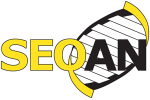 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
19 #include <type_traits>
79 template <std::ranges::viewable_range inner_type>
81 requires std::ranges::random_access_range<inner_type> && std::ranges::sized_range<inner_type> &&
82 (std::is_const_v<std::remove_reference_t<inner_type>> || std::ranges::view<inner_type>)
97 using ungapped_view_type = decltype(
views::type_reduce(std::declval<inner_type &&>()));
111 using size_type = std::ranges::range_size_t<inner_type>;
132 template <
typename other_range_t>
134 requires (!std::same_as<other_range_t, gap_decorator>) &&
136 std::ranges::viewable_range<other_range_t>
156 return anchors.rbegin()->second + ungapped_view.size();
158 return ungapped_view.size();
180 assert(pos <=
size());
182 set_iterator_type it_set = anchors.upper_bound(anchor_gap_t{pos, bound_dummy});
184 if (it_set == anchors.begin())
186 anchors.emplace_hint(anchors.begin(), anchor_gap_t{pos, count});
191 auto gap_len{it_set->second};
192 if (it_set != anchors.begin())
193 gap_len -= (*(
std::prev(it_set))).second;
195 if (it_set->first + gap_len >= pos)
197 anchor_gap_t
gap{it_set->first, it_set->second +
count};
198 it_set = anchors.erase(it_set);
199 anchors.insert(it_set,
gap);
203 anchor_gap_t
gap{pos, it_set->second +
count};
205 anchors.insert(it_set,
gap);
229 throw gap_erase_failure(
"The range to be erased does not correspond to a consecutive gap.");
251 set_iterator_type it = anchors.upper_bound(anchor_gap_t{pos1, bound_dummy});
253 if (it == anchors.begin())
258 size_type const gap_len = gap_length(it);
261 if ((it->first + gap_len) < pos2)
263 throw gap_erase_failure{
"The range to be erased does not correspond to a consecutive gap."};
266 else if (gap_len == pos2 - pos1)
268 it = anchors.erase(it);
273 anchor_gap_t
gap{it->first, it->second - pos2 + pos1};
274 it = anchors.erase(it);
275 it = anchors.insert(it,
gap);
280 update(it, pos2 - pos1);
289 template <
typename unaligned_seq_t>
291 requires std::assignable_from<gap_decorator &, unaligned_seq_t>
369 throw std::out_of_range{
"Trying to access element behind the last in gap_decorator."};
377 throw std::out_of_range{
"Trying to access element behind the last in gap_decorator."};
421 lhs.anchors == rhs.anchors &&
422 std::ranges::equal(lhs.ungapped_view, rhs.ungapped_view))
433 return !(lhs == rhs);
439 auto lit = lhs.
begin();
440 auto rit = rhs.
begin();
442 while (lit != lhs.
end() && rit != rhs.
end() && *lit == *rit)
445 if (rit == rhs.
end())
447 else if (lit == lhs.
end())
456 auto lit = lhs.
begin();
457 auto rit = rhs.
begin();
459 while (lit != lhs.
end() && rit != rhs.
end() && *lit == *rit)
462 if (lit == lhs.
end())
464 else if (rit == rhs.
