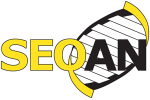 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
183 char const *
const *
const argv,
184 bool version_check =
true,
186 version_check_dev_decision{version_check}
189 throw design_error{
"The application name must only contain alpha-numeric characters "
190 "or '_' and '-' (regex: \"^[a-zA-Z0-9_-]+$\")."};
191 for (
auto & sub : subcommands)
193 throw design_error{
"The subcommand name must only contain alpha-numeric characters or '_'."};
196 init(argc, argv,
std::move(subcommands));
203 if (version_check_future.
valid())
231 template <
typename option_type, val
idator val
idator_type = detail::default_val
idator<option_type>>
235 std::invocable<validator_type, option_type>
242 validator_type option_validator = validator_type{})
244 if (sub_parser !=
nullptr)
245 throw design_error{
"You may only specify flags for the top-level parser."};
247 verify_identifiers(short_id, long_id);
250 std::visit([=, &value] (
auto & f) { f.add_option(value, short_id, long_id, desc, spec, option_validator); },
268 verify_identifiers(short_id, long_id);
271 std::visit([=, &value] (
auto & f) { f.add_flag(value, short_id, long_id, desc, spec); }, format);
294 template <
typename option_type, val
idator val
idator_type = detail::default_val
idator<option_type>>
298 std::invocable<validator_type, option_type>
302 validator_type option_validator = validator_type{})
304 if (sub_parser !=
nullptr)
305 throw design_error{
"You may only specify flags for the top-level parser."};
307 if (has_positional_list_option)
308 throw design_error{
"You added a positional option with a list value before so you cannot add "
309 "any other positional options."};
312 has_positional_list_option =
true;
316 std::visit([=, &value] (
auto & f) { f.add_positional_option(value, desc, option_validator); }, format);
389 if (parse_was_called)
390 throw design_error(
"The function parse() must only be called once!");
394 if (app_version.decide_if_check_is_performed(version_check_dev_decision, version_check_user_decision))
398 version_check_future = app_version_prom.
get_future();
399 app_version(
std::move(app_version_prom));
403 parse_was_called =
true;
410 if (sub_parser ==
nullptr)
412 throw design_error(
"You did not enable subcommand parsing on construction "
413 "so you cannot access the sub-parser!");
430 std::visit([&] (
auto & f) { f.add_section(title, spec); }, format);
441 std::visit([&] (
auto & f) { f.add_subsection(title, spec); }, format);
455 std::visit([&] (
auto & f) { f.add_line(text, is_paragraph, spec); }, format);
478 std::visit([&] (
auto & f) { f.add_list_item(key, desc, spec); }, format);
534 bool parse_was_called{
false};
537 bool has_positional_list_option{
false};
540 bool version_check_dev_decision{};
546 friend struct ::seqan3::detail::test_accessor;
552 std::regex app_name_regex{
"^[a-zA-Z0-9_-]+$"};
597 format = detail::format_short_help{};
601 bool special_format_was_set{
false};
603 for(
int i = 1, argv_len = argc; i < argv_len; ++i)
609 sub_parser = std::make_unique<argument_parser>(
info.
app_name +
"-" + arg, argc - i, argv + i,
false);
612 if (arg ==
"-h" || arg ==
"--help")
614 format = detail::format_help{subcommands,
false};
615 init_standard_options();
616 special_format_was_set =
true;
618 else if (arg ==
"-hh" || arg ==
"--advanced-help")
620 format = detail::format_help{subcommands,
true};
621 init_standard_options();
622 special_format_was_set =
true;
624 else if (arg ==
"--version")
626 format = detail::format_version{};
627 special_format_was_set =
true;
629 else if (arg.substr(0, 13) ==
"--export-help")
635 export_format = arg.
substr(14);
639 if (argv_len <= i + 1)
640 throw too_few_arguments{
"Option --export-help must be followed by a value."};
641 export_format = {argv[i+1]};
644 if (export_format ==
"html")
645 format = detail::format_html{subcommands};
646 else if (export_format ==
"man")
647 format = detail::format_man{subcommands};
652 throw validation_error{
"Validation failed for option --export-help: "
653 "Value must be one of [html, man]"};
654 init_standard_options();
655 special_format_was_set =
true;
657 else if (arg ==
"--copyright")
659 format = detail::format_copyright{};
660 special_format_was_set =
true;
662 else if (arg ==
"--version-check")
665 throw too_few_arguments{
"Option --version-check must be followed by a value."};
670 version_check_user_decision =
true;
672 version_check_user_decision =
false;
674 throw validation_error{
"Value for option --version-check must be 1 or 0."};
684 if (!special_format_was_set)
686 if (!subcommands.
