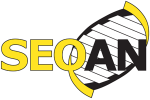 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
19 #include <type_traits>
79 template <std::ranges::viewable_range inner_type>
81 requires std::ranges::random_access_range<inner_type> && std::ranges::sized_range<inner_type> &&
82 (std::is_const_v<std::remove_reference_t<inner_type>> || std::ranges::view<inner_type>)
134 template <
typename other_range_t>
138 std::ranges::viewable_range<other_range_t>
158 return anchors.rbegin()->second + ungapped_view.size();
160 return ungapped_view.size();
182 assert(pos <=
size());
184 set_iterator_type it_set = anchors.upper_bound(anchor_gap_t{pos, bound_dummy});
186 if (it_set == anchors.begin())
188 anchors.emplace_hint(anchors.begin(), anchor_gap_t{pos,
count});
193 auto gap_len{it_set->second};
194 if (it_set != anchors.begin())
195 gap_len -= (*(
std::prev(it_set))).second;
197 if (it_set->first + gap_len >= pos)
199 anchor_gap_t
gap{it_set->first, it_set->second +
count};
200 it_set = anchors.erase(it_set);
201 anchors.insert(it_set,
gap);
205 anchor_gap_t
gap{pos, it_set->second +
count};
207 anchors.insert(it_set,
gap);
231 throw gap_erase_failure(
"The range to be erased does not correspond to a consecutive gap.");
253 set_iterator_type it = anchors.upper_bound(anchor_gap_t{pos1, bound_dummy});
255 if (it == anchors.begin())
260 size_type const gap_len = gap_length(it);
263 if ((it->first + gap_len) < pos2)
265 throw gap_erase_failure{
"The range to be erased does not correspond to a consecutive gap."};
268 else if (gap_len == pos2 - pos1)
270 it = anchors.erase(it);
275 anchor_gap_t
gap{it->first, it->second - pos2 + pos1};
276 it = anchors.erase(it);
277 it = anchors.insert(it,
gap);
282 update(it, pos2 - pos1);
291 template <
typename unaligned_seq_t>
371 throw std::out_of_range{
"Trying to access element behind the last in gap_decorator."};
379 throw std::out_of_range{
"Trying to access element behind the last in gap_decorator."};
422 if (lhs.size() == rhs.size() &&
423 lhs.anchors == rhs.anchors &&
424 std::ranges::equal(lhs.ungapped_view, rhs.ungapped_view))
435 return !(lhs == rhs);
441 auto lit = lhs.begin();
442 auto rit = rhs.begin();
444 while (lit != lhs.end() && rit != rhs.end() && *lit == *rit)
447 if (rit == rhs.end())
449 else if (lit == lhs.end())
458 auto lit = lhs.begin();
459 auto rit = rhs.begin();
461 while (lit != lhs.end() && rit != rhs.end() && *lit == *rit)
464 if (lit == lhs.end())
466 else if (rit == rhs.end())
475 return !(lhs <= rhs);
493 using set_iterator_type =
typename anchor_set_type::iterator;
510 constexpr size_type gap_length(set_iterator_type it)
const noexcept
512 return (it == anchors.begin()) ? it->second : it->second - (*
std::prev(it)).second;
527 void rupdate(size_type
const pos, size_type
const offset)
529 for (
auto it =
std::prev(anchors.end(), 1); it->first > pos;)
531 anchors.emplace_hint(it, anchor_gap_t{it->first +
offset, it->second +
offset});
532 anchors.erase(*it--);
548 void update(set_iterator_type it, size_type
const offset)
550 while (it != anchors.end())
552 anchor_gap_t gap{it->first -
offset, it->second -
offset};
553 it = anchors.erase(it);
554 it = anchors.insert(it, gap);
563 anchor_set_type anchors{};
569 template <std::ranges::viewable_range urng_t>
572 requires !std::ranges::view<std::remove_reference_t<urng_t>>
574 gap_decorator(urng_t && range) -> gap_decorator<std::remove_reference_t<urng_t>
const &>;
577 template <std::ranges::view urng_t>
597 template <std::ranges::viewable_range inner_type>
599 requires std::ranges::random_access_range<inner_type> && std::ranges::sized_range<inner_type> &&
600 (std::is_const_v<std::remove_reference_t<inner_type>> || std::ranges::view<inner_type>)
610 int64_t ungapped_view_pos{0};
616 typename gap_decorator::set_iterator_type anchor_set_it{};
618 bool is_at_gap{
true};
623 assert(new_pos <= host->
size());
626 anchor_set_it = host->anchors.upper_bound(anchor_gap_t{pos, host->bound_dummy});
627 ungapped_view_pos = pos;
629 if (anchor_set_it != host->anchors.begin())
631 typename gap_decorator::set_iterator_type prev{
std::prev(anchor_set_it)};
634 if (prev != host->anchors.begin())
637 ungapped_view_pos -= prev->second;
638 left_gap_end = prev->first + gap_len;
641 if (ungapped_view_pos != static_cast<int64_t>(host->ungapped_view.size()) &&
642 pos >= left_gap_end && (anchor_set_it == host->anchors.end() || pos < anchor_set_it->first))
676 host(&host_), anchor_set_it{host_.anchors.begin()}
678 if (host_.anchors.size() && (*host_.anchors.begin()).first == 0)
681 left_gap_end = anchor_set_it->second;
707 if (pos < left_gap_end)
710 if (anchor_set_it == host->anchors.end() || pos < anchor_set_it->first)
713 if (ungapped_view_pos != static_cast<int64_t>(host->ungapped_view.size()))
718 left_gap_end = anchor_set_it->first + anchor_set_it->second -
719 ((anchor_set_it != host->anchors.begin()) ? (
std::prev(anchor_set_it))->second : 0);
723 if (left_gap_end == host->size())
741 this->jump(this->pos + skip);
764 if (pos < left_gap_end)
766 (anchor_set_it != host->anchors.begin()) ? --anchor_set_it : anchor_set_it;
768 if (anchor_set_it != host->anchors.begin())
771 left_gap_end = prev->first + prev->second -
772 ((prev != host->anchors.begin()) ?
