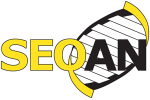 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
23 #if SEQAN3_WITH_CEREAL
24 #include <cereal/types/array.hpp>
25 #endif // SEQAN3_WITH_CEREAL
39 template <arithmetic score_type>
40 struct match_score : detail::strong_type<score_type, match_score<score_type>, detail::strong_type_skill::convert>
50 template <arithmetic score_type>
64 template <arithmetic score_type>
65 struct mismatch_score : detail::strong_type<score_type, mismatch_score<score_type>, detail::strong_type_skill::convert>
75 template <arithmetic score_type>
98 template <
typename derived_t, alphabet alphabet_t, arithmetic score_t>
146 template <arithmetic score_arg_t>
177 template <arithmetic score_arg_t>
185 throw std::invalid_argument{
"You passed a score value to set_simple_scheme that is out of range of the "
186 "scoring scheme's underlying type. Define your scoring scheme with a larger "
187 "template parameter or down-cast you score value beforehand to prevent "
193 matrix[i][j] = (i == j) ?
static_cast<score_t
>(i_ms) :
static_cast<score_t
>(i_mms);
201 std::ranges::copy(matrix, this->matrix.
begin());
215 template <
typename alph1_t,
typename alph2_t>
219 constexpr score_t &
score(alph1_t
const alph1, alph2_t
const alph2) noexcept
221 return matrix[
to_rank(
static_cast<alphabet_t
>(alph1))][
to_rank(
static_cast<alphabet_t
>(alph2))];
225 template <
typename alph1_t,
typename alph2_t>
229 constexpr score_t
score(alph1_t
const alph1, alph2_t
const alph2)
const noexcept
231 return matrix[
to_rank(
static_cast<alphabet_t
>(alph1))][
to_rank(
static_cast<alphabet_t
>(alph2))];
239 constexpr
bool operator==(derived_t
const & rhs)
const noexcept
241 return matrix == rhs.matrix;
245 constexpr
bool operator!=(derived_t
const & rhs)
const noexcept
247 return matrix != rhs.matrix;
258 template <cereal_archive archive_t>
259 void CEREAL_SERIALIZE_FUNCTION_NAME(archive_t & archive)
static constexpr matrix_size_type matrix_size
Size of the matrix dimensions (i.e. size of the alphabet).
Definition: scoring_scheme_base.hpp:114
constexpr bool operator!=(derived_t const &rhs) const noexcept
Checks whether *this is not equal to rhs.
Definition: scoring_scheme_base.hpp:245
constexpr void set_hamming_distance() noexcept
Set the hamming scheme, a variant of the simple scheme where match is scored 0 and mismatch -1.
Definition: scoring_scheme_base.hpp:166
constexpr score_t score(alph1_t const alph1, alph2_t const alph2) const noexcept
Score two letters (either two nucleotids or two amino acids).
Definition: scoring_scheme_base.hpp:229
constexpr scoring_scheme_base(scoring_scheme_base const &) noexcept=default
Defaulted.
A CRTP base class for scoring schemes.
Definition: scoring_scheme_base.hpp:100
Resolves to std::ranges::explicitly_convertible_to<type1, type2>().
Provides basic data structure for strong types.
constexpr void set_custom_matrix(matrix_type const &matrix) noexcept
Set a custom scheme by passing a full matrix with arbitrary content.
Definition: scoring_scheme_base.hpp:199
constexpr auto to_rank
Return the rank representation of a (semi-)alphabet object.
Definition: concept.hpp:143
constexpr score_t & score(alph1_t const alph1, alph2_t const alph2) noexcept
Score two letters (either two nucleotids or two amino acids).
Definition: scoring_scheme_base.hpp:219
Adaptations of algorithms from the Ranges TS.
mismatch_score(score_type) -> mismatch_score< score_type >
Deduce the score type from the provided argument.
A strong type of underlying type score_type that represents the score two different characters.
Definition: scoring_scheme_base.hpp:66
constexpr scoring_scheme_base(match_score< score_arg_t > const ms, mismatch_score< score_arg_t > const mms)
Constructor for the simple scheme (delegates to set_simple_scheme()).
Definition: scoring_scheme_base.hpp:147
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
score_t score_type
Type of the score values.
Definition: scoring_scheme_base.hpp:106
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
alphabet_t alphabet_type
Type of the underlying alphabet.
Definition: scoring_scheme_base.hpp:108
constexpr scoring_scheme_base(matrix_type const &matrix) noexcept
Constructor for a custom scheme (delegates to set_custom_matrix()).
Definition: scoring_scheme_base.hpp:155
constexpr scoring_scheme_base(scoring_scheme_base &&) noexcept=default
Defaulted.
Adaptions of concepts from the Cereal library.
constexpr bool operator==(derived_t const &rhs) const noexcept
Checks whether *this is equal to rhs.
Definition: scoring_scheme_base.hpp:239
std::array< std::array< score_type, matrix_size >, matrix_size > matrix_type
Type of the internal matrix (a two-dimensional array).
Definition: scoring_scheme_base.hpp:120
match_score(score_type) -> match_score< score_type >
Deduce the score type from the provided argument.
A strong type of underlying type score_type that represents the score of two matching characters.
Definition: scoring_scheme_base.hpp:41
auto const convert
A view that converts each element in the input range (implicitly or via static_cast).
Definition: convert.hpp:71
constexpr void set_simple_scheme(match_score< score_arg_t > const ms, mismatch_score< score_arg_t > const mms)
Set the simple scheme (everything is either match or mismatch).
Definition: scoring_scheme_base.hpp:178
Core alphabet concept and free function/type trait wrappers.