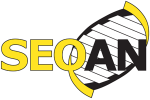 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
18 #include <type_traits>
20 #include <meta/meta.hpp>
133 template <
typename sequence_t,
typename alignment_config_t>
135 requires detail::align_pairwise_single_input<std::remove_reference_t<sequence_t>> &&
137 detail::is_type_specialisation_of_v<alignment_config_t, configuration>
142 "Alignment configuration error: Expects exactly two sequences for pairwise alignments.");
146 "Alignment configuration error: The tuple elements must model std::ranges::viewable_range.");
152 template <
typename sequence_t,
typename alignment_config_t>
153 requires detail::align_pairwise_range_input<sequence_t> &&
154 detail::is_type_specialisation_of_v<alignment_config_t, configuration>
156 alignment_config_t
const & config)
158 using first_seq_t = std::tuple_element_t<0, std::ranges::range_value_t<sequence_t>>;
159 using second_seq_t = std::tuple_element_t<1, std::ranges::range_value_t<sequence_t>>;
161 static_assert(std::ranges::random_access_range<first_seq_t> && std::ranges::sized_range<first_seq_t>,
162 "Alignment configuration error: The sequence must model random_access_range and sized_range.");
163 static_assert(std::ranges::random_access_range<second_seq_t> && std::ranges::sized_range<second_seq_t>,
164 "Alignment configuration error: The sequence must model random_access_range and sized_range.");
167 auto seq_view = std::forward<sequence_t>(sequences) |
views::persist;
169 auto && [algorithm, adapted_config] = detail::alignment_configurator::configure<decltype(seq_view)>(config);
171 using traits_t = detail::alignment_configuration_traits<
remove_cvref_t<decltype(adapted_config)>>;
173 constexpr
auto get_execution_rule = [] ()
175 if constexpr (traits_t::is_parallel)
182 detail::alignment_executor_two_way executor{
std::move(seq_view),
184 traits_t::alignments_per_vector,
185 get_execution_rule()};
187 return alignment_range{
std::move(executor)};
The text is a single range.
Definition: concept.hpp:84
std::remove_cv_t< std::remove_reference_t< t > > remove_cvref_t
Return the input type with const, volatile and references removed (type trait).
Definition: basic.hpp:35
Provides helper type traits for the configuration and execution of the alignment algorithm.
Provides various type traits on generic types.
const auto move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
constexpr auto align_pairwise(sequence_t &&seq, alignment_config_t const &config)
Computes the pairwise alignment for a pair of sequences or a range over sequence pairs.
Definition: align_pairwise.hpp:139
Meta-header for the pairwise execution submodule .
Meta-Header for components of the algorithm submodule.
Provides execution policies.
constexpr auto persist
A view adaptor that wraps rvalue references of non-views.
Definition: persist.hpp:248
constexpr sequenced_policy seq
Global execution policy object for sequenced execution policy.
Definition: execution.hpp:54
Provides seqan3::simd::simd_type.
Provides seqan3::simd::simd_traits.
constexpr parallel_policy par
Global execution policy object for parallel execution policy.
Definition: execution.hpp:59
Provides concepts needed internally for the alignment algorithms.
Provides seqan3::detail::alignment_selector.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
Provides seqan3::views::persist.
Provides seqan3::alignment_result.
Meta-header for the alignment configuration module .
Adaptations of concepts from the Ranges TS.
The concept std::copy_constructible is satisfied if T is an lvalue reference type,...