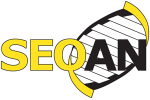 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Provides seqan3::type_list and metaprogramming utilities for working on type lists and type packs.
More...
|
template<typename ... types> |
using | seqan3::type_list = meta::list< types... > |
| Type that contains multiple types, an alias for meta::list.
|
|
|
template<typename ... pack_t> |
constexpr size_t | seqan3::pack_traits::size = sizeof...(pack_t) |
| The size of a type pack. More...
|
|
template<typename query_t , typename ... pack_t> |
constexpr ptrdiff_t | seqan3::pack_traits::count = (SEQAN3_IS_SAME(query_t, pack_t) + ... + 0) |
| Count the occurrences of a type in a pack. More...
|
|
template<typename query_t , typename ... pack_t> |
constexpr ptrdiff_t | seqan3::pack_traits::find = seqan3::pack_traits::detail::find<query_t, pack_t...>() |
| Get the index of the first occurrence of a type in a pack. More...
|
|
template<template< typename > typename pred_t, typename ... pack_t> |
constexpr ptrdiff_t | seqan3::pack_traits::find_if = seqan3::pack_traits::detail::find_if<pred_t, pack_t...>() |
| Get the index of the first type in a pack that satisfies the given predicate. More...
|
|
template<typename query_t , typename ... pack_t> |
constexpr bool | seqan3::pack_traits::contains = (find<query_t, pack_t...> != -1) |
| Whether a type occurs in a pack or not. More...
|
|
|
template<typename ... pack_t> |
using | seqan3::pack_traits::drop_front = typename decltype(detail::drop_front< pack_t... >())::type |
| Return a seqan3::type_list of all the types in the type pack, except the first. More...
|
|
template<template< typename > typename trait_t, typename ... pack_t> |
using | seqan3::pack_traits::transform = seqan3::type_list< trait_t< pack_t >... > |
| Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results. More...
|
|
template<ptrdiff_t i, typename ... pack_t> |
using | seqan3::pack_traits::take = seqan3::list_traits::take< i, type_list< pack_t... > > |
| Return a seqan3::type_list of the first n types in the type pack. More...
|
|
template<ptrdiff_t i, typename ... pack_t> |
using | seqan3::pack_traits::drop = seqan3::list_traits::drop< i, type_list< pack_t... > > |
| Return a seqan3::type_list of the types in the type pack, except the first n . More...
|
|
template<ptrdiff_t i, typename ... pack_t> |
using | seqan3::pack_traits::take_last = seqan3::list_traits::take_last< i, type_list< pack_t... > > |
| Return a seqan3::type_list of the last n types in the type pack. More...
|
|
template<ptrdiff_t i, typename ... pack_t> |
using | seqan3::pack_traits::drop_last = seqan3::list_traits::drop_last< i, type_list< pack_t... > > |
| Return a seqan3::type_list of the types the type pack, except the last n . More...
|
|
template<ptrdiff_t i, typename ... pack_t> |
using | seqan3::pack_traits::split_after = seqan3::list_traits::split_after< i, type_list< pack_t... > > |
| Split a type pack into two parts returned as a pair of seqan3::type_list. More...
|
|
template<typename replace_t , std::ptrdiff_t i, typename ... pack_t> |
using | seqan3::pack_traits::replace_at = decltype(seqan3::list_traits::replace_at< replace_t, i, type_list< pack_t... > >()) |
| Replace the type at the given index with the given type. More...
|
|
|
template<ptrdiff_t idx, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::at = typename decltype(detail::at< idx >(list_t{}))::type |
| Return the type at given index from the type list. More...
|
|
template<seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::front = typename decltype(detail::front(list_t{}))::type |
| Return the first type from the type list. More...
|
|
template<seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::back = typename decltype(detail::back(list_t{}))::type |
| Return the last type from the type list. More...
|
|
|
template<typename ... lists_t> |
using | seqan3::list_traits::concat = decltype(detail::concat(lists_t{}...)) |
| Join two seqan3::type_list s into one. More...
|
|
template<seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::drop_front = decltype(detail::drop_front(list_t{})) |
| Return a seqan3::type_list of all the types in the type list, except the first. More...
|
|
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::take = typename decltype(detail::split_after< i >(type_list<>{}, list_t{}))::first_type |
| Return a seqan3::type_list of the first n types in the input type list. More...
|
|
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::drop = typename decltype(detail::split_after< i >(type_list<>{}, list_t{}))::second_type |
| Return a seqan3::type_list of the types in the input type list, except the first n . More...
