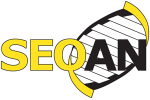 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
24 namespace seqan3::detail
35 char constexpr sam_tag_type_char[11] = {
'A',
'i',
'f',
'Z',
'B',
'B',
'B',
'B',
'B',
'B',
'B'};
37 char constexpr sam_tag_type_char_extra[11] = {
'\0',
'\0',
'\0',
'\0',
'c',
'C',
's',
'S',
'i',
'I',
'f'};
63 #ifdef __cpp_nontype_template_parameter_class
64 template <small_
string<2> str>
65 constexpr uint16_t
operator""_tag()
67 #else // GCC/Clang extension
68 #pragma GCC diagnostic push
69 #pragma GCC diagnostic ignored "-Wpedantic"
70 template <
typename char_t, char_t ...s>
71 constexpr uint16_t
operator""_tag()
73 static_assert(std::same_as<char_t, char>,
"Illegal SAM tag: Type must be char.");
75 #pragma GCC diagnostic pop
78 static_assert(str.size() == 2,
"Illegal SAM tag: Exactly two characters must be given.");
80 char constexpr char0 = str[0];
81 char constexpr char1 = str[1];
84 "Illegal SAM tag: a SAM tag must match /[A-Za-z][A-Za-z0-9]/.");
86 return static_cast<uint16_t
>(char0) * 256 +
static_cast<uint16_t
>(char1);
165 template <u
int16_t tag_value>
174 template <u
int16_t tag_value>
179 template <>
struct sam_tag_type<"AS"_tag> {
using type = int32_t; };
180 template <>
struct sam_tag_type<"BC"_tag> {
using type =
std::string; };
181 template <>
struct sam_tag_type<"BQ"_tag> {
using type =
std::string; };
182 template <>
struct sam_tag_type<"BZ"_tag> {
using type =
std::string; };
183 template <>
struct sam_tag_type<"CB"_tag> {
using type =
std::string; };
184 template <>
struct sam_tag_type<"CC"_tag> {
using type =
std::string; };
186 template <>
struct sam_tag_type<"CM"_tag> {
using type = int32_t; };
187 template <>
struct sam_tag_type<"CO"_tag> {
using type =
std::string; };
188 template <>
struct sam_tag_type<"CP"_tag> {
using type = int32_t; };
189 template <>
struct sam_tag_type<"CQ"_tag> {
using type =
std::string; };
190 template <>
struct sam_tag_type<"CR"_tag> {
using type =
std::string; };
191 template <>
struct sam_tag_type<"CS"_tag> {
using type =
std::string; };
192 template <>
struct sam_tag_type<"CT"_tag> {
using type =
std::string; };
193 template <>
struct sam_tag_type<"CY"_tag> {
using type =
std::string; };
194 template <>
struct sam_tag_type<"E2"_tag> {
using type =
std::string; };
195 template <>
struct sam_tag_type<"FI"_tag> {
using type = int32_t; };
196 template <>
struct sam_tag_type<"FS"_tag> {
using type =
std::string; };
203 template <>
struct sam_tag_type<"H0"_tag> {
using type = int32_t; };
204 template <>
struct sam_tag_type<"H1"_tag> {
using type = int32_t; };
205 template <>
struct sam_tag_type<"H2"_tag> {
using type = int32_t; };
206 template <>
struct sam_tag_type<"HI"_tag> {
using type = int32_t; };
207 template <>
struct sam_tag_type<"IH"_tag> {
using type = int32_t; };
208 template <>
struct sam_tag_type<"LB"_tag> {
using type =
std::string; };
209 template <>
struct sam_tag_type<"MC"_tag> {
using type =
std::string; };
210 template <>
struct sam_tag_type<"MD"_tag> {
using type =
std::string; };
214 template <>
struct sam_tag_type<"MI"_tag> {
using type =
std::string; };
215 template <>
struct sam_tag_type<"MQ"_tag> {
using type = int32_t; };
216 template <>
struct sam_tag_type<"NH"_tag> {
using type = int32_t; };
217 template <>
struct sam_tag_type<"NM"_tag> {
using type = int32_t; };
