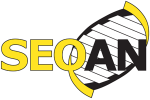 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
17 #include <meta/meta.hpp>
164 template <
field ...fs>
175 static constexpr
size_t index_of(
field f)
177 for (
size_t i = 0; i <
sizeof...(fs); ++i)
178 if (as_array[i] == f)
184 static constexpr
bool contains(
field f)
186 return index_of(f) != npos;
189 static_assert([] () constexpr
191 for (
size_t i = 0; i < as_array.
size(); ++i)
192 for (
size_t j = i + 1; j < as_array.
size(); ++j)
193 if (as_array[i] == as_array[j])
197 } (),
"You may not include a field twice into fields<>.");
224 template <
typename field_types,
typename field_
ids>
225 struct record : detail::transfer_template_args_onto_t<field_types, std::tuple>
229 using base_type = detail::transfer_template_args_onto_t<field_types, std::tuple>;
242 using base_type::base_type;
246 "You must give as many IDs as types to seqan3::record.");
255 template <
size_t ...Is>
258 ((std::get<Is>(tup) = {}), ...);
272 template <
typename field_types,
typename field_
ids>
273 struct tuple_size<
seqan3::record<field_types, field_ids>>
276 static constexpr
size_t value = tuple_size_v<typename seqan3::record<field_types, field_ids>::base_type>;
284 template <
size_t elem_no,
typename field_types,
typename field_
ids>
285 struct tuple_element<elem_no,
seqan3::record<field_types, field_ids>>
288 using type = std::tuple_element_t<elem_no, typename seqan3::record<field_types, field_ids>::base_type>;
302 template <field f,
typename field_types,
typename field_
ids>
306 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
307 return std::get<field_ids::index_of(f)>(r);
311 template <field f,
typename field_types,
typename field_
ids>
314 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
315 return std::get<field_ids::index_of(f)>(r);
319 template <field f,
typename field_types,
typename field_
ids>
322 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
323 return std::get<field_ids::index_of(f)>(
std::move(r));
327 template <field f,
typename field_types,
typename field_
ids>
330 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
331 return std::get<field_ids::index_of(f)>(
std::move(r));
std::tuple_element_t< elem_no, typename seqan3::record< field_types, field_ids >::base_type > type
The member type. Delegates to same type on base_type.
Definition: record.hpp:288
Sequence and qualities combined in one range.
record()=default
Defaulted.
const auto & get(record< field_types, field_ids > const &r)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: record.hpp:312
Please use the field name in lower case.
The "sequence", usually a range of nucleotides or amino acids.
Please use the field name in lower case.
Please use the field name in lower case.
Identifier for user defined file formats and specialisations.
Please use the field name in lower case.
Identifier for user defined file formats and specialisations.
Sequence (SEQ) relative start position (0-based), unsigned value.
Please use the field name in lower case.
Base pair probability matrix of interactions, usually a matrix of float numbers.
Identifier for user defined file formats and specialisations.
Please use the field name in lower case.
Identifier for user defined file formats and specialisations.
Please use the field name in lower case.
Please use the field name in lower case.
The identifier, usually a string.
Identifier for user defined file formats and specialisations.
const auto move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
Please use the field name in lower case.
Identifier for user defined file formats and specialisations.
Provides seqan3::type_list and auxiliary type traits.
Fixed interactions, usually a string of structure alphabet characters.
Please use the field name in lower case.
The bit score (statistical significance indicator), unsigned value.
Please use the field name in lower case.
The (reference) "sequence" information, usually a range of nucleotides or amino acids.
Sequence (REF_SEQ) relative start position (0-based), unsigned value.
constexpr bool contains
Whether a type occurs in a pack or not.
Definition: traits.hpp:193
Please use the field name in lower case.
Please use the field name in lower case.
A class template that holds a choice of seqan3::field.
Definition: record.hpp:165
Energy of a folded sequence, represented by one float number.
Please use the field name in lower case.
Please use the field name in lower case.
Identifier for user defined file formats and specialisations.
Please use the field name in lower case.
The cigar vector (std::vector<seqan3::cigar>) representing the alignment in SAM/BAM format.
Please use the field name in lower case.
Please use the field name in lower case.
The mapping quality of the SEQ alignment, usually a ohred-scaled score.
Please use the field name in lower case.
Comment field of arbitrary content, usually a string.
Please use the field name in lower case.
auto & get(record< field_types, field_ids > &r)
Free function get() for seqan3::record based on seqan3::field.
Definition: record.hpp:304
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
Identifier for user defined file formats and specialisations.
void clear()
(Re-)Initialise all tuple elements with {}.
Definition: record.hpp:249
record & operator=(record const &)=default
Defaulted.
The optional tags in the SAM format, stored in a dictionary.
Please use the field name in lower case.
auto && get(record< field_types, field_ids > &&r)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: record.hpp:320
Sequence and fixed interactions combined in one range.
const auto && get(record< field_types, field_ids > const &&r)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: record.hpp:328
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
Please use the field name in lower case.
Please use the field name in lower case.
Reactivity values of the sequence characters given in a vector of float numbers.
SeqAn specific customisations in the standard namespace.
Identifier for user defined file formats and specialisations.
The identifier of the (reference) sequence that SEQ was aligned to.
field
An enumerator for the fields used in file formats.
Definition: record.hpp:64
The class template that file records are based on; behaves like an std::tuple.
Definition: record.hpp:225
Please use the field name in lower case.
detail::transfer_template_args_onto_t< field_types, std::tuple > base_type
A specialisation of std::tuple.
Definition: record.hpp:229
Please use the field name in lower case.
Please use the field name in lower case.
The qualities, usually in phred-score notation.
Reactivity error values given in a vector corresponding to REACT.
Please use the field name in lower case.
Please use the field name in lower case.
Please use the field name in lower case.
Please use the field name in lower case.
Please use the field name in lower case.
The alignment flag (bit information), uint16_t value.
Identifier for user defined file formats and specialisations.
~record()=default
Defaulted.
Please use the field name in lower case.
Please use the field name in lower case.
Please use the field name in lower case.
A pointer to the seqan3::alignment_file_header object storing header information.
The e-value (length normalized bit score), double value.
The (pairwise) alignment stored in an seqan3::alignment object.
Provides seqan3::type_list.
The mate pair information given as a std::tuple of reference name, offset and template length.