std::prev(prev)->second : 0);
780 else if (anchor_set_it == host->anchors.end() || pos < anchor_set_it->first)
801 this->jump(this->pos - skip);
821 return static_cast<difference_type>(this->pos - lhs.pos);
828 constexpr
reference operator*() const noexcept
831 return (is_at_gap) ?
reference{
gap{}} : reference{host->ungapped_view[ungapped_view_pos]};
850 return lhs.pos == rhs.pos;
857 return lhs.pos != rhs.pos;
864 return lhs.pos < rhs.pos;
871 return lhs.pos > rhs.pos;
878 return lhs.pos <= rhs.pos;
885 return lhs.pos >= rhs.pos;
892 namespace seqan3::detail
896 template <
typename type>
897 constexpr
int enable_view<seqan3::gap_decorator<type>> = 0;
899 template <
typename type>
900 constexpr
int enable_view<seqan3::gap_decorator<type>
const> = 0;
constexpr friend bool operator>=(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is greater than or equal to rhs.
Definition: gap_decorator.hpp:882
typename difference_type< t >::type difference_type_t
Shortcut for seqan3::difference_type (transformation_trait shortcut).
Definition: pre.hpp:166
const_iterator cbegin() const noexcept
Returns an iterator to the first element of the container.
Definition: gap_decorator.hpp:323
The alphabet of a gap character '-'.
Definition: gap.hpp:36
aligned_seq_t::iterator erase_gap(aligned_seq_t &aligned_seq, typename aligned_seq_t::const_iterator pos_it)
An implementation of seqan3::aligned_sequence::erase_gap for sequence containers.
Definition: aligned_sequence_concept.hpp:304
gapped< value_type_t< inner_type > > value_type
The variant type of the alphabet type and gap symbol type (see seqan3::gapped).
Definition: gap_decorator.hpp:101
friend bool operator>(gap_decorator const &lhs, gap_decorator const &rhs) noexcept
Checks whether lhs is greater than rhs.
Definition: gap_decorator.hpp:473
constexpr reference operator[](size_type const i) const noexcept
Return the i-th element as a reference.
Definition: gap_decorator.hpp:394
constexpr gap_decorator_iterator operator++(int) noexcept
Returns an incremented iterator copy.
Definition: gap_decorator.hpp:731
Sequence (SEQ) relative start position (0-based), unsigned value.
Thrown in function seqan3::erase_gap, if a position does not contain a gap.
Definition: exception.hpp:23
size_type size() const noexcept
Returns the total length of the aligned sequence.
Definition: gap_decorator.hpp:155
inner_type unaligned_seq_type
The underlying ungapped range type.
Definition: gap_decorator.hpp:114
friend bool operator>=(gap_decorator const &lhs, gap_decorator const &rhs) noexcept
Checks whether lhs is greater than or equal to rhs.
Definition: gap_decorator.hpp:479
const_iterator begin() const noexcept
Returns an iterator to the first element of the container.
Definition: gap_decorator.hpp:317
typename size_type< t >::type size_type_t
Shortcut for seqan3::size_type (transformation_trait shortcut).
Definition: pre.hpp:195
constexpr friend bool operator>(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is greater than rhs.
Definition: gap_decorator.hpp:868
Adaptations of concepts from the standard library.
const_iterator end() const noexcept
Returns an iterator pointing behind the last element of the decorator.
Definition: gap_decorator.hpp:341
constexpr auto type_reduce
A view adaptor that behaves like std::views::all, but type erases certain ranges.
Definition: type_reduce.hpp:158
Includes customized exception types for the alignment module .
Adaptations of algorithms from the Ranges TS.
constexpr gap_decorator_iterator & operator+=(difference_type const skip) noexcept
Advances iterator by skip many positions.
Definition: gap_decorator.hpp:739
const_reference at(size_type const i) const
Return the i-th element as a reference.
Definition: gap_decorator.hpp:376
typename gap_decorator::const_reference reference
The reference type.
Definition: gap_decorator.hpp:657
A combined alphabet that can hold values of either of its alternatives.
