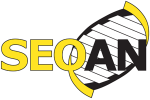 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
15 #include <type_traits>
28 #if SEQAN3_WITH_CEREAL
29 #include <cereal/types/vector.hpp>
32 namespace seqan3::detail
47 template <
typename value_type,
bool const_>
48 struct concatenated_sequences_reference_proxy :
50 decltype(std::declval<value_type const &>() | views::as_const | views::slice(0,1)),
51 decltype(std::declval<value_type &>() | views::slice(0,1))>
57 decltype(std::declval<value_type &>() |
views::slice(0,1))>;
63 concatenated_sequences_reference_proxy(base_t && rhs) : base_t{
std::move(rhs)} {}
66 operator value_type()
const
70 std::ranges::copy(*
this, std::ranges::begin(ret));
125 template <
typename inner_type,
130 std::is_same_v<std::ranges::range_size_t<inner_type>, std::ranges::range_value_t<data_delimiters_type>>
139 data_delimiters_type data_delimiters{0};
152 using reference = detail::concatenated_sequences_reference_proxy<value_type, false>;
156 using const_reference = detail::concatenated_sequences_reference_proxy<value_type, true>;
160 using iterator = detail::random_access_iterator<concatenated_sequences>;
164 using const_iterator = detail::random_access_iterator<concatenated_sequences const>;
172 using size_type = std::ranges::range_size_t<data_delimiters_type>;
177 using allocator_type = void;
187 template <std::ranges::range t>
190 return range_dimension_v<t> == range_dimension_v<value_type> &&
191 std::convertible_to<std::ranges::range_reference_t<t>, std::ranges::range_value_t<value_type>>;
194 static constexpr
bool is_compatible_with_value_type_aux(...)
203 template <std::ranges::range t>
209 template <
typename t>
211 requires is_compatible_with_value_type<std::iter_reference_t<t>>
218 template <std::ranges::range t>
220 requires is_compatible_with_value_type<std::ranges::range_reference_t<t>>
255 template <std::ranges::input_range rng_of_rng_type>
258 requires range_value_t_is_compatible_with_value_type<rng_of_rng_type>
261 if constexpr (std::ranges::sized_range<rng_of_rng_type>)
264 for (
auto && val : rng_of_rng)
266 data_values.insert(data_values.end(), val.begin(), val.end());
267 data_delimiters.push_back(data_delimiters.back() + val.size());
284 template <std::ranges::forward_range rng_type>
287 requires is_compatible_with_value_type<rng_type>
312 template <std::forward_iterator begin_iterator_type,
typename end_iterator_type>
315 requires std::sized_sentinel_for<end_iterator_type, begin_iterator_type> &&
316 iter_value_t_is_compatible_with_value_type<begin_iterator_type>
334 template <std::ranges::forward_range value_type_t = value_type>
336 requires is_compatible_with_value_type<value_type_t>
355 template <std::ranges::forward_range value_type_t>
358 requires is_compatible_with_value_type<value_type_t>
378 template <std::ranges::input_range rng_of_rng_type>
379 void assign(rng_of_rng_type && rng_of_rng)
381 requires range_value_t_is_compatible_with_value_type<rng_of_rng_type>
401 template <std::ranges::forward_range rng_type>
404 requires (is_compatible_with_value_type<rng_type>)
426 template <std::forward_iterator begin_iterator_type,
typename end_iterator_type>
427 void assign(begin_iterator_type begin_it, end_iterator_type end_it)
429 requires iter_value_t_is_compatible_with_value_type<begin_iterator_type> &&
430 std::sized_sentinel_for<end_iterator_type, begin_iterator_type>
449 template <std::ranges::forward_range rng_type = value_type>
452 requires is_compatible_with_value_type<rng_type>
544 throw std::out_of_range{
"Trying to access element behind the last in concatenated_sequences."};
553 throw std::out_of_range{
"Trying to access element behind the last in concatenated_sequences."};
574 return data_values |
views::slice(data_delimiters[i], data_delimiters[i+1]);
624 return (*
this)[
size()-1];
