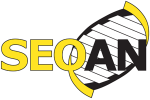 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
17 #include <meta/meta.hpp>
32 template <detail::config_element_specialisation ... configs_t>
35 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
36 constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t> && lhs,
37 pipeable_config_element<rhs_derived_t, rhs_value_t> && rhs)
39 return configuration{
static_cast<lhs_derived_t &&
>(lhs)}.push_back(
static_cast<rhs_derived_t &&
>(rhs));
42 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
43 constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t> && lhs,
44 pipeable_config_element<rhs_derived_t, rhs_value_t>
const & rhs)
46 return configuration{
static_cast<lhs_derived_t &&
>(lhs)}.push_back(
static_cast<rhs_derived_t
const &
>(rhs));
49 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
50 constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t>
const & lhs,
51 pipeable_config_element<rhs_derived_t, rhs_value_t> && rhs)
53 return configuration{
static_cast<lhs_derived_t
const &
>(lhs)}.push_back(
static_cast<rhs_derived_t &&
>(rhs));
56 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
57 constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t>
const & lhs,
58 pipeable_config_element<rhs_derived_t, rhs_value_t>
const & rhs)
60 return configuration{
static_cast<lhs_derived_t
const &
>(lhs)}.push_back(
static_cast<rhs_derived_t
const &
>(rhs));
80 template <detail::config_element_specialisation ... configs_t>
84 template <detail::config_element_specialisation ... _configs_t>
106 template <
typename derived_t,
typename value_t>
107 constexpr
configuration(pipeable_config_element<derived_t, value_t> && elem) :
114 template <
typename derived_t,
typename value_t>
115 constexpr
configuration(pipeable_config_element<derived_t, value_t>
const & elem) :
116 base_type{
static_cast<derived_t
const &
>(elem)}
124 constexpr
size_t size() const noexcept
127 return std::tuple_size_v<base_type>;
160 template <
typename alternative_t>
161 constexpr decltype(
auto)
get_or(alternative_t && alternative) & noexcept
163 return get_or_impl(*
this, alternative, std::forward<alternative_t>(alternative));
167 template <
typename alternative_t>
168 constexpr decltype(
auto)
get_or(alternative_t && alternative) const & noexcept
170 return get_or_impl(*
this, alternative, std::forward<alternative_t>(alternative));
174 template <
typename alternative_t>
175 constexpr decltype(
auto)
get_or(alternative_t && alternative) && noexcept
177 return get_or_impl(
std::move(*
this), alternative, std::forward<alternative_t>(alternative));
181 template <
typename alternative_t>
182 constexpr decltype(
auto)
get_or(alternative_t && alternative) const && noexcept
184 return get_or_impl(
std::move(*
this), alternative, std::forward<alternative_t>(alternative));
188 template <
typename query_t>
194 template <
template <
typename ...>
typename query_t>
197 return (
pack_traits::find_if<detail::is_same_configuration_f<query_t>::template invoke, configs_t...> > -1);
208 template <
typename query_t>
209 [[nodiscard]] constexpr
auto remove() const
211 #if !SEQAN3_WORKAROUND_GCC_95371
212 requires (exists<query_t>())
213 #endif // !SEQAN3_WORKAROUND_GCC_95371
217 return remove_at<index>();
221 template <
template <
typename ...>
typename query_t>
222 [[nodiscard]] constexpr
auto remove() const
224 #if !SEQAN3_WORKAROUND_GCC_95371
225 requires (exists<query_t>())
226 #endif // !SEQAN3_WORKAROUND_GCC_95371
229 constexpr
int index = pack_traits::find_if<detail::is_same_configuration_f<query_t>::template invoke,
231 return remove_at<index>();
247 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
248 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t> && lhs,
249 pipeable_config_element<rhs_derived_t, rhs_value_t> && rhs);
252 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
253 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t> && lhs,
254 pipeable_config_element<rhs_derived_t, rhs_value_t>
const & rhs);
257 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
258 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t>
const & lhs,
259 pipeable_config_element<rhs_derived_t, rhs_value_t> && rhs);
262 template <
typename lhs_derived_t,
typename lhs_value_t,
typename rhs_derived_t,
typename rhs_value_t>
263 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t>
const & lhs,
264 pipeable_config_element<rhs_derived_t, rhs_value_t>
const & rhs);
273 template <
typename rhs_derived_t,
typename rhs_value_t>
275 pipeable_config_element<rhs_derived_t, rhs_value_t> && rhs)
277 return std::move(lhs).push_back(
static_cast<rhs_derived_t &&
>(rhs));
281 template <
typename rhs_derived_t,
typename rhs_value_t>
283 pipeable_config_element<rhs_derived_t, rhs_value_t> && rhs)
285 return lhs.push_back(
static_cast<rhs_derived_t &&
>(rhs));
289 template <
typename rhs_derived_t,
typename rhs_value_t>
291 pipeable_config_element<rhs_derived_t, rhs_value_t>
const & rhs)
293 return std::move(lhs).push_back(
static_cast<rhs_derived_t
const &
>(rhs));
297 template <
typename rhs_derived_t,
typename rhs_value_t>
