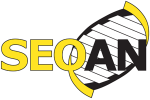 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
17 #include <type_traits>
68 template <
typename value_t,
size_t alignment_v = __STDCPP_DEFAULT_NEW_ALIGNMENT__>
98 template <
class other_value_type,
size_t other_alignment>
141 size_t bytes_to_allocate = n *
sizeof(
value_type);
142 if constexpr (
alignment <= __STDCPP_DEFAULT_NEW_ALIGNMENT__)
143 return static_cast<pointer>(::operator
new(bytes_to_allocate));
176 size_t bytes_to_deallocate = n *
sizeof(
value_type);
177 if constexpr (
alignment <= __STDCPP_DEFAULT_NEW_ALIGNMENT__)
178 ::
operator delete(p, bytes_to_deallocate);
193 template <
typename new_value_type>
205 template <
class value_type2,
size_t alignment2>
213 template <
class value_type2,
size_t alignment2>
value_t value_type
The value type of the allocation.
Definition: aligned_allocator.hpp:76
constexpr bool operator!=(aligned_allocator< value_type2, alignment2 > const &) noexcept
Returns false if the memory-alignment mismatches.
Definition: aligned_allocator.hpp:214
typename std::pointer_traits< pointer >::difference_type difference_type
The difference type of the allocation.
Definition: aligned_allocator.hpp:80
~aligned_allocator()=default
Defaulted.
pointer allocate(size_type const n) const
Allocates sufficiently large memory to hold n many elements of value_type.
Definition: aligned_allocator.hpp:135
aligned_allocator()=default
Defaulted.
aligned_allocator & operator=(aligned_allocator &&)=default
Defaulted.
aligned_allocator(aligned_allocator &&)=default
Defaulted.
static constexpr size_t other_alignment
The alignment for the rebound allocator.
Definition: aligned_allocator.hpp:197
Allocates uninitialized storage whose memory-alignment is specified by alignment.
Definition: aligned_allocator.hpp:70
value_type * pointer
The pointer type of the allocation.
Definition: aligned_allocator.hpp:78
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
constexpr bool operator==(aligned_allocator< value_type2, alignment2 > const &) noexcept
Returns true if the memory-alignment matches.
Definition: aligned_allocator.hpp:207
aligned_allocator & operator=(aligned_allocator const &)=default
Defaulted.
The aligned_allocator member template class aligned_allocator::rebind provides a way to obtain an all...
Definition: aligned_allocator.hpp:195
aligned_allocator(aligned_allocator const &)=default
Defaulted.
constexpr aligned_allocator(aligned_allocator< other_value_type, other_alignment > const &) noexcept
Copy constructor with different value type and alignment.
Definition: aligned_allocator.hpp:99
static constexpr size_t alignment
The memory-alignment of the allocation.
Definition: aligned_allocator.hpp:73
void deallocate(pointer const p, size_type const n) const noexcept
Deallocates the storage referenced by the pointer p, which must be a pointer obtained by an earlier c...
Definition: aligned_allocator.hpp:174