631 return (*
this)[
size()-1];
670 return {data_values, data_delimiters};
726 return data_delimiters.size() - 1;
745 return data_delimiters.max_size() - 1;
765 return data_delimiters.capacity();
792 data_delimiters.reserve(new_cap + 1);
816 data_values.shrink_to_fit();
817 data_delimiters.shrink_to_fit();
837 return data_values.size();
853 return data_values.capacity();
876 data_values.reserve(new_cap);
898 data_delimiters.clear();
899 data_delimiters.push_back(0);
926 template <std::ranges::forward_range rng_type>
929 requires is_compatible_with_value_type<rng_type>
932 return insert(pos, 1, std::forward<rng_type>(value));
960 template <std::ranges::forward_range rng_type>
963 requires is_compatible_with_value_type<rng_type>
969 return begin() + pos_as_num;
981 if constexpr (std::ranges::sized_range<rng_type>)
984 value_len =
std::distance(std::ranges::begin(value), std::ranges::end(value));
986 data_values.reserve(data_values.size() +
count * value_len);
988 | std::views::common;
990 data_values.insert(data_values.begin() + data_delimiters[pos_as_num],
991 std::ranges::begin(placeholder),
992 std::ranges::end(placeholder));
995 size_t i = data_delimiters[pos_as_num];
996 for (
size_t j = 0; j <
count; ++j)
997 for (
auto && v : value)
998 data_values[i++] = v;
1000 data_delimiters.reserve(data_values.size() +
count);
1001 data_delimiters.insert(data_delimiters.begin() + pos_as_num,
1003 *(data_delimiters.begin() + pos_as_num));
1007 data_delimiters[pos_as_num + i + 1] += value_len * (i + 1);
1012 data_delimiters.end(),
1013 [full_len = value_len *
count] (
auto & d) { d += full_len; });
1015 return begin() + pos_as_num;
1042 template <std::forward_iterator begin_iterator_type,
typename end_iterator_type>
1045 requires iter_value_t_is_compatible_with_value_type<begin_iterator_type> &&
1046 std::sized_sentinel_for<end_iterator_type, begin_iterator_type>
1051 if (last - first == 0)
1052 return begin() + pos_as_num;
1055 std::ranges::subrange<begin_iterator_type, end_iterator_type>(first,
1057 std::ranges::distance(first, last));
1059 data_delimiters.reserve(data_values.size() + ilist.size());
1060 data_delimiters.insert(data_delimiters.begin() + pos_as_num,
1062 *(data_delimiters.begin() + pos_as_num));
1067 for (
size_type i = 0; i < ilist.size(); ++i, ++first)
1069 full_len += std::ranges::distance(*first);
1070 data_delimiters[pos_as_num + 1 + i] += full_len;
1074 auto placeholder =
views::repeat_n(std::ranges::range_value_t<value_type>{}, full_len)
1075 | std::views::common;
1077 data_values.insert(data_values.begin() + data_delimiters[pos_as_num],
1078 std::ranges::begin(placeholder),
1079 std::ranges::end(placeholder));
1082 size_t i = data_delimiters[pos_as_num];
1083 for (
auto && v0 : ilist)
1084 for (
auto && v1 : v0)
1085 data_values[i++] = v1;
1090 std::for_each(data_delimiters.begin() + pos_as_num + ilist.size() + 1,
1091 data_delimiters.end(),
1092 [full_len] (
auto & d) { d += full_len; });
1094 return begin() + pos_as_num;
1116 template <std::ranges::forward_range rng_type>
1119 requires is_compatible_with_value_type<rng_type>
1122 return insert(pos, ilist.begin(), ilist.end());
1147 if (last - first == 0)
1148 return begin() + dist;
1154 for (; first != last; ++first)
1157 data_values.erase(data_values.begin() + data_delimiters[distf],
1158 data_values.begin() + data_delimiters[dist]);
1160 data_delimiters.erase(data_delimiters.begin() + distf + 1,
1161 data_delimiters.begin() + dist + 1);
1166 data_delimiters.end(),
1167 [sum_size] (
auto & d) { d -= sum_size; });
1168 return begin() + dist;
1191 return erase(pos, pos + 1);
1210 template <std::ranges::forward_range rng_type>
1213 requires is_compatible_with_value_type<rng_type>
1216 data_values.insert(data_values.end(), std::ranges::begin(value), std::ranges::end(value));
