that satisfies std::is_arithmetic_v<t>.
void operator()(option_value_type const &cmp) const
Tests whether cmp lies inside values.
Definition: validators.hpp:853
void validate_readability(std::filesystem::path const &path) const
Checks if the given path is readable.
Definition: validators.hpp:397
output_file_validator & operator=(output_file_validator const &)=default
Defaulted.
void validate_filename(std::filesystem::path const &path) const
Validates the given filename path based on the specified extensions.
Definition: validators.hpp:361
void operator()(option_value_type const &cmp) const
Validates the value 'cmp' and throws a seqan3::validation_failed on failure.
T rethrow_exception(T... args)
Provides various utility functions.
Provides traits for seqan3::type_list.
void operator()(range_type const &range) const
Tests whether every element in range lies inside [min, max].
Definition: validators.hpp:142
Specifies whether the given callable is invocable with the given arguments.
A validator that checks if a given path is a valid output file.
Definition: validators.hpp:585
output_file_validator(std::vector< std::string > extensions)
Constructs from a given collection of valid extensions.
Definition: validators.hpp:613
double option_value_type
The type of value that this validator invoked upon.
Definition: validators.hpp:112
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:813
void validate_writeability(std::filesystem::path const &path) const
Checks if the given path is writable.
Definition: validators.hpp:425
value_list_validator(range_type rng)
Constructing from a range.
Definition: validators.hpp:204
std::string option_value_type
Type of values that are tested by validator.
Definition: validators.hpp:316
void operator()(range_type const &v) const
Tests whether every path in list v passes validation. See operator()(option_value_type const & value)...
Definition: validators.hpp:349