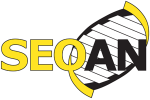 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
40 template <
typename alignment_executor_type>
43 static_assert(!std::is_const_v<alignment_executor_type>,
44 "Cannot create an alignment stream over a const buffer.");
55 using value_type =
typename alignment_executor_type::value_type;
57 using reference =
typename alignment_executor_type::reference;
63 using sentinel = std::ranges::default_sentinel_t;
76 explicit alignment_range(alignment_executor_type
const & _alignment_executor) =
delete;
88 alignment_executor{
new alignment_executor_type{
std::move(_alignment_executor)}}
125 constexpr
sentinel cend()
const =
delete;
139 if (!alignment_executor)
142 if (
auto opt = alignment_executor->bump(); opt.has_value())
163 template <
typename alignment_executor_type>
165 alignment_range(alignment_executor_type &&) -> alignment_range<std::remove_reference_t<alignment_executor_type>>;
171 template <
typename alignment_executor_type>
213 return range_ptr->cache;
219 return &range_ptr->cache;
229 assert(range_ptr !=
nullptr);
231 at_end = !range_ptr->next();
247 std::ranges::default_sentinel_t
const &) noexcept
253 friend constexpr
bool operator==(std::ranges::default_sentinel_t
const & lhs,
261 std::ranges::default_sentinel_t
const & rhs) noexcept
263 return !(lhs == rhs);
267 friend constexpr
bool operator!=(std::ranges::default_sentinel_t
const & lhs,
bool next()
Receives the next alignment result from the executor buffer.
Definition: alignment_range.hpp:137
std::ranges::default_sentinel_t sentinel
The sentinel type.
Definition: alignment_range.hpp:63
pointer operator->() const noexcept
Returns a pointer to the current alignment result.
Definition: alignment_range.hpp:217
constexpr sentinel end() noexcept
Returns a sentinel signaling the end of the alignment range.
Definition: alignment_range.hpp:119
const auto move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
friend class alignment_range_iterator
Befriend the iterator type.
Definition: alignment_range.hpp:46
constexpr friend bool operator==(std::ranges::default_sentinel_t const &lhs, alignment_range_iterator const &rhs) noexcept
Checks whether lhs is equal to rhs.
Definition: alignment_range.hpp:253
void operator++(int)
Returns an iterator incremented by one.
Definition: alignment_range.hpp:236
typename alignment_range::difference_type difference_type
Type for distances between iterators.
Definition: alignment_range.hpp:176
alignment_range()=default
Defaulted.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
The iterator of seqan3::detail::alignment_range.
Definition: alignment_range.hpp:172
constexpr friend bool operator!=(std::ranges::default_sentinel_t const &lhs, alignment_range_iterator const &rhs) noexcept
Checks whether lhs is not equal to rhs.
Definition: alignment_range.hpp:267
constexpr friend bool operator!=(alignment_range_iterator const &lhs, std::ranges::default_sentinel_t const &rhs) noexcept
Checks whether *this is not equal to the sentinel.
Definition: alignment_range.hpp:260
~alignment_range()=default
Defaulted.
Adaptations of concepts from the Ranges TS.
An input range over the alignment results generated by the underlying alignment executor.
Definition: alignment_range.hpp:41
alignment_range & operator=(alignment_range const &)=delete
This is a move-only type.
constexpr iterator begin()
Returns an iterator to the first element of the alignment range.
Definition: alignment_range.hpp:103
typename alignment_executor_type::difference_type difference_type
The offset type.
Definition: alignment_range.hpp:53
typename alignment_executor_type::value_type value_type
The alignment result type.
Definition: alignment_range.hpp:55
alignment_range(alignment_executor_type &&_alignment_executor)
Constructs a new alignment range by taking ownership over the passed alignment buffer.
Definition: alignment_range.hpp:87
void const_iterator
This range is never const-iterable. The const_iterator is always void.
Definition: alignment_range.hpp:61
typename alignment_range::reference reference
Use reference type defined by container.
Definition: alignment_range.hpp:180
typename alignment_executor_type::reference reference
The reference type.
Definition: alignment_range.hpp:57
typename alignment_range::value_type value_type
Value type of container elements.
Definition: alignment_range.hpp:178