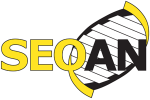 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
16 #include <type_traits>
40 template <
typename value_t,
51 template <
typename ...ends_t>
92 template <
typename value_t>
104 template <
typename value_t>
123 template <
typename value_t>
135 template <
typename value_t>
154 template <
typename value_t>
166 template <
typename value_t>
185 template <
typename value_t>
197 template <
typename value_t>
244 template <
typename ...ends_t>
246 requires
sizeof...(ends_t) <= 4 &&
247 ((detail::is_type_specialisation_of_v<ends_t, front_end_first> ||
248 detail::is_type_specialisation_of_v<ends_t, back_end_first> ||
249 detail::is_type_specialisation_of_v<ends_t, front_end_second> ||
250 detail::is_type_specialisation_of_v<ends_t, back_end_second>) && ...)
255 template <
typename ..._ends_t>
256 static constexpr
bool check_consistency(_ends_t ...ends)
258 if constexpr (
sizeof...(ends) < 2)
264 return [] (
auto head,
auto ...tail) constexpr
266 using head_t = decltype(head);
267 if constexpr (((
head_t::id != decltype(tail)::
id) && ...))
268 return check_consistency(tail...);
275 static_assert(check_consistency(ends_t{}...),
276 "You may not use the same end_gap specifier more than once.");
298 requires
sizeof...(ends_t) > 0
300 detail::for_each([
this](
auto e)
324 assert(pos < values.
size());
340 template <
size_t pos>
343 static_assert(is_static_array[pos],
344 "You may not access an element that was not set in a core constant expression.");
345 return get<pos>(static_values);
361 template <
size_t pos>
364 return get<pos>(is_static_array);
376 [](
auto ...ends) constexpr
379 detail::for_each([&tmp](
auto v)
390 [](
auto ...ends) constexpr
393 detail::for_each([&tmp](
auto v)
395 tmp[decltype(v)::
id()] = decltype(v)::static_value;
410 template <
typename ...ends_t>
411 end_gaps(ends_t
const & ...) -> end_gaps<ends_t...>;
543 template <
typename end_gaps_t>
545 requires detail::is_type_specialisation_of_v<end_gaps_t, end_gaps>
551 static constexpr detail::align_config_id
id{detail::align_config_id::aligned_ends};
558 template <
typename end_gaps_t>
constexpr bool operator[](size_t const pos) const noexcept
Returns the value for the specifier at the given position.
Definition: align_config_aligned_ends.hpp:322
Provides algorithms for meta programming, parameter packs and seqan3::type_list.
The penalty configuration for aligning the front of the second sequence with a gap.
Definition: align_config_aligned_ends.hpp:155
The penalty configuration for aligning the back of the first sequence with a gap.
Definition: align_config_aligned_ends.hpp:124
constexpr end_gaps(ends_t const ...args) noexcept requires sizeof...(ends_t) > 0
Construction from at least one sequence end-gap specifier.
Definition: align_config_aligned_ends.hpp:297
Provides various type traits on generic types.
The identifier, usually a string.
static constexpr bool static_value
Holds the static value if the state is static.
Definition: align_config_aligned_ends.hpp:57
Provides seqan3::type_list and auxiliary type traits.
Provides seqan3::pipeable_config_element.
constexpr end_gaps & operator=(end_gaps const &) noexcept=default
Defaulted.
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
static constexpr bool get_static() noexcept
Returns the static value for the specifier at the given position.
Definition: align_config_aligned_ends.hpp:341
static constexpr bool is_static() noexcept
Returns whether a value at the given position was set statically.
Definition: align_config_aligned_ends.hpp:362
Wraps the sequence end-gap specifiers and provides ordered access to the respective values.
Definition: align_config_aligned_ends.hpp:252
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
A special sub namespace for the alignment configurations.
Definition: align_config_aligned_ends.hpp:514
Adds pipe interface to configuration elements.
Definition: pipeable_config_element.hpp:30
The configuration for aligned sequence ends.
Definition: align_config_aligned_ends.hpp:547
The penalty configuration for aligning the back of the second sequence with a gap.
Definition: align_config_aligned_ends.hpp:186
A mixin class which can maintain a static or a dynamic bool state.
Definition: align_config_aligned_ends.hpp:46
value_t value
The wrapped value.
Definition: align_config_aligned_ends.hpp:68
~end_gaps() noexcept=default
Defaulted.
constexpr end_gaps() noexcept
Default constructor.
Definition: align_config_aligned_ends.hpp:284
The penalty configuration for aligning the front of the first sequence with a gap.
Definition: align_config_aligned_ends.hpp:93
constexpr bool operator()() const noexcept
Returns the wrapped value.
Definition: align_config_aligned_ends.hpp:62
static constexpr bool is_static
Used to differentiate between static and dynamic state.
Definition: align_config_aligned_ends.hpp:55
Provides some utility functions for the alignment configurations.