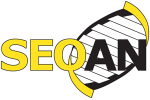 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
37 #include <seqan3/io/detail/record.hpp>
111 template <
typename t>
131 requires std::same_as<typename t::ref_sequences, ref_info_not_given> || requires ()
138 (!std::same_as<typename t::ref_sequences, ref_info_not_given> ||
140 requires std::ranges::forward_range<std::ranges::range_reference_t<typename t::ref_ids>>;
141 requires std::ranges::forward_range<typename t::ref_ids>;
182 template <
typename ref_sequences_t = ref_info_not_given,
typename ref_
ids_t = std::deque<std::
string>>
197 template <
typename _sequence_alphabet>
201 template <
typename _
id_alphabet>
208 template <
typename _quality_alphabet>
371 using dummy_ref_type = decltype(
views::repeat_n(
typename traits_type::sequence_alphabet{},
size_t{}) |
375 using ref_sequence_unsliced_type =
376 detail::lazy_conditional_t<std::ranges::range<typename traits_type::ref_sequences const>,
377 detail::lazy<std::ranges::range_reference_t,
378 typename traits_type::ref_sequences
const>,
382 using ref_sequence_sliced_type = decltype(std::declval<ref_sequence_unsliced_type>() |
views::slice(0, 0));
391 typename traits_type::sequence_alphabet>;
393 using id_type =
typename traits_type::template id_container<char>;
404 ref_sequence_sliced_type>;
424 typename traits_type::quality_alphabet>;
443 decltype(std::declval<sequence_type &>() |
views::slice(0, 0))>,
510 static_assert([] () constexpr
512 for (
field f : selected_field_ids::as_array)
513 if (!field_ids::contains(f))
517 "You selected a field that is not valid for aligment files, please refer to the documentation "
518 "of alignment_file_input::field_ids for the accepted values.");
540 using iterator = detail::in_file_iterator<alignment_file_input>;
606 template <input_stream stream_t, alignment_file_input_format file_format>
608 requires std::same_as<typename std::remove_reference_t<stream_t>::char_type,
stream_char_type>
611 file_format
const & SEQAN3_DOXYGEN_ONLY(format_tag),
613 primary_stream{&stream, stream_deleter_noop}
615 init_by_format<file_format>();
619 template <input_stream stream_t, alignment_file_input_format file_format>
621 requires std::same_as<typename std::remove_reference_t<stream_t>::char_type,
stream_char_type>
624 file_format
const & SEQAN3_DOXYGEN_ONLY(format_tag),
626 primary_stream{
new stream_t{
std::move(stream)}, stream_deleter_default}
628 init_by_format<file_format>();
655 typename traits_type::ref_ids & ref_ids,
656 typename traits_type::ref_sequences & ref_sequences,
661 set_references(ref_ids, ref_sequences);
691 template <input_stream stream_t, alignment_file_input_format file_format>
693 typename traits_type::ref_ids & ref_ids,
694 typename traits_type::ref_sequences & ref_sequences,
695 file_format
const & SEQAN3_DOXYGEN_ONLY(format_tag),
697 primary_stream{&stream, stream_deleter_noop}
700 set_references(ref_ids, ref_sequences);
702 init_by_format<file_format>();
706 template <input_stream stream_t, alignment_file_input_format file_format>
708 typename traits_type::ref_ids & ref_ids,
709 typename traits_type::ref_sequences & ref_sequences,
710 file_format
const & SEQAN3_DOXYGEN_ONLY(format_tag),
712 primary_stream{
new stream_t{
std::move(stream)}, stream_deleter_default}
715 set_references(ref_ids, ref_sequences);
717 init_by_format<file_format>();
723 typename traits_type::ref_ids &&,
724 typename traits_type::ref_sequences &&,
727 template <input_stream stream_t, alignment_file_input_format file_format>
729 typename traits_type::ref_ids &&,
730 typename traits_type::ref_sequences &&,
759 if (!first_record_was_read)
762 first_record_was_read =
true;
833 if (!first_record_was_read)
836 first_record_was_read =
true;
848 primary_stream->rdbuf()->pubsetbuf(stream_buffer.
data(), stream_buffer.