Definition: alphabet_variant.hpp:129
The concept std::same_as<T, U> is satisfied if and only if T and U denote the same type.
constexpr gap_decorator_iterator & operator--() noexcept
Decrements iterator.
Definition: gap_decorator.hpp:759
constexpr gap_decorator_iterator & operator-=(difference_type const skip) noexcept
Advances iterator by skip many positions.
Definition: gap_decorator.hpp:799
friend bool operator<=(gap_decorator const &lhs, gap_decorator const &rhs) noexcept
Checks whether lhs is less than or equal to rhs.
Definition: gap_decorator.hpp:456
constexpr friend bool operator<(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is less than rhs.
Definition: gap_decorator.hpp:861
Provides the seqan3::detail::random_access_iterator class.
constexpr friend bool operator<=(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is less than or equal to rhs.
Definition: gap_decorator.hpp:875
value_type * pointer
The pointer type.
Definition: gap_decorator.hpp:659
iterator erase_gap(const_iterator const it)
Erase one gap symbol at the indicated iterator postion.
Definition: gap_decorator.hpp:227
constexpr gap_decorator_iterator(gap_decorator const &host_, typename gap_decorator::size_type const pos_) noexcept
Construct from seqan3::gap_decorator and explicit position.
Definition: gap_decorator.hpp:691
constexpr gap_decorator_iterator operator--(int) noexcept
Returns a decremented iterator copy.
Definition: gap_decorator.hpp:791
Provides seqan3::views::type_reduce.
constexpr gap_decorator_iterator operator+(difference_type const skip) const noexcept
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:746
reference at(size_type const i)
Return the i-th element as a reference.
Definition: gap_decorator.hpp:368
gap_decorator(other_range_t &&range)
Construct with the ungapped range type.
Definition: gap_decorator.hpp:140
friend bool operator!=(gap_decorator const &lhs, gap_decorator const &rhs) noexcept
Checks whether lhs is not equal to rhs.
Definition: gap_decorator.hpp:433
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
The concept std::assignable_from<LHS, RHS> specifies that an expression of the type and value categor...
constexpr friend gap_decorator_iterator operator-(difference_type const skip, gap_decorator_iterator const &it) noexcept
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:812
size_type_t< inner_type > size_type
The size_type of the underlying sequence.
Definition: gap_decorator.hpp:108
typename gap_decorator::value_type value_type
The value type.
Definition: gap_decorator.hpp:655
void jump(typename gap_decorator::size_type const new_pos)
A helper function that performs the random access into the anchor set, updating all member variables.
Definition: gap_decorator.hpp:621
constexpr gap_decorator_iterator(gap_decorator const &host_) noexcept
Construct from seqan3::gap_decorator and initialising to first position.
Definition: gap_decorator.hpp:675
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
Adaptations of concepts from the Ranges TS.
difference_type_t< inner_type > difference_type
The difference type of the underlying sequence.
Definition: gap_decorator.hpp:110
iterator erase_gap(const_iterator const first, const_iterator const last)
Erase gap symbols at the iterator postions [first, last[.
Definition: gap_decorator.hpp:249
iterator insert_gap(const_iterator const it, size_type const count=1)
Insert a gap of length count at the aligned sequence iterator position.
Definition: gap_decorator.hpp:176
The iterator type over a seqan3::gap_decorator.
Definition: gap_decorator.hpp:602
friend bool operator<(gap_decorator const &lhs, gap_decorator const &rhs) noexcept
Checks whether lhs is less than rhs.
Definition: gap_decorator.hpp:439
friend void assign_unaligned(gap_decorator &dec, unaligned_seq_t &&unaligned)
Assigns a new sequence of type seqan3::gap_decorator::unaligned_seq_type to the decorator.
Definition: gap_decorator.hpp:295
A gap decorator allows the annotation of sequences with gap symbols while leaving the underlying sequ...
Definition: gap_decorator.hpp:84
constexpr gap_decorator_iterator operator-(difference_type const skip) const noexcept
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:806
typename gap_decorator::difference_type difference_type
The difference type.
Definition: gap_decorator.hpp:653
Provides the seqan3::detail::inherited_iterator_base template.
constexpr difference_type operator-(gap_decorator_iterator const lhs) const noexcept
Returns the distance between two iterators.
Definition: gap_decorator.hpp:819
constexpr friend bool operator!=(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is not equal to rhs.
Definition: gap_decorator.hpp:854
Core alphabet concept and free function/type trait wrappers.
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134
const_iterator cend() const noexcept
Returns an iterator pointing behind the last element of the decorator.
Definition: gap_decorator.hpp:347
gap_decorator(urng_t &&range) -> gap_decorator< std::remove_reference_t< urng_t > const & >
Ranges (not views!) always deduce to const & range_type since they are access-only anyway.
constexpr friend gap_decorator_iterator operator+(difference_type const skip, gap_decorator_iterator const &it) noexcept
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:752
constexpr reference operator[](difference_type const n) const noexcept
Return underlying container value currently pointed at.
Definition: gap_decorator.hpp:835
reference const_reference
const_reference type equals reference type equals value type because the underlying sequence must not...
Definition: gap_decorator.hpp:106