299 pipeable_config_element<rhs_derived_t, rhs_value_t>
const & rhs)
301 return lhs.push_back(
static_cast<rhs_derived_t
const &
>(rhs));
311 template <
typename lhs_derived_t,
typename lhs_value_t>
312 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t> && lhs,
319 template <
typename lhs_derived_t,
typename lhs_value_t>
320 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t> && lhs,
327 template <
typename lhs_derived_t,
typename lhs_value_t>
328 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t>
const & lhs,
335 template <
typename lhs_derived_t,
typename lhs_value_t>
336 friend constexpr
auto operator|(pipeable_config_element<lhs_derived_t, lhs_value_t>
const & lhs,
348 template <
typename ...rhs_configs_t>
356 static_cast<rhs_base_t
>(
std::move(rhs))));
360 template <
typename ...rhs_configs_t>
367 return make_configuration(
std::tuple_cat(
static_cast<lhs_base_t
>(lhs),
368 static_cast<rhs_base_t
>(
std::move(rhs))));
372 template <
typename ...rhs_configs_t>
380 static_cast<rhs_base_t
>(rhs)));
384 template <
typename ...rhs_configs_t>
391 return make_configuration(
std::tuple_cat(
static_cast<lhs_base_t
>(lhs),
392 static_cast<rhs_base_t
>(rhs)));
401 template <
typename ..._configs_t>
407 template <
typename ..._configs_t>
417 template <tuple_like tuple_t>
418 static constexpr
auto make_configuration(tuple_t && tpl)
422 return configuration<>{};
426 auto impl = [](
auto & impl_ref,
auto && config,
auto && head,
auto && tail)
428 using cfg_t = decltype(config);
431 return std::forward<cfg_t>(config).push_back(std::get<0>(
std::forward<decltype(head)>(head)));
435 auto [_head, _tail] = tuple_split<1>(
std::forward<decltype(tail)>(tail));
436 auto tmp = std::forward<cfg_t>(config).push_back(std::get<0>(
std::forward<decltype(head)>(head)));
441 auto [head, tail] = tuple_split<1>(
std::forward<decltype(tpl)>(tpl));
472 template <detail::config_element_specialisation config_element_t>
473 constexpr
auto push_back(config_element_t elem)
const &
477 "Configuration error: The passed element cannot be combined with one or more elements in the "
478 "current configuration.");
486 template <detail::config_element_specialisation config_element_t>
487 constexpr
auto push_back(config_element_t elem) &&
491 "Configuration error: The passed element cannot be combined with one or more elements in the "
492 "current configuration.");
504 [[nodiscard]] constexpr
auto remove_at()
const
506 static_assert((index >= 0) && (index <
sizeof...(configs_t)),
"Index to remove from config is out of bounds.");
508 auto [head, middle] = tuple_split<index>(
static_cast<base_type
>(*
this));
532 template <
typename this_t,
typename query_t,
typename alternative_t>
533 static constexpr decltype(
auto) get_or_impl(this_t && me,
534 query_t const & SEQAN3_DOXYGEN_ONLY(query),
535 alternative_t && alternative) noexcept
537 if constexpr (exists<query_t>())
539 return get<query_t>(std::forward<this_t>(me));
544 return static_cast<ret_type
>(alternative);
549 template <
typename this_t,
550 template <
typename ...>
typename query_template_t,
typename ...parameters_t,
551 typename alternative_t>
552 static constexpr decltype(
auto) get_or_impl(this_t && me,
553 query_template_t<parameters_t...> const &,
554 alternative_t && alternative) noexcept
556 if constexpr (exists<query_template_t>())
558 return get<query_template_t>(std::forward<this_t>(me));
563 return static_cast<ret_type
>(alternative);
575 template <
typename derived_t,
typename value_t>
581 template <
typename derived_t,
typename value_t>
616 template <
template <
typename ...>
class query_t,
typename ...configs_t>
619 constexpr
auto pos = pack_traits::find_if<detail::is_same_configuration_f<query_t>::template invoke, configs_t...>;
620 static_assert(pos > -1,
"Access error: The requested type is not contained.");
622 return get<pos>(config);
626 template <
template <
typename ...>
class query_t,
typename ...configs_t>
629 constexpr
auto pos = pack_traits::find_if<detail::is_same_configuration_f<query_t>::template invoke, configs_t...>;
630 static_assert(pos > -1,
"Access error: The requested type is not contained.");
632 return get<pos>(config);
636 template <
template <
typename ...>
class query_t,
typename ...configs_t>
639 constexpr
auto pos = pack_traits::find_if<detail::is_same_configuration_f<query_t>::template invoke, configs_t...>;
640 static_assert(pos > -1,
"Access error: The requested type is not contained.");
646 template <
template <
typename ...>
class query_t,
typename ...configs_t>
649 constexpr
auto pos = pack_traits::find_if<detail::is_same_configuration_f<query_t>::template invoke, configs_t...>;
650 static_assert(pos > -1,
"Access error: The requested type is not contained.");
669 template <seqan3::detail::config_element_specialisation ... configs_t>
670 struct tuple_size<
seqan3::configuration<configs_t...>>
681 template <
size_t pos, seqan3::detail::config_element_specialisation ... configs_t>
682 struct tuple_element<pos,
seqan3::configuration<configs_t...>>
constexpr configuration(pipeable_config_element< derived_t, value_t > const &elem)
Constructs a configuration from a single configuration element.