end())
473 return !(lhs <= rhs);
491 using set_iterator_type =
typename anchor_set_type::iterator;
508 size_type gap_length(set_iterator_type it)
const
510 return (it == anchors.begin()) ? it->second : it->second - (*
std::prev(it)).second;
525 void rupdate(size_type
const pos, size_type
const offset)
527 for (
auto it =
std::prev(anchors.end(), 1); it->first > pos;)
529 anchors.emplace_hint(it, anchor_gap_t{it->first + offset, it->second + offset});
530 anchors.erase(*it--);
546 void update(set_iterator_type it, size_type
const offset)
548 while (it != anchors.end())
550 anchor_gap_t gap{it->first - offset, it->second - offset};
551 it = anchors.erase(it);
552 it = anchors.insert(it, gap);
558 ungapped_view_type ungapped_view{};
561 anchor_set_type anchors{};
567 template <std::ranges::viewable_range urng_t>
575 template <
std::ranges::view urng_t>
595 template <
std::ranges::viewable_range inner_type>
597 requires
std::ranges::random_access_range<inner_type> &&
std::ranges::sized_range<inner_type> &&
598 (
std::is_const_v<
std::remove_reference_t<inner_type>> ||
std::ranges::view<inner_type>)
608 int64_t ungapped_view_pos{0};
614 typename gap_decorator::set_iterator_type anchor_set_it{};
616 bool is_at_gap{
true};
621 assert(new_pos <= host->
size());
624 anchor_set_it = host->anchors.upper_bound(anchor_gap_t{pos, host->bound_dummy});
625 ungapped_view_pos = pos;
627 if (anchor_set_it != host->anchors.begin())
629 typename gap_decorator::set_iterator_type prev{
std::prev(anchor_set_it)};
632 if (prev != host->anchors.begin())
635 ungapped_view_pos -= prev->second;
636 left_gap_end = prev->first + gap_len;
639 if (ungapped_view_pos !=
static_cast<int64_t
>(host->ungapped_view.size()) &&
640 pos >= left_gap_end && (anchor_set_it == host->anchors.end() || pos < anchor_set_it->first))
674 host(&host_), anchor_set_it{host_.anchors.
begin()}
676 if (host_.anchors.size() && (*host_.anchors.begin()).first == 0)
679 left_gap_end = anchor_set_it->second;
704 if (pos < left_gap_end)
707 if (anchor_set_it == host->anchors.end() || pos < anchor_set_it->first)
710 if (ungapped_view_pos !=
static_cast<int64_t
>(host->ungapped_view.size()))
715 left_gap_end = anchor_set_it->first + anchor_set_it->second -
716 ((anchor_set_it != host->anchors.begin()) ? (
std::prev(anchor_set_it))->second : 0);
720 if (left_gap_end == host->size())
738 this->jump(this->pos + skip);
760 if (pos < left_gap_end)
762 (anchor_set_it != host->anchors.begin()) ? --anchor_set_it : anchor_set_it;
764 if (anchor_set_it != host->anchors.begin())
767 left_gap_end = prev->first + prev->second -
768 ((prev != host->anchors.begin()) ?
std::prev(prev)->second : 0);
776 else if (anchor_set_it == host->anchors.end() || pos < anchor_set_it->first)
797 this->jump(this->pos - skip);
826 return (is_at_gap) ?
reference{
gap{}} : reference{host->ungapped_view[ungapped_view_pos]};
844 return lhs.pos == rhs.pos;
850 return lhs.pos != rhs.pos;
856 return lhs.pos < rhs.pos;
862 return lhs.pos > rhs.pos;
868 return lhs.pos <= rhs.pos;
874 return lhs.pos >= rhs.pos;
gap_decorator_iterator operator--(int)
Returns a decremented iterator copy.
Definition: gap_decorator.hpp:787
const_iterator cbegin() const noexcept
Returns an iterator to the first element of the container.
Definition: gap_decorator.hpp:321
The alphabet of a gap character '-'.
Definition: gap.hpp:37
gap_decorator_iterator & operator=(gap_decorator_iterator const &)=default
Defaulted.
Thrown in function seqan3::erase_gap, if a position does not contain a gap.
Definition: exception.hpp:24
inner_type unaligned_seq_type
The underlying ungapped range type.
Definition: gap_decorator.hpp:117
gap_decorator(gap_decorator const &)=default
Defaulted.
const_iterator begin() const noexcept
Returns an iterator to the first element of the container.
Definition: gap_decorator.hpp:315
Adaptations of concepts from the standard library.
const_iterator end() const noexcept
Returns an iterator pointing behind the last element of the decorator.
Definition: gap_decorator.hpp:339
reference operator[](difference_type const n) const
Return underlying container value currently pointed at.
Definition: gap_decorator.hpp:830
constexpr auto type_reduce
A view adaptor that behaves like std::views::all, but type erases certain ranges.
Definition: type_reduce.hpp:158
Includes customized exception types for the alignment module .
friend bool operator>=(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is greater than or equal to rhs.
