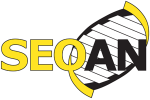 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
33 template <arithmetic score_type>
34 struct gap_score : detail::strong_type<score_type, gap_score<score_type>, detail::strong_type_skill::convert>
36 using detail::strong_type<score_type, gap_score<score_type>, detail::strong_type_skill::convert>::strong_type;
44 template <arithmetic score_type>
59 template <arithmetic score_type>
60 struct gap_open_score : detail::strong_type<score_type, gap_open_score<score_type>, detail::strong_type_skill::convert>
62 using detail::strong_type<score_type, gap_open_score<score_type>, detail::strong_type_skill::convert>::strong_type;
70 template <arithmetic score_type>
83 template <arithmetic score_t =
int8_t>
108 template <arithmetic score_arg_t>
117 template <arithmetic score_arg_t>
141 template <arithmetic score_arg_t>
149 throw std::invalid_argument{
"You passed a score value to set_affine/set_linear that is out of range of the "
150 "scoring scheme's underlying type. Define your scoring scheme with a larger "
151 "template parameter or down-cast your score value beforehand to prevent "
155 gap = static_cast<score_arg_t>(g);
156 gap_open = static_cast<score_arg_t>(go);
169 template <arithmetic score_arg_t>
209 constexpr ptrdiff_t
score(
size_t const number_of_consecutive_gaps)
const noexcept
211 return (gap_open * (number_of_consecutive_gaps ? 1 : 0)) + number_of_consecutive_gaps *
gap;
228 return !(*
this == rhs);
239 template <cereal_archive archive_t>
240 void CEREAL_SERIALIZE_FUNCTION_NAME(archive_t & archive)
251 score_t gap_open = 0;
259 gap_scheme() -> gap_scheme<int8_t>;
266 template <
floating_po
int score_arg_type>
267 gap_scheme(gap_score<score_arg_type>, gap_open_score<score_arg_type>) -> gap_scheme<float>;
273 template <
floating_po
int score_arg_type>
274 gap_scheme(gap_score<score_arg_type>) -> gap_scheme<float>;
280 template <arithmetic score_arg_type>
281 gap_scheme(gap_score<score_arg_type>, gap_open_score<score_arg_type>) -> gap_scheme<int8_t>;
287 template <arithmetic score_arg_type>
288 gap_scheme(gap_score<score_arg_type>) -> gap_scheme<int8_t>;
constexpr ptrdiff_t score(size_t const number_of_consecutive_gaps) const noexcept
Compute the score of a stretch of gap characters.
Definition: gap_scheme.hpp:209
The alphabet of a gap character '-'.
Definition: gap.hpp:36
constexpr score_t get_gap_score() const noexcept
A strong type of underlying type score_type that represents the score of any character against a gap ...
Definition: gap_scheme.hpp:187
constexpr bool operator!=(gap_scheme const &rhs) const noexcept
Checks whether *this is not equal to rhs.
Definition: gap_scheme.hpp:226
constexpr void set_linear(gap_score< score_arg_t > const g)
Set the Linear gap costs model.
Definition: gap_scheme.hpp:170
constexpr gap_scheme(gap_score< score_arg_t > const g)
Constructor for the Linear gap costs model (delegates to set_linear()).
Definition: gap_scheme.hpp:118
A scheme for representing and computing scores against gap characters.
Definition: gap_scheme.hpp:84
Provides basic data structure for strong types.
constexpr score_t get_gap_open_score() const noexcept
A strong type of underlying type score_type that represents an additional score (usually negative) th...
Definition: gap_scheme.hpp:200
A strong type of underlying type score_type that represents an additional score (usually negative) th...
Definition: gap_scheme.hpp:60
constexpr gap_scheme(gap_score< score_arg_t > const g, gap_open_score< score_arg_t > const go)
Constructor for the Affine gap costs model (delegates to set_affine()).
Definition: gap_scheme.hpp:109
A strong type of underlying type score_type that represents the score of any character against a gap ...
Definition: gap_scheme.hpp:34
constexpr gap_scheme & operator=(gap_scheme const &) noexcept=default
Defaulted.
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr score_t & get_gap_open_score() noexcept
Return the gap open score.
Definition: gap_scheme.hpp:194
constexpr gap_scheme() noexcept=default
Defaulted.
Adaptions of concepts from the Cereal library.
constexpr bool operator==(gap_scheme const &rhs) const noexcept
Checks whether *this is equal to rhs.
Definition: gap_scheme.hpp:220
~gap_scheme() noexcept=default
Defaulted.
score_t score_type
The template parameter exposed as member type.
Definition: gap_scheme.hpp:92
constexpr score_t & get_gap_score() noexcept
Return the gap score.
Definition: gap_scheme.hpp:181
constexpr void set_affine(gap_score< score_arg_t > const g, gap_open_score< score_arg_t > const go)
Set the Affine gap costs model.
Definition: gap_scheme.hpp:142