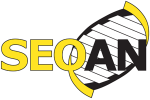 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
20 #include <type_traits>
37 #include <seqan3/io/detail/record.hpp>
137 template <
typename t>
154 requires std::is_floating_point_v<typename t::bpp_prob>;
171 requires std::is_same_v<typename t::structure_alphabet, dssp9>
177 <
typename t::template structured_seq_alphabet
178 <
typename t::seq_alphabet,
typename t::structure_alphabet>,
179 typename t::seq_alphabet,
typename t::structure_alphabet>,
180 typename t::template structured_seq_alphabet<typename t::seq_alphabet, typename t::structure_alphabet>>;
187 requires std::is_floating_point_v<typename t::energy_type::value_type>;
190 requires std::is_floating_point_v<typename t::react_type>;
235 template <
typename _seq_alphabet>
244 template <
typename _
id_alphabet>
256 template <
typename _bpp_prob,
typename _bpp_partner>
260 template <
typename _bpp_item>
264 template <
typename _bpp_queue>
273 template <
typename _structure_alphabet>
279 template <
typename _seq_alphabet,
typename _structure_alphabet>
283 template <
typename _structured_seq_alphabet>
297 template <
typename _react_type>
306 template <
typename _comment_alphabet>
332 template <
typename _seq_alphabet,
typename _structure_alphabet>
487 field::structured_seq,
494 static_assert([]() constexpr
496 for (
field f : selected_field_ids::as_array)
497 if (!field_ids::contains(f))
501 "You selected a field that is not valid for structure files, please refer to the documentation "
502 "of structure_file_input::field_ids for the accepted values.");
504 static_assert([]() constexpr
509 }(),
"You may not select field::structured_seq and either of field::seq and field::structure "
510 "at the same time.");
517 using seq_type =
typename traits_type::template seq_container<typename traits_type::seq_alphabet>;
520 using id_type =
typename traits_type::template id_container<typename traits_type::id_alphabet>;
522 using bpp_type =
typename traits_type::template bpp_container
523 <
typename traits_type::template bpp_queue
524 <
typename traits_type::template bpp_item
525 <
typename traits_type::bpp_prob,
typename traits_type::bpp_partner>>>;
528 <
typename traits_type::structure_alphabet>;
531 <
typename traits_type::template structured_seq_alphabet
532 <
typename traits_type::seq_alphabet,
typename traits_type::structure_alphabet>>;
536 using react_type =
typename traits_type::template react_container<typename traits_type::react_type>;
539 <
typename traits_type::comment_alphabet>;
567 using iterator = detail::in_file_iterator<structure_file_input>;
610 primary_stream->rdbuf()->pubsetbuf(stream_buffer.
data(), stream_buffer.
size());
612 std::ios_base::in | std::ios::binary);
614 if (!primary_stream->good())
615 throw file_open_error{
"Could not open file " + filename.
string() +
" for reading."};
618 secondary_stream = detail::make_secondary_istream(*primary_stream, filename);
621 detail::set_format(format, filename);
639 template <input_stream stream_t, structure_file_input_format file_format>
641 requires std::same_as<typename std::remove_reference_t<stream_t>::char_type,
char>
644 file_format
const & SEQAN3_DOXYGEN_ONLY(format_tag),
646 primary_stream{&stream, stream_deleter_noop},
647 format{detail::structure_file_input_format_exposer<file_format>{}}
649 static_assert(list_traits::contains<file_format, valid_formats>,
650 "You selected a format that is not in the valid_formats of this file.");
653 secondary_stream = detail::make_secondary_istream(*primary_stream);
657 template <input_stream stream_t, structure_file_input_format file_format>
659 requires std::same_as<typename std::remove_reference_t<stream_t>::char_type,
char>
662 file_format
const & SEQAN3_DOXYGEN_ONLY(format_tag),
664 primary_stream{
new stream_t{
std::move(stream)}, stream_deleter_default},
665 format{detail::structure_file_input_format_exposer<file_format>{}}
667 static_assert(list_traits::contains<file_format, valid_formats>,
668 "You selected a format that is not in the valid_formats of this file.");
671 secondary_stream = detail::make_secondary_istream(*primary_stream);
696 if (!first_record_was_read)
699 first_record_was_read =
true;
779 stream_ptr_t primary_stream{
nullptr, stream_deleter_noop};
781 stream_ptr_t secondary_stream{
nullptr, stream_deleter_noop};
784 bool first_record_was_read{
false};
789 using format_type =
typename detail::variant_from_tags<
valid_formats,
790 detail::structure_file_input_format_exposer>::type;
796 void read_next_record()
799 record_buffer.clear();
809 assert(!