1239 auto back_length = data_delimiters[
size()] - data_delimiters[
size() - 1];
1240 data_values.resize(data_values.size() - back_length);
1241 data_delimiters.pop_back();
1273 data_delimiters.resize(
count + 1, data_delimiters.back());
1274 data_values.resize(data_delimiters.back());
1282 template <std::ranges::forward_range rng_type>
1285 requires is_compatible_with_value_type<rng_type>
1310 std::swap(data_values, rhs.data_values);
1311 std::swap(data_delimiters, rhs.data_delimiters);
1317 std::swap(data_values, rhs.data_values);
1318 std::swap(data_delimiters, rhs.data_delimiters);
1329 return raw_data() == rhs.raw_data();
1335 return raw_data() != rhs.raw_data();
1341 return raw_data() < rhs.raw_data();
1347 return raw_data() > rhs.raw_data();
1353 return raw_data() <= rhs.raw_data();
1359 return raw_data() >= rhs.raw_data();
1370 template <cereal_archive archive_t>
1371 void CEREAL_SERIALIZE_FUNCTION_NAME(archive_t & archive)
1373 archive(data_values, data_delimiters);
A more refined container concept than seqan3::random_access_container.
Provides seqan3::views::as_const.
size_type concat_size() const noexcept
Returns the cumulative size of all elements in the container.
Definition: concatenated_sequences.hpp:835
detail::concatenated_sequences_reference_proxy< value_type, false > reference
A proxy of type views::slice that represents the range on the concatenated vector.
Definition: concatenated_sequences.hpp:152
iterator insert(const_iterator pos, rng_type &&value)
Inserts value before position in the container.
Definition: concatenated_sequences.hpp:927
reference at(size_type const i)
Return the i-th element as a view.
Definition: concatenated_sequences.hpp:540
constexpr concatenated_sequences & operator=(concatenated_sequences const &)=default
Default constructors.
reference back()
Return the last element as a view.
Definition: concatenated_sequences.hpp:621
concatenated_sequences & operator=(std::initializer_list< value_type_t > ilist)
Construct/assign from std::initializer_list.
Definition: concatenated_sequences.hpp:356
bool empty() const noexcept
Checks whether the container is empty.
Definition: concatenated_sequences.hpp:708
concatenated_sequences(rng_of_rng_type &&rng_of_rng)
Construct/assign from a different range.
Definition: concatenated_sequences.hpp:256
void shrink_to_fit()
Requests the removal of unused capacity.
Definition: concatenated_sequences.hpp:814
constexpr concatenated_sequences(concatenated_sequences &&)=default
Default constructors.
constexpr bool operator==(concatenated_sequences const &rhs) const noexcept
Checks whether *this is equal to rhs.
Definition: concatenated_sequences.hpp:1327
size_type capacity() const noexcept
Returns the number of elements that the container has currently allocated space for.
Definition: concatenated_sequences.hpp:763
const_iterator cbegin() const noexcept
Returns an iterator to the first element of the container.
Definition: concatenated_sequences.hpp:488
detail::random_access_iterator< concatenated_sequences const > const_iterator
The const iterator type of this container (a random access iterator).
Definition: concatenated_sequences.hpp:164
const_iterator end() const noexcept
Returns an iterator to the element following the last element of the container.
Definition: concatenated_sequences.hpp:512
iterator end() noexcept
Returns an iterator to the element following the last element of the container.
Definition: concatenated_sequences.hpp:506
iterator erase(const_iterator pos)
Removes specified elements from the container.
Definition: concatenated_sequences.hpp:1189
constexpr void swap(concatenated_sequences &rhs) noexcept
Swap contents with another instance.
Definition: concatenated_sequences.hpp:1308
constexpr void swap(concatenated_sequences &&rhs) noexcept
Swap contents with another instance.