size());
850 std::ios_base::in | std::ios::binary);
852 if (!primary_stream->good())
855 secondary_stream = detail::make_secondary_istream(*primary_stream, filename);
856 detail::set_format(format, filename);
860 template <
typename format_type>
861 void init_by_format()
863 static_assert(list_traits::contains<format_type, valid_formats>,
864 "You selected a format that is not in the valid_formats of this file.");
866 format = detail::alignment_file_input_format_exposer<format_type>{};
867 secondary_stream = detail::make_secondary_istream(*primary_stream);
894 stream_ptr_t primary_stream{
nullptr, stream_deleter_noop};
896 stream_ptr_t secondary_stream{
nullptr, stream_deleter_noop};
899 bool first_record_was_read{
false};
904 using format_type =
typename detail::variant_from_tags<
valid_formats,
905 detail::alignment_file_input_format_exposer>::type;
914 typename traits_type::ref_sequences
const * reference_sequences_ptr{
nullptr};
927 template <std::ranges::forward_range ref_sequences_t>
928 void set_references(
typename traits_type::ref_ids & ref_ids, ref_sequences_t && ref_sequences)
930 assert(std::ranges::distance(ref_ids) == std::ranges::distance(ref_sequences));
933 reference_sequences_ptr = &ref_sequences;
936 for (int32_t idx = 0; idx < std::ranges::distance(ref_ids); ++idx)
938 header_ptr->ref_id_info.emplace_back(std::ranges::distance(ref_sequences[idx]),
"");
940 if constexpr (std::ranges::contiguous_range<std::ranges::range_reference_t<
941 typename traits_type::ref_ids>> &&
942 std::ranges::sized_range<std::ranges::range_reference_t<typename traits_type::ref_ids>> &&
943 std::ranges::borrowed_range<std::ranges::range_reference_t<typename traits_type::ref_ids>>)
945 auto &&
id = header_ptr->ref_ids()[idx];
950 header_ptr->ref_dict[header_ptr->ref_ids()[idx]] = idx;
957 void read_next_record()
960 record_buffer.clear();
961 detail::get_or_ignore<field::header_ptr>(record_buffer) = header_ptr.get();
971 auto call_read_func = [
this] (
auto & ref_seq_info)
975 f.read_alignment_record(*secondary_stream,
979 detail::get_or_ignore<field::seq>(record_buffer),
980 detail::get_or_ignore<field::qual>(record_buffer),
981 detail::get_or_ignore<field::id>(record_buffer),
982 detail::get_or_ignore<field::offset>(record_buffer),
983 detail::get_or_ignore<field::ref_seq>(record_buffer),
984 detail::get_or_ignore<field::ref_id>(record_buffer),
985 detail::get_or_ignore<field::ref_offset>(record_buffer),
986 detail::get_or_ignore<field::alignment>(record_buffer),
987 detail::get_or_ignore<field::cigar>(record_buffer),
988 detail::get_or_ignore<field::flag>(record_buffer),
989 detail::get_or_ignore<field::mapq>(record_buffer),
990 detail::get_or_ignore<field::mate>(record_buffer),
991 detail::get_or_ignore<field::tags>(record_buffer),
992 detail::get_or_ignore<field::evalue>(record_buffer),
993 detail::get_or_ignore<field::bit_score>(record_buffer));
997 assert(!
format.valueless_by_exception());
999 if constexpr (!std::same_as<typename traits_type::ref_sequences, ref_info_not_given>)
1000 call_read_func(*reference_sequences_ptr);
1002 call_read_func(std::ignore);
1013 template <input_stream stream_type,
1016 detail::fields_specialisation selected_field_ids>
1018 file_format
const &,
1025 template <input_stream stream_type,
1029 file_format
const &,
1036 template <input_stream stream_type,
1039 file_format
const &)
1045 template <input_stream stream_type,
1048 file_format
const &)
1054 template <std::ranges::forward_range ref_ids_t,
1055 std::ranges::forward_range ref_sequences_t,
1067 template <std::ranges::forward_range ref_ids_t,
1068 std::ranges::forward_range ref_sequences_t>
1078 template <input_stream stream_type,
1079 std::ranges::forward_range ref_ids_t,
1080 std::ranges::forward_range ref_sequences_t,
1086 file_format
const &,
1094 template <input_stream stream_type,
1095 std::ranges::forward_range ref_ids_t,
1096 std::ranges::forward_range ref_sequences_t,
1102 file_format
const &,
1110 template <input_stream stream_type,
1111 std::ranges::forward_range ref_ids_t,
1112 std::ranges::forward_range ref_sequences_t,
1117 file_format
const &)
1124 template <input_stream stream_type,
1125 std::ranges::forward_range ref_ids_t,
1126 std::ranges::forward_range ref_sequences_t,
1131 file_format
const &)
1153 seqan3::detail::fields_specialisation selected_field_ids,
1154 seqan3::detail::type_list_of_alignment_file_input_formats valid_formats>
1155 struct tuple_size<
seqan3::alignment_file_input<traits_type, selected_field_ids, valid_formats>>
1158 static constexpr
size_t value = selected_field_ids::as_array.size();
1166 template <
size_t elem_no,
1168 seqan3::detail::fields_specialisation selected_field_ids,
1169 seqan3::detail::type_list_of_alignment_file_input_formats valid_formats>
1170 struct tuple_element<elem_no,
seqan3::alignment_file_input<traits_type, selected_field_ids, valid_formats>>
1171 : tuple_element<elem_no, typename seqan3::alignment_file_input<traits_type,
1173 valid_formats>::file_as_tuple_type>
Provides helper data structures for the seqan3::alignment_file_output.
