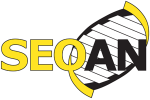 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
22 namespace seqan3::pack_traits::detail
31 template <
typename query_t,
typename ...pack_t>
32 constexpr ptrdiff_t
find()
35 return ((
SEQAN3_IS_SAME(query_t, pack_t) ?
false : ++i) && ...) ? -1 : i;
44 template <
template <
typename>
typename pred_t,
typename ...pack_t>
48 return ((pred_t<pack_t>::value ?
false : ++i) && ...) ? -1 : i;
57 template <ptrdiff_t idx,
typename head_t,
typename ...tail_t>
60 if constexpr (idx == 0)
61 return
std::type_identity<head_t>{};
62 else if constexpr (idx > 0)
66 return at<idx - 1, tail_t...>();
69 return at<
sizeof...(tail_t) + 1 + idx, head_t, tail_t...>();
77 template <
typename head_t,
typename ...tail_t>
85 template <
typename head_t,
typename ...tail_t>
115 template <
typename ...pack_t>
116 inline constexpr
size_t size =
sizeof...(pack_t);
133 template <
typename query_t,
typename ...pack_t>
151 template <
typename query_t,
typename ...pack_t>
152 inline constexpr ptrdiff_t
find = seqan3::pack_traits::detail::find<query_t, pack_t...>();
174 template <
template <
typename>
typename pred_t,
typename ...pack_t>
175 inline constexpr ptrdiff_t
find_if = seqan3::pack_traits::detail::find_if<pred_t, pack_t...>();
192 template <
typename query_t,
typename ...pack_t>
216 template <ptrdiff_t idx,
typename ...pack_t>
218 requires (idx >= 0 && idx <
sizeof...(pack_t)) ||
219 (-idx <=
sizeof...(pack_t))
221 using at =
typename decltype(detail::at<idx, pack_t...>())::type;
236 template <
typename ...pack_t>
238 requires (
sizeof...(pack_t) > 0)
240 using
front = typename decltype(detail::
front<pack_t...>())::type;
258 template <typename ...pack_t>
260 requires (sizeof...(pack_t) > 0)
262 using
back = typename decltype((
std::type_identity<pack_t>{}, ...))::type;
283 template <
typename ...pack_t>
285 requires (
sizeof...(pack_t) > 0)
306 template <template <typename> typename trait_t, typename ...pack_t>
317 namespace
seqan3::list_traits::detail
325 template <ptrdiff_t idx,
typename ...pack_t>
332 template <
typename ...pack_t>
339 template <
typename ...pack_t>
347 template <
typename ...pack1_t,
357 template <
typename ...pack1_t,
359 typename ...more_lists_t>
369 template <
typename ...pack_t>
379 template <ptrdiff_t idx,
381 typename head_t,
typename ...pack2_t>
384 if constexpr (idx ==
sizeof...(pack2_t) + 1)
386 else if constexpr (idx == 0)
397 template <
template <
typename>
typename trait_t,
typename ...pack_t>
407 template <
typename replace_t,
409 typename head_t,
typename ...pack2_t>
410 type_list<pack1_t..., replace_t, pack2_t...>
429 template <seqan3::detail::type_list_specialisation list_t>
431 inline constexpr
size_t size = 0;
440 template <
typename ...pack_t>
444 template <
typename query_t, seqan3::detail::type_list_specialisation list_t>
445 inline constexpr ptrdiff_t
count = -1;
454 template <
typename query_t,
typename ...pack_t>
459 template <
typename query_t, seqan3::detail::type_list_specialisation list_t>
460 inline constexpr ptrdiff_t
find = -1;
469 template <
typename query_t,
typename ...pack_t>
471 seqan3::pack_traits::detail::find<query_t, pack_t...>();
474 template <
template <
typename>
typename pred_t, seqan3::detail::type_list_specialisation list_t>
475 inline constexpr ptrdiff_t
find_if = -1;
484 template <
template <
typename>
typename pred_t,
typename ...pack_t>
486 seqan3::pack_traits::detail::find_if<pred_t, pack_t...>();
494 template <
typename query_t, seqan3::detail::type_list_specialisation list_t>
495 inline constexpr
bool contains = (find<query_t, list_t> != -1);
519 template <ptrdiff_t
idx, seqan3::detail::type_list_specialisation list_t>
521 requires (idx >= 0 && idx < size<list_t>) || (-idx <= size<list_t>)
523 using at =
typename decltype(detail::at<idx>(list_t{}))::type;
538 template <seqan3::detail::type_list_specialisation list_t>
540 requires (size<list_t> > 0)
542 using
front = typename decltype(detail::
front(list_t{}))::type;
560 template <seqan3::detail::type_list_specialisation list_t>
562 requires (size<list_t> > 0)
564 using
back = typename decltype(detail::
back(list_t{}))::type;
588 template <
typename ...lists_t>
590 requires (seqan3::detail::type_list_specialisation<lists_t> && ...)
