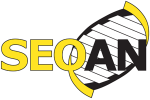 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
15 #include <type_traits>
17 #include <sdsl/int_vector.hpp>
61 template <writable_semialphabet alphabet_type>
63 requires std::regular<alphabet_type>
69 static constexpr
size_t bits_per_letter = detail::ceil_log2(alphabet_size<alphabet_type>);
71 static_assert(bits_per_letter <= 64,
"alphabet must be representable in at most 64bit.");
74 using data_type = sdsl::int_vector<bits_per_letter>;
81 class reference_proxy_type :
public alphabet_proxy<reference_proxy_type, alphabet_type>
90 std::ranges::range_reference_t<data_type> internal_proxy;
93 constexpr
void on_update() noexcept
95 internal_proxy =
static_cast<base_t &
>(*this).
to_rank();
100 using base_t::operator=;
105 reference_proxy_type() =
delete;
107 constexpr reference_proxy_type(reference_proxy_type
const &) noexcept =
default;
108 constexpr reference_proxy_type(reference_proxy_type &&) noexcept =
default;
109 constexpr reference_proxy_type &
operator=(reference_proxy_type
const &) noexcept =
default;
110 constexpr reference_proxy_type &
operator=(reference_proxy_type &&) noexcept =
default;
111 ~reference_proxy_type() noexcept =
default;
114 reference_proxy_type(std::ranges::range_reference_t<data_type>
const &
internal) noexcept :
115 internal_proxy{
internal}
117 static_cast<base_t &
>(*this).assign_rank(
internal);
125 template <
typename t>
126 requires std::is_same_v<std::ranges::range_value_t<std::remove_cvref_t<t>>, alphabet_type>
127 static constexpr
bool has_same_value_type_v =
true;
141 using iterator = detail::random_access_iterator<bitcompressed_vector>;
143 using const_iterator = detail::random_access_iterator<bitcompressed_vector const>;
152 using allocator_type = void;
178 template <std::ranges::input_range other_range_t>
180 requires has_same_value_type_v<other_range_t>
217 template <std::forward_iterator begin_iterator_type,
typename end_iterator_type>
220 requires std::sentinel_for<end_iterator_type, begin_iterator_type> &&
221 std::common_reference_with<std::iter_value_t<begin_iterator_type>,
value_type>
272 template <std::ranges::input_range other_range_t>
275 requires std::common_reference_with<std::ranges::range_value_t<other_range_t>,
value_type>
315 template <std::forward_iterator begin_iterator_type,
typename end_iterator_type>
316 void assign(begin_iterator_type begin_it, end_iterator_type end_it)
318 requires std::sentinel_for<end_iterator_type, begin_iterator_type> &&
319 std::common_reference_with<std::iter_value_t<begin_iterator_type>,
value_type>
428 throw std::out_of_range{
"Trying to access element behind the last in bitcompressed_vector."};
438 throw std::out_of_range{
"Trying to access element behind the last in bitcompressed_vector."};
513 return (*
this)[
size()-1];
520 return (*
this)[
size()-1];
538 constexpr data_type
const &
raw_data() const noexcept
595 return data.max_size();
615 return data.capacity();
638 data.reserve(new_cap);
658 data.shrink_to_fit();
701 return insert(pos, 1, value);
727 data.insert(data.begin() + pos_as_num,
count,
to_rank(value));
729 return begin() + pos_as_num;
756 template <std::forward_iterator begin_iterator_type,
typename end_iterator_type>
759 requires std::sentinel_for<end_iterator_type, begin_iterator_type> &&
760 std::common_reference_with<std::iter_value_t<begin_iterator_type>,
value_type>
765 auto v = std::ranges::subrange<begin_iterator_type, end_iterator_type>{begin_it, end_it}
766 | views::convert<value_type>
768 data.insert(data.begin() + pos_as_num, std::ranges::begin(v), std::ranges::end(v));
770 return begin() + pos_as_num;
817 if (begin_it >= end_it)
823 data.erase(data.cbegin() + begin_it_pos,
824 data.cbegin() + end_it_pos);
826 return begin() + begin_it_pos;
850 return erase(pos, pos + 1);
870 data.push_back(
to_rank(value));
990 return data == rhs.data;
996 return data != rhs.data;
1002 return data < rhs.data;
1008 return data > rhs.data;
1014 return data <= rhs.data;
1020 return data >= rhs.data;
1031 template <cereal_archive archive_t>
1032 void CEREAL_SERIALIZE_FUNCTION_NAME(archive_t & archive)
const_iterator begin() const noexcept
Returns an iterator to the first element of the container.
Definition: bitcompressed_vector.hpp:366
reference front() noexcept
Return the first element. Calling front on an empty container is undefined.
