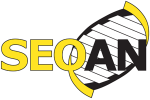 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
96 constexpr
aa10li() noexcept = default;
141 ret[
'D'] = ret[
'B']; ret[
'd'] = ret[
'B'];
142 ret[
'E'] = ret[
'B']; ret[
'e'] = ret[
'B'];
143 ret[
'L'] = ret[
'J']; ret[
'l'] = ret[
'J'];
144 ret[
'M'] = ret[
'J']; ret[
'm'] = ret[
'J'];
145 ret[
'N'] = ret[
'H']; ret[
'n'] = ret[
'H'];
146 ret[
'O'] = ret[
'K']; ret[
'o'] = ret[
'K'];
147 ret[
'Q'] = ret[
'B']; ret[
'q'] = ret[
'B'];
148 ret[
'R'] = ret[
'K']; ret[
'r'] = ret[
'K'];
149 ret[
'S'] = ret[
'A']; ret[
's'] = ret[
'A'];
150 ret[
'T'] = ret[
'A']; ret[
't'] = ret[
'A'];
151 ret[
'U'] = ret[
'C']; ret[
'u'] = ret[
'C'];
152 ret[
'V'] = ret[
'I']; ret[
'v'] = ret[
'I'];
153 ret[
'W'] = ret[
'F']; ret[
'w'] = ret[
'F'];
154 ret[
'X'] = ret[
'A']; ret[
'x'] = ret[
'A'];
155 ret[
'Y'] = ret[
'F']; ret[
'y'] = ret[
'F'];
156 ret[
'Z'] = ret[
'B']; ret[
'z'] = ret[
'B'];
184 constexpr
aa10li operator""_aa10li(
char const c) noexcept
201 inline aa10li_vector operator""_aa10li(
char const *
const s,
size_t const n)
206 for (
size_t i = 0; i < n; ++i)
207 r[i].assign_char(s[i]);
Provides seqan3::aminoacid_alphabet.
static constexpr detail::min_viable_uint_t< size > alphabet_size
The size of the alphabet, i.e. the number of different values it can take.
Definition: alphabet_base.hpp:176
Provides seqan3::aminoacid_base.
constexpr derived_type & assign_char(char_type const c) noexcept
Assign from a character, implicitly converts invalid characters.
Definition: alphabet_base.hpp:142
detail::min_viable_uint_t< size - 1 > rank_type
The type of the alphabet when represented as a number (e.g. via to_rank()).
Definition: alphabet_base.hpp:64
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
constexpr aa10li() noexcept=default
Defaulted.
static constexpr std::array< rank_type, 256 > char_to_rank
Char to value conversion table.
Definition: aa10li.hpp:125
A CRTP-base that refines seqan3::alphabet_base and is used by the amino acids.
Definition: aminoacid_base.hpp:30
constexpr char_type to_lower(char_type const c) noexcept
Converts 'A'-'Z' to 'a'-'z' respectively; other characters are returned as is.
Definition: transform.hpp:81
static constexpr char_type rank_to_char[alphabet_size]
Value to char conversion table.
Definition: aa10li.hpp:110
The reduced Li amino acid alphabet.
Definition: aa10li.hpp:81