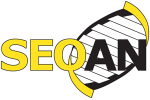 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
36 template <arithmetic score_type>
37 struct gap_score : detail::strong_type<score_type, gap_score<score_type>, detail::strong_type_skill::convert>
47 template <arithmetic score_type>
63 template <arithmetic score_type>
64 struct gap_open_score : detail::strong_type<score_type, gap_open_score<score_type>, detail::strong_type_skill::convert>
74 template <arithmetic score_type>
88 template <arithmetic score_t =
int8_t>
122 template <arithmetic score_arg_t>
146 template <arithmetic score_arg_t>
154 throw std::invalid_argument{
"You passed a score value to set_affine/set_linear that is out of range of the "
155 "scoring scheme's underlying type. Define your scoring scheme with a larger "
156 "template parameter or down-cast your score value beforehand to prevent "
160 gap =
static_cast<score_arg_t
>(g);
161 gap_open =
static_cast<score_arg_t
>(go);
174 template <arithmetic score_arg_t>
214 constexpr ptrdiff_t
score(
size_t const number_of_consecutive_gaps)
const noexcept
216 return (gap_open * (number_of_consecutive_gaps ? 1 : 0)) + number_of_consecutive_gaps *
gap;
224 constexpr
bool operator==(
gap_scheme const & rhs)
const noexcept
233 return !(*
this == rhs);
244 template <cereal_archive archive_t>
245 void CEREAL_SERIALIZE_FUNCTION_NAME(archive_t & archive)
256 score_t gap_open = 0;
265 #pragma GCC diagnostic push
266 #pragma GCC diagnostic ignored "-Wdeprecated-declarations"
275 template <
floating_po
int score_arg_type>
282 template <
floating_po
int score_arg_type>
289 template <arithmetic score_arg_type>
296 template <arithmetic score_arg_type>
300 #pragma GCC diagnostic pop
constexpr ptrdiff_t score(size_t const number_of_consecutive_gaps) const noexcept
Compute the score of a stretch of gap characters.
Definition: gap_scheme.hpp:214
The alphabet of a gap character '-'.
Definition: gap.hpp:37
constexpr score_t get_gap_score() const noexcept
A strong type of underlying type score_type that represents the score of any character against a gap ...
Definition: gap_scheme.hpp:192
constexpr bool operator!=(gap_scheme const &rhs) const noexcept
Checks whether *this is not equal to rhs.
Definition: gap_scheme.hpp:231
constexpr void set_linear(gap_score< score_arg_t > const g)
Set the Linear gap costs model.
Definition: gap_scheme.hpp:175
constexpr gap_scheme(gap_score< score_arg_t > const g)
Constructor for the Linear gap costs model (delegates to set_linear()).
Definition: gap_scheme.hpp:123
A scheme for representing and computing scores against gap characters.
Definition: gap_scheme.hpp:90
Provides basic data structure for strong types.
constexpr score_t get_gap_open_score() const noexcept
A strong type of underlying type score_type that represents an additional score (usually negative) th...
Definition: gap_scheme.hpp:205
A strong type of underlying type score_type that represents an additional score (usually negative) th...
Definition: gap_scheme.hpp:65
gap_scheme(gap_score< score_arg_type >, gap_open_score< score_arg_type >) -> gap_scheme< int8_t >
Attention: This guide does not actually deduce from the underlying type, but always defaults to int8_...
gap_score(score_type) -> gap_score< score_type >
Deduce the score type from the given argument.
A strong type of underlying type score_type that represents the score of any character against a gap ...
Definition: gap_scheme.hpp:38
gap_scheme() -> gap_scheme< int8_t >
Default constructed objects deduce to int8_t.
gap_scheme(gap_score< score_arg_type >, gap_open_score< score_arg_type >) -> gap_scheme< float >
Attention: This guide does not actually deduce from the underlying type, but always defaults to float...
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
gap_scheme(gap_score< score_arg_type >) -> gap_scheme< float >
Attention: This guide does not actually deduce from the underlying type, but always defaults to float...
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
constexpr score_t & get_gap_open_score() noexcept
Return the gap open score.
Definition: gap_scheme.hpp:199
gap_open_score(score_type) -> gap_open_score< score_type >
Deduce the score type from the given argument.
constexpr gap_scheme() noexcept=default
Defaulted.
Adaptions of concepts from the Cereal library.
A type that satisfies std::is_arithmetic_v<t>.
score_t score_type
The template parameter exposed as member type.
Definition: gap_scheme.hpp:97
constexpr score_t & get_gap_score() noexcept
Return the gap score.
Definition: gap_scheme.hpp:186
auto const convert
A view that converts each element in the input range (implicitly or via static_cast).
Definition: convert.hpp:71
constexpr void set_affine(gap_score< score_arg_t > const g, gap_open_score< score_arg_t > const go)
Set the Affine gap costs model.
Definition: gap_scheme.hpp:147
gap_scheme(gap_score< score_arg_type >) -> gap_scheme< int8_t >
Attention: This guide does not actually deduce from the underlying type, but always defaults to int8_...