Definition: bitcompressed_vector.hpp:484
iterator insert(const_iterator pos, size_type const count, value_type const value)
Inserts count copies of value before position in the container.
Definition: bitcompressed_vector.hpp:723
reference operator[](size_type const i) noexcept
Return the i-th element.
Definition: bitcompressed_vector.hpp:458
const_iterator cend() const noexcept
Returns an iterator to the element following the last element of the container.
Definition: bitcompressed_vector.hpp:402
constexpr bool operator!=(bitcompressed_vector const &rhs) const noexcept
Checks whether *this is not equal to rhs.
Definition: bitcompressed_vector.hpp:994
constexpr auto assign_rank_to
Assign a rank to an alphabet object.
Definition: concept.hpp:239
constexpr friend void swap(bitcompressed_vector &lhs, bitcompressed_vector &rhs) noexcept
Swap contents with another instance.
Definition: bitcompressed_vector.hpp:971
constexpr void swap(bitcompressed_vector &&rhs) noexcept
Swap contents with another instance.
Definition: bitcompressed_vector.hpp:954
const_reference operator[](size_type const i) const noexcept
Return the i-th element.
Definition: bitcompressed_vector.hpp:465
constexpr void swap(bitcompressed_vector &rhs) noexcept
Swap contents with another instance.
Definition: bitcompressed_vector.hpp:948
void assign(other_range_t &&range)
Assign from a different range.
Definition: bitcompressed_vector.hpp:273
constexpr bool operator>(bitcompressed_vector const &rhs) const noexcept
Checks whether *this is greater than rhs.
Definition: bitcompressed_vector.hpp:1006
void pop_back()
Removes the last element of the container.
Definition: bitcompressed_vector.hpp:889
reference back() noexcept
Return the last element.
Definition: bitcompressed_vector.hpp:510
Provides C++20 additions to the <iterator> header.
Provides seqan3::views::convert.
iterator begin() noexcept
Returns an iterator to the first element of the container.
Definition: bitcompressed_vector.hpp:360
bitcompressed_vector & operator=(std::initializer_list< value_type > ilist)
Assign from std::initializer_list.
Definition: bitcompressed_vector.hpp:253
constexpr auto to_rank
Return the rank representation of a (semi-)alphabet object.
Definition: concept.hpp:143
iterator insert(const_iterator pos, value_type const value)
Inserts value before position in the container.
Definition: bitcompressed_vector.hpp:699
bitcompressed_vector(std::initializer_list< value_type > ilist)
Construct from std::initializer_list.
Definition: bitcompressed_vector.hpp:238
iterator erase(const_iterator begin_it, const_iterator end_it)
Removes specified elements from the container.
Definition: bitcompressed_vector.hpp:815
const_iterator end() const noexcept
Returns an iterator to the element following the last element of the container.
Definition: bitcompressed_vector.hpp:396
constexpr bool operator<=(bitcompressed_vector const &rhs) const noexcept
Checks whether *this is less than or equal to rhs.
Definition: bitcompressed_vector.hpp:1012
alphabet_type const_reference
Equals the alphabet_type / value_type.
Definition: bitcompressed_vector.hpp:139
iterator insert(const_iterator pos, std::initializer_list< value_type > const &ilist)
Inserts elements from initializer list before position in the container.
Definition: bitcompressed_vector.hpp:791
constexpr bitcompressed_vector & operator=(bitcompressed_vector &&)=default
Defaulted.
void push_back(value_type const value)
Appends the given element value to the end of the container.
Definition: bitcompressed_vector.hpp:868
Provides the seqan3::detail::random_access_iterator class.
iterator end() noexcept
Returns an iterator to the element following the last element of the container.
Definition: bitcompressed_vector.hpp:390
constexpr data_type & raw_data() noexcept
Provides direct, unsafe access to underlying data structures.
Definition: bitcompressed_vector.hpp:532
reference_proxy_type reference
A proxy type that enables assignment, if the underlying data structure also provides a proxy.
Definition: bitcompressed_vector.hpp:137
bitcompressed_vector()=default
Defaulted.
Provides seqan3::alphabet_proxy.
constexpr friend void swap(bitcompressed_vector &&lhs, bitcompressed_vector &&rhs) noexcept
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: bitcompressed_vector.hpp:977
void assign(begin_iterator_type begin_it, end_iterator_type end_it)
Assign from pair of iterators.
Definition: bitcompressed_vector.hpp:316
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
~bitcompressed_vector()=default
Defaulted.
void assign(std::initializer_list< value_type > ilist)
Assign from std::initializer_list.
Definition: bitcompressed_vector.hpp:337
void shrink_to_fit()
Requests the removal of unused capacity.
Definition: bitcompressed_vector.hpp:656
void resize(size_type const count)
Resizes the container to contain count elements.
Definition: bitcompressed_vector.hpp:921
bitcompressed_vector(other_range_t &&range)
Construct from a different range.
