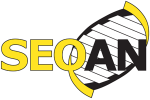 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
16 #include <seqan3/alphabet/detail/convert.hpp>
28 template <
typename derived_type, auto size>
66 template <
typename other_aa_type>
74 if constexpr (is_constexpr_default_constructible_v<other_aa_type> &&
78 detail::writable_constexpr_alphabet<other_aa_type>
82 static_cast<derived_type &>(*
this) =
83 detail::convert_through_char_representation<derived_type, other_aa_type>[
seqan3::to_rank(other)];
111 return valid_char_table[static_cast<uint8_t>(c)];
124 for (uint8_t c : derived_type::rank_to_char)
Provides seqan3::alphabet_base.
Provides seqan3::aminoacid_alphabet.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:174
constexpr auto to_char
Return the char representation of an alphabet object.
Definition: concept.hpp:320
A CRTP-base that makes defining a custom alphabet easier.
Definition: alphabet_base.hpp:51
constexpr auto to_rank
Return the rank representation of a (semi-)alphabet object.
Definition: concept.hpp:142
This is an empty base class that can be inherited by types that shall model seqan3::aminoacid_alphabe...
Definition: concept.hpp:32
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
A concept that indicates whether an alphabet represents amino acids.
detail::min_viable_uint_t< size - 1 > rank_type
The type of the alphabet when represented as a number (e.g. via to_rank()).
Definition: alphabet_base.hpp:62
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr aminoacid_base(other_aa_type const other) noexcept
Allow explicit construction from any other aminoacid type and convert via the character representatio...
Definition: aminoacid_base.hpp:72
std::conditional_t< std::same_as< char, void >, char, char > char_type
The char representation; conditional needed to make semi alphabet definitions legal.
Definition: alphabet_base.hpp:60
constexpr auto assign_char_to
Assign a character to an alphabet object.
Definition: concept.hpp:416
A CRTP-base that refines seqan3::alphabet_base and is used by the amino acids.
Definition: aminoacid_base.hpp:29
constexpr char_type to_lower(char_type const c) noexcept
Converts 'A'-'Z' to 'a'-'z' respectively; other characters are returned as is.
Definition: transform.hpp:81
static constexpr bool char_is_valid(char_type const c) noexcept
Validate whether a character value has a one-to-one mapping to an alphabet value.
Definition: aminoacid_base.hpp:109
constexpr rank_type to_rank() const noexcept
Return the letter's numeric value (rank in the alphabet).
Definition: alphabet_base.hpp:116
Refines seqan3::alphabet and adds assignability.