format.valueless_by_exception());
816 "You may not select field::structured_seq and field::structure at the same time.");
818 "You may not select field::structured_seq and field::seq at the same time.");
819 f.read_structure_record(*secondary_stream,
821 detail::get_or_ignore<field::structured_seq>(record_buffer),
822 detail::get_or_ignore<field::id>(record_buffer),
823 detail::get_or_ignore<field::bpp>(record_buffer),
824 detail::get_or_ignore<field::structured_seq>(record_buffer),
825 detail::get_or_ignore<field::energy>(record_buffer),
826 detail::get_or_ignore<field::react>(record_buffer),
827 detail::get_or_ignore<field::react_err>(record_buffer),
828 detail::get_or_ignore<field::comment>(record_buffer),
829 detail::get_or_ignore<field::offset>(record_buffer));
833 f.read_structure_record(*secondary_stream,
835 detail::get_or_ignore<field::seq>(record_buffer),
836 detail::get_or_ignore<field::id>(record_buffer),
837 detail::get_or_ignore<field::bpp>(record_buffer),
838 detail::get_or_ignore<field::structure>(record_buffer),
839 detail::get_or_ignore<field::energy>(record_buffer),
840 detail::get_or_ignore<field::react>(record_buffer),
841 detail::get_or_ignore<field::react_err>(record_buffer),
842 detail::get_or_ignore<field::comment>(record_buffer),
843 detail::get_or_ignore<field::offset>(record_buffer));
857 template <input_stream stream_type,
860 detail::fields_specialisation selected_field_ids>
867 template <input_stream stream_type,
Provides seqan3::rna15, container aliases and string literals.
A more refined container concept than seqan3::container.
meta::list< types... > type_list
Type that contains multiple types, an alias for meta::list.
Definition: type_list.hpp:31
The protein structure alphabet of the characters "HGIEBTSCX".
Definition: dssp9.hpp:61
@ offset
Sequence (SEQ) relative start position (0-based), unsigned value.
Resolves to std::ranges::explicitly_convertible_to<type1, type2>().
@ id
The identifier, usually a string.
Provides the seqan3::record template and the seqan3::field enum.
This header includes C++17 filesystem support and imports it into namespace std::filesystem (independ...
constexpr bool contains
Whether a type occurs in a pack or not.
Definition: traits.hpp:193
A class template that holds a choice of seqan3::field.
Definition: record.hpp:166
constexpr sequenced_policy seq
Global execution policy object for sequenced execution policy.
Definition: execution.hpp:54
A concept that indicates whether an alphabet represents RNA structure.
Provides seqan3::aa27, container aliases and string literals.
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
The 15 letter RNA alphabet, containing all IUPAC smybols minus the gap.
Definition: rna15.hpp:49
Provides the composite of aminoacid with structure alphabets.
Provides exceptions used in the I/O module.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
The five letter RNA alphabet of A,C,G,U and the unknown character N.
Definition: rna5.hpp:47
Provides alphabet adaptations for standard char types.
Provides the dssp format for protein structure.
Provides seqan3::rna5, container aliases and string literals.
A seqan3::alphabet_tuple_base that joins a nucleotide alphabet with an RNA structure alphabet.
Definition: structured_rna.hpp:55
field
An enumerator for the fields used in file formats.
Definition: record.hpp:65
Provides the seqan3::detail::in_file_iterator class template.
A seqan3::alphabet_tuple_base that joins an aminoacid alphabet with a protein structure alphabet.
Definition: structured_aa.hpp:54
Provides traits for seqan3::type_list.
Refines seqan3::alphabet and adds assignability.
The twenty-seven letter amino acid alphabet.
Definition: aa27.hpp:44