|
|
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::take_last = drop< size< list_t > - i, list_t > |
| Return a seqan3::type_list of the last n types in the input type list. More...
|
|
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::drop_last = take< size< list_t > - i, list_t > |
| Return a seqan3::type_list of the types the input type list, except the last n . More...
|
|
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::split_after = decltype(detail::split_after< i >(type_list<>{}, list_t{})) |
| Split a seqan3::type_list into two parts returned as a pair of seqan3::type_list. More...
|
|
template<template< typename > typename trait_t, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::transform = decltype(detail::transform< trait_t >(list_t{})) |
| Apply a transformation trait to every type in the list and return a seqan3::type_list of the results. More...
|
|
template<typename replace_t , std::ptrdiff_t i, seqan3::detail::type_list_specialisation list_t> |
using | seqan3::list_traits::replace_at = decltype(detail::replace_at< replace_t >(detail::split_after< i >(type_list<>{}, list_t{}))) |
| Replace the type at the given index with the given type. More...
|
|
Provides seqan3::type_list and metaprogramming utilities for working on type lists and type packs.
All traits are defined in the header <seqan3/core/type_list/traits.hpp>
.
◆ at [1/2]
template<ptrdiff_t idx, typename ... pack_t>
Return the type at given index from the type pack.
- Template Parameters
-
idx | The index; must be smaller than the size of the pack. |
pack_t | The type pack. |
Negative indexes are supported (e.g. at<-1, int, double, bool &>
is bool &
).
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ at [2/2]
template<ptrdiff_t idx, seqan3::detail::type_list_specialisation list_t>
Return the type at given index from the type list.
- Template Parameters
-
idx | The index; must be smaller than the size of the type list. |
list_t | The type_list. |
Negative indexes are supported (e.g. at<-1, type_list<int, double, bool &>>
is bool &
).
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ back [1/2]
template<typename ... pack_t>
Return the last type from the type pack.
- Template Parameters
-
(Compile-time) Complexity
- Number of template instantiations: O(n) (possibly O(1))
- Other operations: O(1)
Notably faster than seqan3::pack_traits::at<size<pack...> - 1, pack...>
(no recursive template instantiations).
◆ back [2/2]
template<seqan3::detail::type_list_specialisation list_t>
Return the last type from the type list.
- Template Parameters
-
(Compile-time) Complexity
- Number of template instantiations: O(n) (possibly O(1))
- Other operations: O(1)
Notably faster than seqan3::pack_traits::at<size<pack...> - 1, pack...>
(no recursive template instantiations).
◆ concat
template<typename ... lists_t>
Join two seqan3::type_list s into one.
- Template Parameters
-
list1_t | The first (input) type list. |
list2_t | The second (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n) in the number of type lists
- Other operations: O(n) in the number of type lists
Complexity is independent of the number of types in each list.
◆ drop [1/2]
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
Return a seqan3::type_list of the types in the input type list, except the first n
.
- Template Parameters
-
i | The amount to drop; must be >= 0 and <= the size of the input type list. |
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ drop [2/2]
template<ptrdiff_t i, typename ... pack_t>
Return a seqan3::type_list of the types in the type pack, except the first n
.
- Template Parameters
-
i | The amount to drop; must be >= 0 and <= the size of the type pack. |
pack_t | The type pack. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ drop_front [1/2]
template<typename ... pack_t>
Return a seqan3::type_list of all the types in the type pack, except the first.
- Template Parameters
-
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(1)
◆ drop_front [2/2]
template<seqan3::detail::type_list_specialisation list_t>
Return a seqan3::type_list of all the types in the type list, except the first.
- Template Parameters
-
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(1)
◆ drop_last [1/2]
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
Return a seqan3::type_list of the types the input type list, except the last n
.
- Template Parameters
-
i | The amount to drop; must be >= 0 and <= the size of the input type list. |
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ drop_last [2/2]
template<ptrdiff_t i, typename ... pack_t>
Return a seqan3::type_list of the types the type pack, except the last n
.
- Template Parameters
-
i | The amount to drop; must be >= 0 and <= the size of the type pack. |
pack_t | The type pack. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ front [1/2]
template<typename ... pack_t>
Return the first type from the type pack.
- Template Parameters
-
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(1)
◆ front [2/2]
template<seqan3::detail::type_list_specialisation list_t>
Return the first type from the type list.
- Template Parameters
-
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(1)
◆ replace_at [1/2]
template<typename replace_t , std::ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
Replace the type at the given index with the given type.
