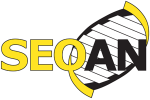 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
59 struct argument_parsing<t &> : argument_parsing<t>
63 struct argument_parsing<t const &> : argument_parsing<t>
69 namespace seqan3::detail::adl_only
82 template <
typename option_t>
83 struct enumeration_names_fn
87 seqan3::is_constexpr_default_constructible_v<std::remove_cvref_t<option_t>>,
95 template <
typename dummy =
int>
99 { impl(priority_tag<1>{}, s_option_t{}, dummy{}) };
100 std::same_as<decltype(impl(priority_tag<1>{}, s_option_t{}, dummy{})),
104 auto operator()()
const
106 return impl(priority_tag<1>{}, s_option_t{});
151 template <
typename option_type>
153 requires requires { { detail::adl_only::enumeration_names_fn<option_type>{}() }; }
168 template <
typename option_type>
172 { seqan3::enumeration_names<option_type> };
186 template <
typename option_type>
206 template <
typename char_t,
typename option_type>
210 inline debug_stream_type<char_t> &
operator<<(debug_stream_type<char_t> & s, option_type && op)
212 for (
auto & [key, value] : enumeration_names<option_type>)
218 return s <<
"<UNKNOWN_VALUE>";
option_spec
Used to further specify argument_parser options/flags.
Definition: auxiliary.hpp:233
Provides C++20 additions to the type_traits header.
#define SEQAN3_CPO_IMPL(PRIO, TERM)
A macro that helps defining the overload set of a customisation point.
Definition: customisation_point.hpp:45
@ DEFAULT
The default were no checking or special displaying is happening.
Definition: auxiliary.hpp:234
Provides various type traits on generic types.
Provides seqan3::debug_stream and related types.
auto const enumeration_names
Return a conversion map from std::string_view to option_type.
Definition: auxiliary.hpp:155
@ REQUIRED
Definition: auxiliary.hpp:235
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
Checks whether the free function seqan3::enumeration_names can be called on the type.
Checks whether the the type can be used in an add_(positional_)option call on the argument parser.
The identity transformation (a transformation_trait that returns the input).
debug_stream_type< char_t > & operator<<(debug_stream_type< char_t > &stream, alignment_t &&alignment)
Stream operator for alignments, which are represented as tuples of aligned sequences.
Definition: debug_stream_alignment.hpp:103
@ HIDDEN
Definition: auxiliary.hpp:244
A type that can be specialised to provide customisation point implementations for the seqan3::argumen...
Definition: auxiliary.hpp:51
@ ADVANCED
Definition: auxiliary.hpp:240
A namespace for third party and standard library specialisations of SeqAn customisation points.
Definition: char.hpp:44
Helper utilities for defining customisation point objects.