Definition: concatenated_sequences.hpp:1315
detail::random_access_iterator< concatenated_sequences > iterator
The iterator type of this container (a random access iterator).
Definition: concatenated_sequences.hpp:160
Provides C++20 additions to the <iterator> header.
void clear() noexcept
Removes all elements from the container.
Definition: concatenated_sequences.hpp:895
concatenated_sequences(std::initializer_list< value_type_t > ilist)
Construct/assign from std::initializer_list.
Definition: concatenated_sequences.hpp:338
const_reference concat() const
Return the concatenation of all members.
Definition: concatenated_sequences.hpp:654
Adaptations of concepts from the standard library.
void push_back(rng_type &&value)
Appends the given element value to the end of the container.
Definition: concatenated_sequences.hpp:1211
constexpr bool operator>=(concatenated_sequences const &rhs) const noexcept
Checks whether *this is greater than or equal to rhs.
Definition: concatenated_sequences.hpp:1357
detail::concatenated_sequences_reference_proxy< value_type, true > const_reference
An immutable proxy of type views::slice that represents the range on the concatenated vector.
Definition: concatenated_sequences.hpp:156
void reserve(size_type const new_cap)
Increase the capacity to a value that's greater or equal to new_cap.
Definition: concatenated_sequences.hpp:790
void pop_back()
Removes the last element of the container.
Definition: concatenated_sequences.hpp:1236
concatenated_sequences()=default
Default constructors.
void resize(size_type const count)
Resizes the container to contain count elements.
Definition: concatenated_sequences.hpp:1270
constexpr bool operator<=(concatenated_sequences const &rhs) const noexcept
Checks whether *this is less than or equal to rhs.
Definition: concatenated_sequences.hpp:1351
iterator erase(const_iterator first, const_iterator last)
Removes specified elements from the container.
Definition: concatenated_sequences.hpp:1143
void resize(size_type const count, rng_type &&value)
Resizes the container to contain count elements.
Definition: concatenated_sequences.hpp:1283
Provides the seqan3::detail::random_access_iterator class.
void assign(size_type const count, rng_type &&value)
Construct/assign with count times value.
Definition: concatenated_sequences.hpp:402
void concat_reserve(size_type const new_cap)
Increase the concat_capacity() to a value that's greater or equal to new_cap.
Definition: concatenated_sequences.hpp:874
const_iterator begin() const noexcept
Returns an iterator to the first element of the container.
Definition: concatenated_sequences.hpp:482
Provides seqan3::views::slice.
auto const as_const
A view that provides only const & to elements of the underlying range.
Definition: as_const.hpp:87
Provides seqan3::views::repeat_n.
Container that stores sequences concatenated internally.
Definition: concatenated_sequences.hpp:133
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
const_iterator cend() const noexcept
Returns an iterator to the element following the last element of the container.
Definition: concatenated_sequences.hpp:518
constexpr concatenated_sequences & operator=(concatenated_sequences &&)=default
Default constructors.
iterator begin() noexcept
Returns an iterator to the first element of the container.
Definition: concatenated_sequences.hpp:476
reference concat()
Return the concatenation of all members.
Definition: concatenated_sequences.hpp:648
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
reference operator[](size_type const i)
Return the i-th element as a view.
Definition: concatenated_sequences.hpp:571
const_reference at(size_type const i) const
Return the i-th element as a view.
Definition: concatenated_sequences.hpp:549
size_type max_size() const noexcept
Returns the maximum number of elements the container is able to hold due to system or library impleme...
Definition: concatenated_sequences.hpp:743
const_reference back() const
Return the last element as a view.
Definition: concatenated_sequences.hpp:628
concatenated_sequences(size_type const count, rng_type &&value)
Construct/assign with count times value.
Definition: concatenated_sequences.hpp:285
constexpr concatenated_sequences(concatenated_sequences const &)=default
Default constructors.
std::pair< decltype(data_values) const &, decltype(data_delimiters) const & > data() const
Provides direct, unsafe access to underlying data structures.
Definition: concatenated_sequences.hpp:688
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
Provides seqan3::views::join.