- Template Parameters
-
replace_t | The type to replace the old type with. |
i | The index of the type to be replaced. |
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ replace_at [2/2]
template<typename replace_t , std::ptrdiff_t i, typename ... pack_t>
Replace the type at the given index with the given type.
- Template Parameters
-
replace_t | The type to replace the old type with. |
i | The index of the type to be replaced. |
pack_t | The (input) type pack. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ split_after [1/2]
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
Split a seqan3::type_list into two parts returned as a pair of seqan3::type_list.
- Template Parameters
-
i | The number of elements after which to split; must be >= 0 and <= the size of the input type list. |
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ split_after [2/2]
template<ptrdiff_t i, typename ... pack_t>
Split a type pack into two parts returned as a pair of seqan3::type_list.
- Template Parameters
-
i | The number of elements after which to split; must be >= 0 and <= the size of the type pack. |
pack_t | The type pack. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ take [1/2]
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
Return a seqan3::type_list of the first n
types in the input type list.
- Template Parameters
-
i | The target size; must be >= 0 and <= the size of the input type list. |
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ take [2/2]
template<ptrdiff_t i, typename ... pack_t>
Return a seqan3::type_list of the first n
types in the type pack.
- Template Parameters
-
i | The target size; must be >= 0 and <= the size of the type pack. |
pack_t | The type pack. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ take_last [1/2]
template<ptrdiff_t i, seqan3::detail::type_list_specialisation list_t>
Return a seqan3::type_list of the last n
types in the input type list.
- Template Parameters
-
i | The target size; must be >= 0 and <= the size of the input type list. |
list_t | The (input) type list. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ take_last [2/2]
template<ptrdiff_t i, typename ... pack_t>
Return a seqan3::type_list of the last n
types in the type pack.
- Template Parameters
-
i | The target size; must be >= 0 and <= the size of the type pack. |
pack_t | The type pack. |
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ transform [1/2]
template<template< typename > typename trait_t, typename ... pack_t>
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
- Template Parameters
-
trait_t | The transformation trait, can be an alias template, e.g. a transformation trait shortcut. |
pack_t | The type pack. |
The transformation trait given as first argument can be an alias template, e.g. std::type_identity_t, not std::type_identity. The alias must take exactly one argument and be defined for all types in the pack.
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(1)
◆ transform [2/2]
template<template< typename > typename trait_t, seqan3::detail::type_list_specialisation list_t>
Apply a transformation trait to every type in the list and return a seqan3::type_list of the results.
- Template Parameters
-
trait_t | The trait to transform, must be an alias template, e.g. a transformation trait shortcut. |
list_t | The (input) type list. |
The transformation trait given as first argument must be an alias template, e.g. std::type_identity_t, not std::type_identity. The alias must take exactly one argument and be defined for all types in the input list.
(Compile-time) Complexity
- Number of template instantiations: O(n)
- Other operations: O(n)
◆ contains [1/2]
template<typename query_t , typename ... pack_t>
constexpr bool seqan3::pack_traits::contains = (find<query_t, pack_t...> != -1) |
|
inlineconstexpr |
Whether a type occurs in a pack or not.
- Template Parameters
-
query_t | The type you are searching for. |
pack_t | The type pack. |
- Returns
true
or false
.
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(n), possibly ==
i
, where i
is the index of the first occurrence
int main()
{
static_assert(seqan3::pack_traits::find<double, int, float, bool> == -1);
static_assert(seqan3::pack_traits::find<bool, int, float, bool> == 2);
}
◆ contains [2/2]
template<typename query_t , seqan3::detail::type_list_specialisation list_t>
constexpr bool seqan3::list_traits::contains = (find<query_t, list_t> != -1) |
|
inlineconstexpr |
Whether a type occurs in a type list or not.
- Template Parameters
-
query_t | The type you are searching for. |
pack_t | The type pack. |
- Returns
true
or false
.
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(n), possibly ==
i
, where i
is the index of the first occurrence
int main()
{
static_assert(seqan3::pack_traits::find<double, int, float, bool> == -1);
static_assert(seqan3::pack_traits::find<bool, int, float, bool> == 2);
}
int main()
{
static_assert(seqan3::list_traits::contains<double, list_t> == false);
static_assert(seqan3::list_traits::contains<float, list_t> == true);
}
◆ count
template<typename query_t , typename ... pack_t>
constexpr ptrdiff_t seqan3::pack_traits::count = (SEQAN3_IS_SAME(query_t, pack_t) + ... + 0) |
|
inlineconstexpr |
Count the occurrences of a type in a pack.