218 template <>
struct sam_tag_type<"OC"_tag> {
using type =
std::string; };
219 template <>
struct sam_tag_type<"OP"_tag> {
using type = int32_t; };
220 template <>
struct sam_tag_type<"OQ"_tag> {
using type =
std::string; };
221 template <>
struct sam_tag_type<"OX"_tag> {
using type =
std::string; };
222 template <>
struct sam_tag_type<"PG"_tag> {
using type =
std::string; };
223 template <>
struct sam_tag_type<"PQ"_tag> {
using type = int32_t; };
224 template <>
struct sam_tag_type<"PT"_tag> {
using type =
std::string; };
225 template <>
struct sam_tag_type<"PU"_tag> {
using type =
std::string; };
226 template <>
struct sam_tag_type<"Q2"_tag> {
using type =
std::string; };
227 template <>
struct sam_tag_type<"QT"_tag> {
using type =
std::string; };
228 template <>
struct sam_tag_type<"QX"_tag> {
using type =
std::string; };
229 template <>
struct sam_tag_type<"R2"_tag> {
using type =
std::string; };
230 template <>
struct sam_tag_type<"RG"_tag> {
using type =
std::string; };
231 template <>
struct sam_tag_type<"RT"_tag> {
using type =
std::string; };
232 template <>
struct sam_tag_type<"RX"_tag> {
using type =
std::string; };
236 template <>
struct sam_tag_type<"SA"_tag> {
using type =
std::string; };
237 template <>
struct sam_tag_type<"SM"_tag> {
using type = int32_t; };
241 template <>
struct sam_tag_type<"TC"_tag> {
using type = int32_t; };
242 template <>
struct sam_tag_type<"U2"_tag> {
using type =
std::string; };
243 template <>
struct sam_tag_type<"UQ"_tag> {
using type = int32_t; };
349 template <u
int16_t tag>
356 if ((*this).count(tag) == 0)
359 return std::get<sam_tag_type_t<tag>>((*this)[tag]);
363 template <u
int16_t tag>
369 if ((*this).count(tag) == 0)
372 return std::get<sam_tag_type_t<tag>>(
std::move((*
this)[tag]));
377 template <u
int16_t tag>
381 auto const &
get() const &
383 return std::get<sam_tag_type_t<tag>>((*this).at(tag));
388 template <u
int16_t tag>
392 auto const &&
get() const &&
394 return std::get<sam_tag_type_t<tag>>(
std::move((*this).at(tag)));
constexpr auto is_alnum
Checks whether c is a alphanumeric character.
Definition: predicate.hpp:220
constexpr auto is_alpha
Checks whether c is a alphabetical character.
Definition: predicate.hpp:239
Implements a small string that can be used for compile time computations.
Definition: small_string.hpp:43
Provides seqan3::type_list and auxiliary type traits.
detail::sam_tag_variant variant_type
The variant type defining all valid SAM tag field types.
Definition: sam_tag_dictionary.hpp:332
typename sam_tag_type< tag_value >::type sam_tag_type_t
Short cut helper for seqan3::sam_tag_type::type.
Definition: sam_tag_dictionary.hpp:175
The generic base class.
Definition: sam_tag_dictionary.hpp:167
detail::sam_tag_variant type
The type for all unknown tags with no extra overload defaults to an std::variant.
Definition: sam_tag_dictionary.hpp:169
auto & get() &
Uses std::map::operator[] for access and default initializes new keys.
Definition: sam_tag_dictionary.hpp:354
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
A constexpr string implementation to manipulate string literals at compile time.
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
The SAM tag dictionary class that stores all optional SAM fields.
Definition: sam_tag_dictionary.hpp:325
Provides character predicates for tokenisation.