607 template <seqan3::detail::type_list_specialisation list_t>
609 requires (size<list_t> > 0)
627 template <ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
629 requires (i >= 0 && i <= size<list_t>)
647 template <ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
649 requires (i >= 0 && i <= size<list_t>)
667 template <ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
669 requires (i >= 0 && i <= size<list_t>)
687 template <ptrdiff_t i,
seqan3::detail::type_list_specialisation list_t>
689 requires (i >= 0 && i <=
size<list_t>)
707 template <ptrdiff_t i,
seqan3::detail::type_list_specialisation list_t>
709 requires (i >= 0 && i <=
size<list_t>)
730 template <
template <
typename>
typename trait_t, seqan3::detail::type_list_specialisation list_t>
731 using transform = decltype(detail::transform<trait_t>(list_t{}));
748 template <
typename replace_t, std::ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
750 requires (i >= 0 && i < size<list_t>)
783 template <ptrdiff_t i,
typename ...pack_t>
785 requires (i >= 0 && i <=
sizeof...(pack_t))
803 template <ptrdiff_t i, typename ...pack_t>
805 requires (i >= 0 && i <= sizeof...(pack_t))
823 template <ptrdiff_t i, typename ...pack_t>
825 requires (i >= 0 && i <= sizeof...(pack_t))
843 template <ptrdiff_t i, typename ...pack_t>
845 requires (i >= 0 && i <= sizeof...(pack_t))
863 template <ptrdiff_t i, typename ...pack_t>
865 requires (i >= 0 && i <= sizeof...(pack_t))
884 template <typename replace_t,
std::ptrdiff_t i, typename ...pack_t>
886 requires (i >= 0 && i < sizeof...(pack_t))
decltype(detail::transform< trait_t >(list_t{})) transform
Apply a transformation trait to every type in the list and return a seqan3::type_list of the results.
Definition: traits.hpp:731
decltype(seqan3::list_traits::replace_at< replace_t, i, type_list< pack_t... > >()) replace_at
Replace the type at the given index with the given type.
Definition: traits.hpp:888
seqan3::list_traits::take_last< i, type_list< pack_t... > > take_last
Return a seqan3::type_list of the last n types in the type pack.
Definition: traits.hpp:827
seqan3::list_traits::split_after< i, type_list< pack_t... > > split_after
Split a type pack into two parts returned as a pair of seqan3::type_list.
Definition: traits.hpp:867
decltype(detail::replace_at< replace_t >(detail::split_after< i >(type_list<>{}, list_t{}))) replace_at
Replace the type at the given index with the given type.
Definition: traits.hpp:752
meta::list< types... > type_list
Type that contains multiple types, an alias for meta::list.
Definition: type_list.hpp:31
constexpr ptrdiff_t find
Get the index of the first occurrence of a type in a pack.
Definition: traits.hpp:152
Provides various type traits on generic types.
typename decltype(detail::back(list_t{}))::type back
Return the last type from the type list.
Definition: traits.hpp:564
decltype(detail::drop_front(list_t{})) drop_front
Return a seqan3::type_list of all the types in the type list, except the first.
Definition: traits.hpp:611
typename decltype(detail::split_after< i >(type_list<>{}, list_t{}))::first_type take
Return a seqan3::type_list of the first n types in the input type list.
Definition: traits.hpp:631
decltype(detail::split_after< i >(type_list<>{}, list_t{})) split_after
Split a seqan3::type_list into two parts returned as a pair of seqan3::type_list.
Definition: traits.hpp:711
Namespace containing traits for working on seqan3::type_list.
take< size< list_t > - i, list_t > drop_last
Return a seqan3::type_list of the types the input type list, except the last n.
Definition: traits.hpp:691
constexpr bool contains
Whether a type occurs in a pack or not.
Definition: traits.hpp:193
#define SEQAN3_IS_SAME(...)
A macro that behaves like std::is_same_v, except that it doesn't need to instantiate the template on ...
Definition: basic.hpp:157
typename decltype(detail::split_after< i >(type_list<>{}, list_t{}))::second_type drop
Return a seqan3::type_list of the types in the input type list, except the first n.
Definition: traits.hpp:651
drop< size< list_t > - i, list_t > take_last
Return a seqan3::type_list of the last n types in the input type list.
Definition: traits.hpp:671
typename decltype(detail::drop_front< pack_t... >())::type drop_front
Return a seqan3::type_list of all the types in the type pack, except the first.
Definition: traits.hpp:287
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
constexpr ptrdiff_t find_if
Get the index of the first type in a pack that satisfies the given predicate.
Definition: traits.hpp:175
typename decltype(detail::front(list_t{}))::type front
Return the first type from the type list.
Definition: traits.hpp:542
seqan3::list_traits::drop_last< i, type_list< pack_t... > > drop_last
Return a seqan3::type_list of the types the type pack, except the last n.
Definition: traits.hpp:847
typename decltype(detail::at< idx >(list_t{}))::type at
Return the type at given index from the type list.
Definition: traits.hpp:523
typename decltype(detail::front< pack_t... >())::type front
Return the first type from the type pack.
Definition: traits.hpp:240
seqan3::list_traits::drop< i, type_list< pack_t... > > drop
Return a seqan3::type_list of the types in the type pack, except the first n.
Definition: traits.hpp:807
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
typename decltype((std::type_identity< pack_t >{},...))::type back
Return the last type from the type pack.
Definition: traits.hpp:262
The identity transformation (a transformation_trait that returns the input).
Definition: type_traits:31
SeqAn specific customisations in the standard namespace.
seqan3::list_traits::take< i, type_list< pack_t... > > take
Return a seqan3::type_list of the first n types in the type pack.
Definition: traits.hpp:787
decltype(detail::concat(lists_t{}...)) concat
Join two seqan3::type_list s into one.
Definition: traits.hpp:592
seqan3::type_list< trait_t< pack_t >... > transform
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
Definition: traits.hpp:307
typename decltype(detail::at< idx, pack_t... >())::type at
Return the type at given index from the type pack.
Definition: traits.hpp:221
Namespace containing traits for working on type packs.
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134
Provides seqan3::type_list.