Provides quality alphabet composites.
A more refined container concept than seqan3::container.
meta::list< types... > type_list
Type that contains multiple types, an alias for meta::list.
Definition: type_list.hpp:31
Provides seqan3::dna15, container aliases and string literals.
Provides seqan3::tuple_like.
@ offset
Sequence (SEQ) relative start position (0-based), unsigned value.
Provides seqan3::gap_decorator.
Resolves to std::ranges::explicitly_convertible_to<type1, type2>().
@ id
The identifier, usually a string.
The 15 letter DNA alphabet, containing all IUPAC smybols minus the gap.
Definition: dna15.hpp:49
sam_flag
An enum flag that describes the properties of an aligned read (given as a SAM record).
Definition: misc.hpp:71
Provides the seqan3::record template and the seqan3::field enum.
This header includes C++17 filesystem support and imports it into namespace std::filesystem (independ...
constexpr bool contains
Whether a type occurs in a pack or not.
Definition: traits.hpp:193
A class template that holds a choice of seqan3::field.
Definition: record.hpp:166
constexpr sequenced_policy seq
Global execution policy object for sequenced execution policy.
Definition: execution.hpp:54
A combined alphabet that can hold values of either of its alternatives.
Definition: alphabet_variant.hpp:133
Provides seqan3::views::slice.
Provides seqan3::views::repeat_n.
Provides seqan3::aa27, container aliases and string literals.
@ cigar
The cigar vector (std::vector<seqan3::cigar>) representing the alignment in SAM/BAM format.
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
@ mapq
The mapping quality of the SEQ alignment, usually a ohred-scaled score.
Provides exceptions used in the I/O module.
Provides seqan3::dna5, container aliases and string literals.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
Quality type for traditional Sanger and modern Illumina Phred scores (typical range).
Definition: phred42.hpp:44
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
Provides alphabet adaptations for standard char types.
Adaptations of concepts from the Ranges TS.
The SAM tag dictionary class that stores all optional SAM fields.
Definition: sam_tag_dictionary.hpp:325
The generic alphabet concept that covers most data types used in ranges.
SeqAn specific customisations in the standard namespace.
Provides the seqan3::cigar alphabet.
field
An enumerator for the fields used in file formats.
Definition: record.hpp:65
constexpr auto slice
A view adaptor that returns a half-open interval on the underlying range.
Definition: slice.hpp:141
constexpr auto repeat_n
A view factory that repeats a given value n times.
Definition: repeat_n.hpp:94
A gap decorator allows the annotation of sequences with gap symbols while leaving the underlying sequ...
Definition: gap_decorator.hpp:85
The five letter DNA alphabet of A,C,G,T and the unknown character N.
Definition: dna5.hpp:49
seqan3::type_list< trait_t< pack_t >... > transform
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
Definition: traits.hpp:307
Provides the seqan3::detail::in_file_iterator class template.
@ flag
The alignment flag (bit information), uint16_t value.
Thrown if there is an unspecified filesystem or stream error while opening, e.g. permission problem.
Definition: exception.hpp:40
Provides traits for seqan3::type_list.
Refines seqan3::alphabet and adds assignability.
A concept that indicates whether a writable alphabet represents quality scores.
Provides seqan3::phred42 quality scores.
@ header_ptr
A pointer to the seqan3::alignment_file_header object storing header information.
@ alignment
The (pairwise) alignment stored in an seqan3::alignment object.