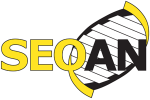 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
20 #include <range/v3/view/chunk.hpp>
98 template <
typename stream_type,
99 typename seq_legal_alph_type,
bool seq_qual_combined,
107 qual_type & SEQAN3_DOXYGEN_ONLY(qualities))
110 auto stream_it = std::ranges::begin(stream_view);
114 std::ranges::back_inserter(idbuffer));
115 if (idbuffer !=
"ID")
116 throw parse_error{
"An entry has to start with the code word ID."};
118 if constexpr (!detail::decays_to_ignore_v<id_type>)
127 std::ranges::back_inserter(
id));
128 id.push_back(*stream_it);
130 }
while (*stream_it !=
'Q');
144 std::ranges::back_inserter(
id));
150 std::ranges::back_inserter(
id));
162 }
while (*stream_it !=
'Q');
167 auto constexpr is_end =
is_char<
'/'> ;
168 if constexpr (!detail::decays_to_ignore_v<seq_type>)
173 auto constexpr is_legal_alph = is_in_alphabet<seq_legal_alph_type>;
176 if (!is_legal_alph(c))
180 " evaluated to false on " +
181 detail::make_printable(c)};
186 std::ranges::back_inserter(sequence));
199 template <
typename stream_type,
205 seq_type && sequence,
207 qual_type && SEQAN3_DOXYGEN_ONLY(qualities))
210 [[maybe_unused]]
size_t sequence_size = 0;
211 [[maybe_unused]]
char buffer[50];
212 if constexpr (!detail::decays_to_ignore_v<seq_type>)
213 sequence_size = std::ranges::distance(sequence);
216 if constexpr (detail::decays_to_ignore_v<id_type>)
218 throw std::logic_error{
"The ID field may not be set to ignore when writing embl files."};
222 if (ranges::empty(
id))
227 std::ranges::copy(
id, stream_it);
232 std::ranges::copy(
id, stream_it);
234 auto res =
std::to_chars(&buffer[0], &buffer[0] +
sizeof(buffer), sequence_size);
235 std::copy(&buffer[0], res.ptr, stream_it);
242 if constexpr (detail::decays_to_ignore_v<seq_type>)
244 throw std::logic_error{
"The SEQ field may not be set to ignore when writing embl files."};
248 if (ranges::empty(sequence))
252 auto res =
std::to_chars(&buffer[0], &buffer[0] +
sizeof(buffer), sequence_size);
253 std::copy(&buffer[0], res.ptr, stream_it);
255 auto seqChunk = sequence | ranges::view::chunk(60);
258 for (
auto chunk : seqChunk)
261 | ranges::view::chunk(10)
262 | views::join(
' '), stream_it);
265 bp =
std::min(sequence_size, bp + 60);
266 uint8_t num_blanks = 60 * i - bp;
267 num_blanks += num_blanks / 10;
Provides seqan3::views::take_until and seqan3::views::take_until_or_throw.
const auto to_char
A view that calls seqan3::to_char() on each element in the input range.
Definition: to_char.hpp:65
Provides std::from_chars and std::to_chars if not defined in the stl <charconv> header.
const auto char_to
A view over an alphabet, given a range of characters.
Definition: char_to.hpp:69
bool embl_genbank_complete_header
Complete header given for embl or genbank.
Definition: output_options.hpp:42
constexpr auto is_space
Checks whether c is a space character.
Definition: predicate.hpp:146
Adaptations of algorithms from the Ranges TS.
Provides seqan3::views::repeat_n.
Provides seqan3::views::to.
Thrown if there is a parse error, such as reading an unexpected character from an input stream.
Definition: exception.hpp:47
Provides various transformation traits used by the range module.
typename value_type< t >::type value_type_t
Shortcut for seqan3::value_type (transformation_trait shortcut).
Definition: pre.hpp:48
Provides seqan3::dna5, container aliases and string literals.
::ranges::ostreambuf_iterator ostreambuf_iterator
Alias for ranges::ostreambuf_iterator. Writes successive characters onto the output stream from which...
Definition: iterator.hpp:204
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr auto take_until
A view adaptor that returns elements from the underlying range until the functor evaluates to true (o...
Definition: take_until.hpp:585
The options type defines various option members that influence the behaviour of all or some formats.
Definition: output_options.hpp:21
constexpr auto is_digit
Checks whether c is a digital character.
Definition: predicate.hpp:287
Provides seqan3::sequence_file_output_options.
constexpr auto is_cntrl
Checks whether c is a control character.
Definition: predicate.hpp:110
Provides seqan3::views::join.
Provides seqan3::views::to_char.
Adaptations of concepts from the Ranges TS.
constexpr auto take_line_or_throw
A view adaptor that returns a single line from the underlying range (throws if there is no end-of-lin...
Definition: take_line.hpp:90
constexpr auto take_until_or_throw
A view adaptor that returns elements from the underlying range until the functor evaluates to true (t...
Definition: take_until.hpp:599
Provides seqan3::views::take_exactly and seqan3::views::take_exactly_or_throw.
Provides character predicates for tokenisation.
Provides seqan3::views::istreambuf.
constexpr auto repeat_n
A view factory that repeats a given value n times.
Definition: repeat_n.hpp:94
seqan3::type_list< trait_t< pack_t >... > transform
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
Definition: traits.hpp:307
Provides seqan3::views::take_line and seqan3::views::take_line_or_throw.
Provides seqan3::ostream and seqan3::ostreambuf iterator.
Provides various utility functions.
constexpr auto is_char
Checks whether a given letter is the same as the template non-type argument.
Definition: predicate.hpp:83
constexpr auto is_blank
Checks whether c is a blank character.
Definition: predicate.hpp:163
Provides various utility functions.
Provides seqan3::views::char_to.
constexpr auto istreambuf
A view factory that returns a view over the stream buffer of an input stream.
Definition: istreambuf.hpp:113