- Template Parameters
-
query_t | The type you are searching for. |
pack_t | The type pack. |
- Returns
- The number of occurrences of the
query_t
in pack_t
.
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(n)
int main()
{
static_assert(seqan3::pack_traits::count<int, int, float, bool, int> == 2);
}
◆ count< query_t, type_list< pack_t... > >
template<typename query_t , typename ... pack_t>
constexpr ptrdiff_t seqan3::list_traits::count< query_t, type_list< pack_t... > > |
|
inlineconstexpr |
Initial value:
Count the occurrences of a type in a type list.
- Template Parameters
-
query_t | The type you are searching for. |
pack_t | The type pack. |
- Returns
- The number of occurrences of the
query_t
in pack_t
.
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(n)
int main()
{
static_assert(seqan3::pack_traits::count<int, int, float, bool, int> == 2);
}
int main()
{
static_assert(seqan3::list_traits::count<int, list_t> == 2);
}
◆ find
template<typename query_t , typename ... pack_t>
constexpr ptrdiff_t seqan3::pack_traits::find = seqan3::pack_traits::detail::find<query_t, pack_t...>() |
|
inlineconstexpr |
Get the index of the first occurrence of a type in a pack.
- Template Parameters
-
query_t | The type you are searching for. |
pack_t | The type pack. |
- Returns
- The position of the first occurrence of
query_t
in pack_t
or -1
if it is not contained.
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(n), possibly ==
i
, where i
is the return value
int main()
{
static_assert(seqan3::pack_traits::find<double, int, float, bool> == -1);
static_assert(seqan3::pack_traits::find<bool, int, float, bool> == 2);
}
◆ find< query_t, type_list< pack_t... > >
template<typename query_t , typename ... pack_t>
constexpr ptrdiff_t seqan3::list_traits::find< query_t, type_list< pack_t... > > |
|
inlineconstexpr |
Initial value:=
seqan3::pack_traits::detail::find<query_t, pack_t...>()
Get the index of the first occurrence of a type in a type list.
- Template Parameters
-
query_t | The type you are searching for. |
pack_t | The type pack. |
- Returns
- The position of the first occurrence of
query_t
in pack_t
or -1
if it is not contained.
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(n), possibly ==
i
, where i
is the return value
int main()
{
static_assert(seqan3::pack_traits::find<double, int, float, bool> == -1);
static_assert(seqan3::pack_traits::find<bool, int, float, bool> == 2);
}
int main()
{
static_assert(seqan3::list_traits::find<double, list_t> == -1);
static_assert(seqan3::list_traits::find<bool, list_t> == 2);
}
◆ find_if
template<template< typename > typename pred_t, typename ... pack_t>
constexpr ptrdiff_t seqan3::pack_traits::find_if = seqan3::pack_traits::detail::find_if<pred_t, pack_t...>() |
|
inlineconstexpr |
Get the index of the first type in a pack that satisfies the given predicate.
- Template Parameters
-
pred_t | The predicate that is being evaluated (a class template). |
pack_t | The type pack. |
- Returns
- The index or
-1
if no types match.
Note that the predicate must be given as a type template (variable templates cannot be passed as template arguments unfortunately). This means e.g. find_if<std::is_integral, float, double, int, float>
(not std::is_integral_v
!).
(Compile-time) Complexity
- Number of template instantiations: O(n), possibly ==
i
, where i
is the return value
Other operations: O(n), possibly == i
, where i
is the return value
Only the predicate is instantiated.
int main()
{
static_assert(seqan3::pack_traits::find_if<std::is_pointer, int, float, double> == -1);
static_assert(seqan3::pack_traits::find_if<std::is_integral, int, float, double> == 0);
}
◆ find_if< pred_t, type_list< pack_t... > >
template<template< typename > typename pred_t, typename ... pack_t>
constexpr ptrdiff_t seqan3::list_traits::find_if< pred_t, type_list< pack_t... > > |
|
inlineconstexpr |
Initial value:=
seqan3::pack_traits::detail::find_if<pred_t, pack_t...>()
Get the index of the first type in a type list that satisfies the given predicate.
- Template Parameters
-
pred_t | The predicate that is being evaluated (a class template). |
pack_t | The type pack. |
- Returns
- The index or
-1
if no types match.
Note that the predicate must be given as a type template (variable templates cannot be passed as template arguments unfortunately). This means e.g. find_if<std::is_integral, float, double, int, float>
(not std::is_integral_v
!).
