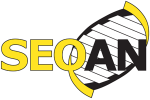 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
101 template <
typename stream_type,
102 typename seq_legal_alph_type,
bool seq_qual_combined,
110 qual_type & qualities)
113 auto stream_it = begin(stream_view);
116 size_t sequence_size_before = 0;
117 size_t sequence_size_after = 0;
118 if constexpr (!detail::decays_to_ignore_v<seq_type>)
119 sequence_size_before =
size(sequence);
122 if (*stream_it !=
'@')
125 detail::make_printable(*stream_it)};
129 if constexpr (!detail::decays_to_ignore_v<id_type>)
135 std::ranges::back_inserter(
id));
142 std::ranges::back_inserter(
id));
153 if constexpr (!detail::decays_to_ignore_v<seq_type>)
155 auto constexpr is_legal_alph = is_in_alphabet<seq_legal_alph_type>;
158 if (!is_legal_alph(c))
162 " evaluated to false on " +
163 detail::make_printable(c)};
168 std::ranges::back_inserter(sequence));
169 sequence_size_after =
size(sequence);
173 auto it = begin(seq_view);
174 auto it_end = end(seq_view);
178 ++sequence_size_after;
183 if (*stream_it !=
'+')
186 detail::make_printable(*stream_it)};
191 auto qview = stream_view | std::views::filter(!
is_space)
193 if constexpr (seq_qual_combined)
198 begin(qualities) + sequence_size_before);
200 else if constexpr (!detail::decays_to_ignore_v<qual_type>)
203 std::ranges::back_inserter(qualities));
207 detail::consume(qview);
212 template <
typename stream_type,
218 seq_type && sequence,
220 qual_type && qualities)
225 if constexpr (detail::decays_to_ignore_v<id_type>)
227 throw std::logic_error{
"The ID field may not be set to ignore when writing FASTQ files."};
235 std::ranges::copy(
id, stream_it);
241 if constexpr (detail::decays_to_ignore_v<seq_type>)
243 throw std::logic_error{
"The SEQ and SEQ_QUAL fields may not both be set to ignore when writing FASTQ files."};
256 if constexpr (!detail::decays_to_ignore_v<id_type>)
261 std::ranges::copy(
id, stream_it);
267 if constexpr (detail::decays_to_ignore_v<qual_type>)
269 throw std::logic_error{
"The QUAL and SEQ_QUAL fields may not both be set to ignore when writing FASTQ files."};
273 if (empty(qualities))
276 if constexpr (std::ranges::sized_range<seq_type> && std::ranges::sized_range<qual_type>)
278 assert(
size(sequence) ==
size(qualities));
constexpr auto take_exactly_or_throw
A view adaptor that returns the first size elements from the underlying range and also exposes size i...
Definition: take_exactly.hpp:91
Provides various shortcuts for common std::ranges functions.
Provides seqan3::views::take_until and seqan3::views::take_until_or_throw.
const auto to_char
A view that calls seqan3::to_char() on each element in the input range.
Definition: to_char.hpp:65
const auto char_to
A view over an alphabet, given a range of characters.
Definition: char_to.hpp:69
bool fastq_double_id
Whether to write the ID only '@' or also after '+' line.
Definition: output_options.hpp:34
constexpr auto is_space
Checks whether c is a space character.
Definition: predicate.hpp:146
Adaptations of algorithms from the Ranges TS.
Thrown if there is a parse error, such as reading an unexpected character from an input stream.
Definition: exception.hpp:47
Provides various transformation traits used by the range module.
typename value_type< t >::type value_type_t
Shortcut for seqan3::value_type (transformation_trait shortcut).
Definition: pre.hpp:48
Provides seqan3::dna5, container aliases and string literals.
::ranges::ostreambuf_iterator ostreambuf_iterator
Alias for ranges::ostreambuf_iterator. Writes successive characters onto the output stream from which...
Definition: iterator.hpp:204
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
The options type defines various option members that influence the behaviour of all or some formats.
Definition: output_options.hpp:21
bool add_carriage_return
The default plain text line-ending is "\n", but on Windows an additional carriage return is recommend...
Definition: output_options.hpp:39
Provides seqan3::sequence_file_output_options.
constexpr auto is_cntrl
Checks whether c is a control character.
Definition: predicate.hpp:110
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
Provides alphabet adaptations for standard char types.
Provides seqan3::views::to_char.
Provides seqan3::views::take.
Adaptations of concepts from the Ranges TS.
constexpr auto take_line_or_throw
A view adaptor that returns a single line from the underlying range (throws if there is no end-of-lin...
Definition: take_line.hpp:90
constexpr auto take_until_or_throw
A view adaptor that returns elements from the underlying range until the functor evaluates to true (t...
Definition: take_until.hpp:599
Provides seqan3::views::take_exactly and seqan3::views::take_exactly_or_throw.
Provides character predicates for tokenisation.
Provides seqan3::detail::ignore_output_iterator for writing to null stream.
Provides aliases for qualified.
Provides seqan3::views::istreambuf.
seqan3::type_list< trait_t< pack_t >... > transform
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
Definition: traits.hpp:307
Provides seqan3::views::take_line and seqan3::views::take_line_or_throw.
Provides seqan3::ostream and seqan3::ostreambuf iterator.
Provides various utility functions.
constexpr auto is_blank
Checks whether c is a blank character.
Definition: predicate.hpp:163
Provides various utility functions.
Provides seqan3::views::char_to.
constexpr auto istreambuf
A view factory that returns a view over the stream buffer of an input stream.
Definition: istreambuf.hpp:113