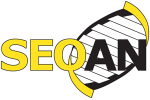 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
92 template <
typename stream_type,
93 typename seq_legal_alph_type,
bool seq_qual_combined,
101 qual_type & SEQAN3_DOXYGEN_ONLY(qualities))
104 auto stream_it = std::ranges::begin(stream_view);
108 std::cpp20::back_inserter(idbuffer));
109 if (idbuffer !=
"ID")
110 throw parse_error{
"An entry has to start with the code word ID."};
112 if constexpr (!detail::decays_to_ignore_v<id_type>)
116 std::ranges::copy(idbuffer |
views::char_to<std::ranges::range_value_t<id_type>>, std::cpp20::back_inserter(
id));
121 std::cpp20::back_inserter(
id));
122 id.push_back(*stream_it);
124 }
while (*stream_it !=
'Q');
138 std::cpp20::back_inserter(
id));
144 std::cpp20::back_inserter(
id));
156 }
while (*stream_it !=
'Q');
161 auto constexpr is_end =
is_char<
'/'> ;
162 if constexpr (!detail::decays_to_ignore_v<seq_type>)
167 auto constexpr is_legal_alph = is_in_alphabet<seq_legal_alph_type>;
170 if (!is_legal_alph(c))
174 " evaluated to false on " +
175 detail::make_printable(c)};
180 std::cpp20::back_inserter(
sequence));
193 template <
typename stream_type,
201 qual_type && SEQAN3_DOXYGEN_ONLY(qualities))
203 seqan3::detail::fast_ostreambuf_iterator stream_it{*stream.rdbuf()};
205 [[maybe_unused]]
size_t sequence_size = 0;
207 if constexpr (!detail::decays_to_ignore_v<seq_type>)
208 sequence_size = std::ranges::distance(
sequence);
211 if constexpr (detail::decays_to_ignore_v<id_type>)
213 throw std::logic_error{
"The ID field may not be set to ignore when writing embl files."};
217 if (ranges::empty(
id))
222 stream_it.write_range(
id);
227 stream_it.write_range(
id);
229 stream_it.write_number(sequence_size);
235 if constexpr (detail::decays_to_ignore_v<seq_type>)
237 throw std::logic_error{
"The SEQ field may not be set to ignore when writing embl files."};
246 stream_it.write_number(sequence_size);
251 auto it = std::ranges::begin(char_sequence);
252 size_t written_chars{0};
253 uint8_t chunk_size{10u};
255 while (it != std::ranges::end(char_sequence))
257 auto current_end = it;
258 size_t steps = std::ranges::advance(current_end, chunk_size, std::ranges::end(char_sequence));
260 using subrange_t = std::ranges::subrange<decltype(it), decltype(it), std::ranges::subrange_kind::sized>;
261 it = stream_it.write_range(subrange_t{it, current_end, chunk_size - steps});
263 written_chars += chunk_size;
265 if (written_chars % 60 == 0)
267 stream_it.write_number(written_chars);
273 auto characters_in_last_line = sequence_size % 60;
274 auto number_of_padding_needed = 65 - characters_in_last_line - characters_in_last_line / chunk_size;
276 stream_it.write_number(sequence_size);
Provides seqan3::views::take_until and seqan3::views::take_until_or_throw.
auto const char_to
A view over an alphabet, given a range of characters.
Definition: char_to.hpp:69
bool embl_genbank_complete_header
Complete header given for embl or genbank.
Definition: output_options.hpp:42
constexpr auto is_space
Checks whether c is a space character.
Definition: predicate.hpp:146
Adaptations of algorithms from the Ranges TS.
Provides seqan3::views::repeat_n.
Thrown if there is a parse error, such as reading an unexpected character from an input stream.
Definition: exception.hpp:48
Provides various transformation traits used by the range module.
Provides seqan3::dna5, container aliases and string literals.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
constexpr auto take_until
A view adaptor that returns elements from the underlying range until the functor evaluates to true (o...
Definition: take_until.hpp:610
The options type defines various option members that influence the behaviour of all or some formats.
Definition: output_options.hpp:22
constexpr auto is_digit
Checks whether c is a digital character.
Definition: predicate.hpp:287
bool add_carriage_return
The default plain text line-ending is "\n", but on Windows an additional carriage return is recommend...
Definition: output_options.hpp:39
Provides seqan3::sequence_file_output_options.
constexpr auto is_cntrl
Checks whether c is a control character.
Definition: predicate.hpp:110
Provides seqan3::views::to_char.
Adaptations of concepts from the Ranges TS.
constexpr auto take_line_or_throw
A view adaptor that returns a single line from the underlying range (throws if there is no end-of-lin...
Definition: take_line.hpp:90
constexpr auto take_until_or_throw
A view adaptor that returns elements from the underlying range until the functor evaluates to true (t...
Definition: take_until.hpp:624
Provides character predicates for tokenisation.
SeqAn specific customisations in the standard namespace.
auto const to_char
A view that calls seqan3::to_char() on each element in the input range.
Definition: to_char.hpp:65
Provides seqan3::views::istreambuf.
constexpr auto repeat_n
A view factory that repeats a given value n times.
Definition: repeat_n.hpp:94
seqan3::type_list< trait_t< pack_t >... > transform
Apply a transformation trait to every type in the pack and return a seqan3::type_list of the results.
Definition: traits.hpp:307
Provides seqan3::views::take_line and seqan3::views::take_line_or_throw.
Provides seqan3::fast_istreambuf_iterator and seqan3::fast_ostreambuf_iterator, as well as,...
Provides various utility functions.
constexpr auto is_char
Checks whether a given letter is the same as the template non-type argument.
Definition: predicate.hpp:83
constexpr auto is_blank
Checks whether c is a blank character.
Definition: predicate.hpp:163
Provides various utility functions.
The generic concept for a sequence.
Provides seqan3::views::char_to.
constexpr auto istreambuf
A view factory that returns a view over the stream buffer of an input stream.
Definition: istreambuf.hpp:113