(Compile-time) Complexity
- Number of template instantiations: O(n), possibly ==
i
, where i
is the return value
Other operations: O(n), possibly == i
, where i
is the return value
Only the predicate is instantiated.
int main()
{
static_assert(seqan3::pack_traits::find_if<std::is_pointer, int, float, double> == -1);
static_assert(seqan3::pack_traits::find_if<std::is_integral, int, float, double> == 0);
}
int main()
{
static_assert(seqan3::list_traits::find<double, list_t> == -1);
static_assert(seqan3::list_traits::find<bool, list_t> == 2);
}
◆ size
template<typename ... pack_t>
constexpr size_t seqan3::pack_traits::size = sizeof...(pack_t) |
|
inlineconstexpr |
The size of a type pack.
- Template Parameters
-
- Returns
sizeof...(pack_t)
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(1)
int main()
{
static_assert(seqan3::pack_traits::size<int, float, bool, int> == 4);
}
◆ size< type_list< pack_t... > >
template<typename ... pack_t>
constexpr size_t seqan3::list_traits::size< type_list< pack_t... > > = sizeof...(pack_t) |
|
inlineconstexpr |
The size of a type list.
- Template Parameters
-
- Returns
sizeof...(pack_t)
(Compile-time) Complexity
- Number of template instantiations: O(1)
- Other operations: O(1)
int main()
{
static_assert(seqan3::pack_traits::size<int, float, bool, int> == 4);
}
int main()
{
static_assert(seqan3::list_traits::size<list_t> == 4);
}
decltype(detail::transform< trait_t >(list_t{})) transform
Apply a transformation trait to every type in the list and return a seqan3::type_list of the results.
Definition: traits.hpp:731
seqan3::list_traits::take_last< i, type_list< pack_t... > > take_last
Return a seqan3::type_list of the last n types in the type pack.
Definition: traits.hpp:827
decltype(detail::replace_at< replace_t >(detail::split_after< i >(type_list<>{}, list_t{}))) replace_at
Replace the type at the given index with the given type.
Definition: traits.hpp:752
meta::list< types... > type_list
Type that contains multiple types, an alias for meta::list.
Definition: type_list.hpp:31
typename decltype(detail::back(list_t{}))::type back
Return the last type from the type list.
Definition: traits.hpp:564
decltype(detail::drop_front(list_t{})) drop_front
Return a seqan3::type_list of all the types in the type list, except the first.
Definition: traits.hpp:611
typename decltype(detail::split_after< i >(type_list<>{}, list_t{}))::first_type take
Return a seqan3::type_list of the first n types in the input type list.
Definition: traits.hpp:631
take< size< list_t > - i, list_t > drop_last
Return a seqan3::type_list of the types the input type list, except the last n.
Definition: traits.hpp:691
typename decltype(detail::split_after< i >(type_list<>{}, list_t{}))::second_type drop
Return a seqan3::type_list of the types in the input type list, except the first n.
Definition: traits.hpp:651
drop< size< list_t > - i, list_t > take_last
Return a seqan3::type_list of the last n types in the input type list.
Definition: traits.hpp:671
typename decltype(detail::drop_front< pack_t... >())::type drop_front
Return a seqan3::type_list of all the types in the type pack, except the first.
Definition: traits.hpp:287
Provides various transformation traits used by the range module.
typename decltype(detail::front(list_t{}))::type front
Return the first type from the type list.
Definition: traits.hpp:542
typename decltype(detail::at< idx >(list_t{}))::type at
Return the type at given index from the type list.
Definition: traits.hpp:523
typename decltype(detail::front< pack_t... >())::type front
Return the first type from the type pack.
Definition: traits.hpp:240
seqan3::list_traits::drop< i, type_list< pack_t... > > drop
Return a seqan3::type_list of the types in the type pack, except the first n.
Definition: traits.hpp:807
typename decltype((std::type_identity< pack_t >{},...))::type back
Return the last type from the type pack.
Definition: traits.hpp:262
seqan3::list_traits::take< i, type_list< pack_t... > > take
Return a seqan3::type_list of the first n types in the type pack.
Definition: traits.hpp:787
decltype(detail::concat(lists_t{}...)) concat
Join two seqan3::type_list s into one.
Definition: traits.hpp:592
seqan3::type_list< trait_t< pack_t >... > transform
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
Definition: traits.hpp:307
Provides traits for seqan3::type_list.
typename decltype(detail::at< idx, pack_t... >())::type at
Return the type at given index from the type pack.
Definition: traits.hpp:221
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134