empty() && sub_parser ==
nullptr)
688 throw too_few_arguments{detail::to_string(
"Please specify which sub program you want to use ",
689 "(one of ", subcommands,
"). Use -h/--help for more information.")};
692 format = detail::format_parse(argc,
std::move(argv_new));
697 void init_standard_options()
700 add_list_item(
"\\fB-h\\fP, \\fB--help\\fP",
"Prints the help page.");
702 "Prints the help page including advanced options.");
703 add_list_item(
"\\fB--version\\fP",
"Prints the version information.");
704 add_list_item(
"\\fB--copyright\\fP",
"Prints the copyright/license information.");
706 "Export the help page information. Value must be one of [html, man].");
707 if (version_check_dev_decision)
708 add_list_item(
"\\fB--version-check\\fP (bool)",
"Whether to to check for the newest app version. Default: 1.");
717 template <
typename id_type>
718 bool id_exists(id_type
const &
id)
720 if (detail::format_parse::is_empty_id(
id))
734 void verify_identifiers(
char const short_id,
std::string const & long_id)
736 auto constexpr allowed =
is_alnum || is_char<'_'> || is_char<'@'>;
738 if (id_exists(short_id))
739 throw design_error(
"Option Identifier '" +
std::string(1, short_id) +
"' was already used before.");
740 if (id_exists(long_id))
741 throw design_error(
"Option Identifier '" + long_id +
"' was already used before.");
742 if (long_id.
length() == 1)
743 throw design_error(
"Long IDs must be either empty, or longer than one character.");
744 if (!allowed(short_id) && !is_char<'\0'>(short_id))
745 throw design_error(
"Option identifiers may only contain alphanumeric characters, '_', or '@'.");
746 if (long_id.
size() > 0 && is_char<'-'>(long_id[0]))
747 throw design_error(
"First character of long ID cannot be '-'.");
751 if (!(allowed(c) || is_char<
'-'>(c)))
752 throw design_error(
"Long identifiers may only contain alphanumeric characters, '_', '-', or '@'.");
754 if (detail::format_parse::is_empty_id(short_id) && detail::format_parse::is_empty_id(long_id))
755 throw design_error(
"Option Identifiers cannot both be empty.");
764 detail::format_short_help,
765 detail::format_version,
768 detail::format_copyright
769 > format{detail::format_help{{},
false}};
772 std::set<std::string> used_option_ids{
"h",
"hh",
"help",
"advanced-help",
"export-help",
"version",
"copyright"};
constexpr auto is_alnum
Checks whether c is a alphanumeric character.
Definition: predicate.hpp:220
void add_subsection(std::string const &title, option_spec const spec=option_spec::DEFAULT)
Adds an help page subsection to the seqan3::argument_parser.
Definition: argument_parser.hpp:439
argument_parser(argument_parser &&)=default
Defaulted.
option_spec
Used to further specify argument_parser options/flags.
Definition: auxiliary.hpp:233
A more refined container concept than seqan3::container.
argument_parser & get_sub_parser()
Returns a reference to the sub-parser instance if subcommand parsing was enabled.
Definition: argument_parser.hpp:408
The SeqAn command line parser.
Definition: argument_parser.hpp:154
void add_line(std::string const &text, bool is_paragraph=false, option_spec const spec=option_spec::DEFAULT)
Adds an help page text line to the seqan3::argument_parser.
Definition: argument_parser.hpp:453
void add_option(option_type &value, char const short_id, std::string const &long_id, std::string const &desc, option_spec const spec=option_spec::DEFAULT, validator_type option_validator=validator_type{})
Adds an option to the seqan3::argument_parser.
Definition: argument_parser.hpp:237
constexpr ptrdiff_t find
Get the index of the first occurrence of a type in a pack.
Definition: traits.hpp:152
@ DEFAULT
The default were no checking or special displaying is happening.
Definition: auxiliary.hpp:234
Checks if program is run interactively and retrieves dimensions of terminal (Transferred from seqan2)...
argument_parser(argument_parser const &)=default
Defaulted.
argument_parser()=delete
Deleted.
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
void add_section(std::string const &title, option_spec const spec=option_spec::DEFAULT)
Adds an help page section to the seqan3::argument_parser.
Definition: argument_parser.hpp:428
void add_positional_option(option_type &value, std::string const &desc, validator_type option_validator=validator_type{})
Adds a positional option to the seqan3::argument_parser.
Definition: argument_parser.hpp:300
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
void add_list_item(std::string const &key, std::string const &desc, option_spec const spec=option_spec::DEFAULT)
Adds an help page list item (key-value) to the seqan3::argument_parser.
Definition: argument_parser.hpp:476
void add_flag(bool &value, char const short_id, std::string const &long_id, std::string const &desc, option_spec const spec=option_spec::DEFAULT)
Adds a flag to the seqan3::argument_parser.
Definition: argument_parser.hpp:262
argument_parser & operator=(argument_parser const &)=default
Defaulted.
argument_parser(std::string const app_name, int const argc, char const *const *const argv, bool version_check=true, std::vector< std::string > subcommands={})
Initializes an seqan3::argument_parser object from the command line arguments.
Definition: argument_parser.hpp:181
Checks whether the the type can be used in an add_(positional_)option call on the argument parser.
~argument_parser()
The destructor.
Definition: argument_parser.hpp:200
Provides character predicates for tokenisation.
argument_parser_meta_data info
Aggregates all parser related meta data (see seqan3::argument_parser_meta_data struct).
Definition: argument_parser.hpp:530
Auxiliary for pretty printing of exception messages.
void parse()
Initiates the actual command line parsing.
Definition: argument_parser.hpp:387
argument_parser & operator=(argument_parser &&)=default
Defaulted.
Argument parser exception that is thrown whenever there is an design error directed at the developer ...
Definition: exceptions.hpp:171
Forward declares seqan3::detail::test_accessor.
Provides the version check functionality.