Definition: bitcompressed_vector.hpp:182
constexpr bitcompressed_vector & operator=(bitcompressed_vector const &)=default
Defaulted.
bitcompressed_vector(begin_iterator_type begin_it, end_iterator_type end_it)
Construct from pair of iterators.
Definition: bitcompressed_vector.hpp:218
constexpr bool operator>=(bitcompressed_vector const &rhs) const noexcept
Checks whether *this is greater than or equal to rhs.
Definition: bitcompressed_vector.hpp:1018
bitcompressed_vector(size_type const count, value_type const value)
Construct with count times value.
Definition: bitcompressed_vector.hpp:198
iterator insert(const_iterator pos, begin_iterator_type begin_it, end_iterator_type end_it)
Inserts elements from range [begin_it, end_it) before position in the container.
Definition: bitcompressed_vector.hpp:757
const_reference at(size_type const i) const
Return the i-th element.
Definition: bitcompressed_vector.hpp:434
Provides seqan3::views::to_char.
Adaptations of concepts from the Ranges TS.
constexpr bool operator<(bitcompressed_vector const &rhs) const noexcept
Checks whether *this is less than rhs.
Definition: bitcompressed_vector.hpp:1000
size_type capacity() const noexcept
Returns the number of elements that the container has currently allocated space for.
Definition: bitcompressed_vector.hpp:613
const_reference back() const noexcept
Return the last element.
Definition: bitcompressed_vector.hpp:517
void reserve(size_type const new_cap)
Increase the capacity to a value that's greater or equal to new_cap.
Definition: bitcompressed_vector.hpp:636
void assign(size_type const count, value_type const value)
Assign with count times value.
Definition: bitcompressed_vector.hpp:294
bool empty() const noexcept
Checks whether the container is empty.
Definition: bitcompressed_vector.hpp:558
SeqAn specific customisations in the standard namespace.
constexpr bool operator==(bitcompressed_vector const &rhs) const noexcept
Checks whether *this is equal to rhs.
Definition: bitcompressed_vector.hpp:988
constexpr auto to_rank() const noexcept
Returns the rank.
Definition: alphabet_proxy.hpp:219
Adaptions of concepts from the Cereal library.
detail::random_access_iterator< bitcompressed_vector > iterator
The iterator type of this container (a random access iterator).
Definition: bitcompressed_vector.hpp:141
detail::random_access_iterator< bitcompressed_vector const > const_iterator
The const_iterator type of this container (a random access iterator).
Definition: bitcompressed_vector.hpp:143
A space-optimised version of std::vector that compresses multiple letters into a single byte.
Definition: bitcompressed_vector.hpp:66
size_type size() const noexcept
Returns the number of elements in the container, i.e. std::distance(begin(), end()).
Definition: bitcompressed_vector.hpp:574
std::ranges::range_difference_t< data_type > difference_type
A signed integer type (usually std::ptrdiff_t)
Definition: bitcompressed_vector.hpp:145
void resize(size_type const count, value_type const value)
Resizes the container to contain count elements.
Definition: bitcompressed_vector.hpp:931
Provides math related functionality.
constexpr data_type const & raw_data() const noexcept
Provides direct, unsafe access to underlying data structures.
Definition: bitcompressed_vector.hpp:538
size_type max_size() const noexcept
Returns the maximum number of elements the container is able to hold due to system or library impleme...
Definition: bitcompressed_vector.hpp:593
void clear() noexcept
Removes all elements from the container.
Definition: bitcompressed_vector.hpp:676
Refines seqan3::alphabet and adds assignability.
constexpr bitcompressed_vector(bitcompressed_vector const &)=default
Defaulted.
reference at(size_type const i)
Return the i-th element.
Definition: bitcompressed_vector.hpp:424
std::ranges::range_size_t< data_type > size_type
An unsigned integer type (usually std::size_t)
Definition: bitcompressed_vector.hpp:147
constexpr bitcompressed_vector(bitcompressed_vector &&)=default
Defaulted.
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134
alphabet_type value_type
Equals the alphabet_type.
Definition: bitcompressed_vector.hpp:135
A CRTP-base that eases the definition of proxy types returned in place of regular alphabets.
Definition: alphabet_proxy.hpp:65
const_iterator cbegin() const noexcept
Returns an iterator to the first element of the container.
Definition: bitcompressed_vector.hpp:372
iterator erase(const_iterator pos)
Removes specified elements from the container.
Definition: bitcompressed_vector.hpp:848
auto const to_rank
A view that calls seqan3::to_rank() on each element in the input range.
Definition: to_rank.hpp:67
const_reference front() const noexcept
Return the first element. Calling front on an empty container is undefined.
Definition: bitcompressed_vector.hpp:491
Provides seqan3::views::to_rank.