Definition: configuration.hpp:115
constexpr friend auto operator|(configuration const &lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > &&rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:282
constexpr ptrdiff_t find
Get the index of the first occurrence of a type in a pack.
Definition: traits.hpp:152
constexpr auto const & get(configuration< configs_t... > const &config) noexcept
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:627
configuration(pipeable_config_element< derived_t, value_t > const &) -> configuration< std::remove_cvref_t< derived_t >>
Deduces the correct configuration element type from the passed seqan3::pipeable_config_element.
Provides seqan3::pipeable_config_element.
static constexpr bool exists() noexcept
Checks if the given type exists in the tuple.
Definition: configuration.hpp:189
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > &&lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
constexpr friend auto operator|(configuration &&lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:290
constexpr decltype(auto) get_or(alternative_t &&alternative) &noexcept
Returns the stored configuration element if present otherwise the given alternative.
Definition: configuration.hpp:161
constexpr bool contains
Whether a type occurs in a pack or not.
Definition: traits.hpp:193
auto operator|(validator1_type &&vali1, validator2_type &&vali2)
Enables the chaining of validators.
Definition: validators.hpp:1037
Provides concepts for the configuration classes.
Collection of elements to configure an algorithm.
Definition: configuration.hpp:82
Provides utility functions for tuple like interfaces.
friend class configuration
Friend declaration for other instances of the configuration.
Definition: configuration.hpp:85
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
constexpr configuration(configuration const &)=default
Defaulted.
constexpr size_t size() const noexcept
Returns the number of contained config elements.
Definition: configuration.hpp:125
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > const &lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
constexpr friend auto operator|(configuration &&lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > &&rhs)
Combines a seqan3::configuration with a seqan3::pipeable_config_element.
Definition: configuration.hpp:274
typename remove_rvalue_reference< t >::type remove_rvalue_reference_t
Return the input type with && removed, but lvalue references preserved (transformation_trait shortcut...
Definition: basic.hpp:69
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
constexpr ptrdiff_t find_if
Get the index of the first type in a pack that satisfies the given predicate.
Definition: traits.hpp:175
constexpr friend auto operator|(configuration &&lhs, configuration< rhs_configs_t... > const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:373
constexpr friend auto operator|(configuration &&lhs, configuration< rhs_configs_t... > &&rhs)
Combines two seqan3::configuration objects.
Definition: configuration.hpp:349
~configuration()=default
Defaulted.
configuration(pipeable_config_element< derived_t, value_t > &&) -> configuration< std::remove_cvref_t< derived_t >>
Deduces the correct configuration element type from the passed seqan3::pipeable_config_element.
constexpr configuration()=default
Defaulted.
constexpr configuration & operator=(configuration const &)=default
Defaulted.
constexpr friend auto operator|(configuration const &lhs, configuration< rhs_configs_t... > const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:385
constexpr configuration(configuration &&)=default
Defaulted.
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > &&lhs, configuration &&rhs)
Combines a seqan3::pipeable_config_element with a seqan3::configuration.
Definition: configuration.hpp:312
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > const &lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > &&rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > &&lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > &&rhs)
Combines two seqan3::pipeable_config_element objects to a seqan3::configuration.
Provides functionality to access get function by enum values.
SeqAn specific customisations in the standard namespace.
constexpr auto & get(configuration< configs_t... > &config) noexcept
Returns the stored element.
Definition: configuration.hpp:617
constexpr auto remove() const
Remove a config element from the configuration.
Definition: configuration.hpp:209
constexpr configuration & operator=(configuration &&)=default
Defaulted.
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > &&lhs, configuration const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:320
Provides traits for seqan3::type_list.
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > const &lhs, configuration const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:336
constexpr friend auto operator|(configuration const &lhs, pipeable_config_element< rhs_derived_t, rhs_value_t > const &rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:298
constexpr auto tuple_pop_front(tuple_t &&t)
Removes the first element of a tuple.
Definition: tuple_utility.hpp:179
constexpr friend auto operator|(configuration const &lhs, configuration< rhs_configs_t... > &&rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:361
constexpr configuration(pipeable_config_element< derived_t, value_t > &&elem)
Constructs a configuration from a single configuration element.
Definition: configuration.hpp:107
constexpr friend auto operator|(pipeable_config_element< lhs_derived_t, lhs_value_t > const &lhs, configuration &&rhs)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: configuration.hpp:328
Provides seqan3::type_list.