Adaptations of concepts from the Ranges TS.
size_type concat_capacity() const noexcept
Returns the concatenated size the container has currently allocated space for.
Definition: concatenated_sequences.hpp:851
const_reference front() const
Return the first element as a view. Calling front on an empty container is undefined.
Definition: concatenated_sequences.hpp:603
The identity transformation (a transformation_trait that returns the input).
const_reference operator[](size_type const i) const
Return the i-th element as a view.
Definition: concatenated_sequences.hpp:578
size_type size() const noexcept
Returns the number of elements in the container, i.e. std::distance(begin(), end()).
Definition: concatenated_sequences.hpp:724
std::ranges::range_difference_t< data_delimiters_type > difference_type
A signed integer type (usually std::ptrdiff_t)
Definition: concatenated_sequences.hpp:168
std::ranges::range_size_t< data_delimiters_type > size_type
An unsigned integer type (usually std::size_t)
Definition: concatenated_sequences.hpp:172
~concatenated_sequences()=default
Default constructors.
constexpr auto slice
A view adaptor that returns a half-open interval on the underlying range.
Definition: slice.hpp:141
void assign(begin_iterator_type begin_it, end_iterator_type end_it)
Construct/assign from pair of iterators.
Definition: concatenated_sequences.hpp:427
reference front()
Return the first element as a view. Calling front on an empty container is undefined.
Definition: concatenated_sequences.hpp:596
constexpr bool operator<(concatenated_sequences const &rhs) const noexcept
Checks whether *this is less than rhs.
Definition: concatenated_sequences.hpp:1339
concatenated_sequences(begin_iterator_type begin_it, end_iterator_type end_it)
Construct/assign from pair of iterators.
Definition: concatenated_sequences.hpp:313
Adaptions of concepts from the Cereal library.
constexpr auto repeat_n
A view factory that repeats a given value n times.
Definition: repeat_n.hpp:94
std::pair< decltype(data_values) &, decltype(data_delimiters) & > raw_data()
Provides direct, unsafe access to underlying data structures.
Definition: concatenated_sequences.hpp:668
void assign(std::initializer_list< rng_type > ilist)
Construct/assign from std::initializer_list.
Definition: concatenated_sequences.hpp:450
iterator insert(const_iterator pos, std::initializer_list< rng_type > const &ilist)
Inserts elements from initializer list before position in the container.
Definition: concatenated_sequences.hpp:1117
void assign(rng_of_rng_type &&rng_of_rng)
Construct/assign from a different range.
Definition: concatenated_sequences.hpp:379
iterator insert(const_iterator pos, size_type const count, rng_type &&value)
Inserts count copies of value before position in the container.
Definition: concatenated_sequences.hpp:961
static constexpr bool is_compatible_with_value_type
Whether a type satisfies seqan3::range_compatible with this class's value_type or reference type.
Definition: concatenated_sequences.hpp:204
iterator insert(const_iterator pos, begin_iterator_type first, end_iterator_type last)
Inserts elements from range [first, last) before position in the container.
Definition: concatenated_sequences.hpp:1043
constexpr bool operator>(concatenated_sequences const &rhs) const noexcept
Checks whether *this is greater than rhs.
Definition: concatenated_sequences.hpp:1345
std::pair< decltype(data_values) &, decltype(data_delimiters) & > data()
Provides direct, unsafe access to underlying data structures.
Definition: concatenated_sequences.hpp:681
static constexpr bool iter_value_t_is_compatible_with_value_type
Whether a type satisfies seqan3::range_compatible with this class.
Definition: concatenated_sequences.hpp:213
std::pair< decltype(data_values) const &, decltype(data_delimiters) const & > raw_data() const
Provides direct, unsafe access to underlying data structures.
Definition: concatenated_sequences.hpp:674
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134
constexpr bool operator!=(concatenated_sequences const &rhs) const noexcept
Checks whether *this is not equal to rhs.
Definition: concatenated_sequences.hpp:1333
static constexpr bool range_value_t_is_compatible_with_value_type
Whether a type satisfies seqan3::range_compatible with this class.
Definition: concatenated_sequences.hpp:222