Definition: gap_decorator.hpp:872
friend bool operator>=(gap_decorator const &lhs, gap_decorator const &rhs)
Checks whether lhs is greater than or equal to rhs.
Definition: gap_decorator.hpp:477
Adaptations of algorithms from the Ranges TS.
const_reference at(size_type const i) const
Return the i-th element as a reference.
Definition: gap_decorator.hpp:374
typename gap_decorator::const_reference reference
The reference type.
Definition: gap_decorator.hpp:655
gap_decorator_iterator operator+(difference_type const skip) const
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:743
A combined alphabet that can hold values of either of its alternatives.
Definition: alphabet_variant.hpp:133
gap_decorator_iterator & operator=(gap_decorator_iterator &&)=default
Defaulted.
Provides the seqan3::detail::random_access_iterator class.
value_type * pointer
The pointer type.
Definition: gap_decorator.hpp:657
gap_decorator & operator=(gap_decorator &&rhs)=default
Defaulted.
iterator erase_gap(const_iterator const it)
Erase one gap symbol at the indicated iterator postion.
Definition: gap_decorator.hpp:225
gap_decorator_iterator(gap_decorator_iterator &&)=default
Defaulted.
friend gap_decorator_iterator operator-(difference_type const skip, gap_decorator_iterator const &it)
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:808
Provides seqan3::views::type_reduce.
reference at(size_type const i)
Return the i-th element as a reference.
Definition: gap_decorator.hpp:366
friend bool operator>(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is greater than rhs.
Definition: gap_decorator.hpp:860
gap_decorator_iterator operator-(difference_type const skip) const
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:802
gap_decorator(other_range_t &&range)
Construct with the ungapped range type.
Definition: gap_decorator.hpp:138
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
friend bool operator<=(gap_decorator const &lhs, gap_decorator const &rhs)
Checks whether lhs is less than or equal to rhs.
Definition: gap_decorator.hpp:454
gap_decorator_iterator & operator--()
Decrements iterator.
Definition: gap_decorator.hpp:755
typename gap_decorator::value_type value_type
The value type.
Definition: gap_decorator.hpp:653
void jump(typename gap_decorator::size_type const new_pos)
A helper function that performs the random access into the anchor set, updating all member variables.
Definition: gap_decorator.hpp:619
friend bool operator<(gap_decorator const &lhs, gap_decorator const &rhs)
Checks whether lhs is less than rhs.
Definition: gap_decorator.hpp:437
friend bool operator!=(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is not equal to rhs.
Definition: gap_decorator.hpp:848
gap_decorator_iterator(gap_decorator const &host_)
Construct from seqan3::gap_decorator and initialising to first position.
Definition: gap_decorator.hpp:673
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
gap_decorator(gap_decorator &&rhs)=default
Defaulted.
gap_decorator_iterator & operator+=(difference_type const skip)
Advances iterator by skip many positions.
Definition: gap_decorator.hpp:736
Adaptations of concepts from the Ranges TS.
friend bool operator<=(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is less than or equal to rhs.
Definition: gap_decorator.hpp:866
gap_decorator_iterator(gap_decorator_iterator const &)=default
Defaulted.
~gap_decorator_iterator()=default
Defaulted.
friend bool operator<(gap_decorator_iterator const &lhs, gap_decorator_iterator const &rhs) noexcept
Checks whether *this is less than rhs.
Definition: gap_decorator.hpp:854
friend bool operator>(gap_decorator const &lhs, gap_decorator const &rhs)
Checks whether lhs is greater than rhs.
Definition: gap_decorator.hpp:471
friend bool operator!=(gap_decorator const &lhs, gap_decorator const &rhs)
Checks whether lhs is not equal to rhs.
Definition: gap_decorator.hpp:431
iterator erase_gap(const_iterator const first, const_iterator const last)
Erase gap symbols at the iterator postions [first, last[.
Definition: gap_decorator.hpp:247
difference_type operator-(gap_decorator_iterator const lhs) const noexcept
Returns the distance between two iterators.
Definition: gap_decorator.hpp:814
SeqAn specific customisations in the standard namespace.
iterator insert_gap(const_iterator const it, size_type const count=1)
Insert a gap of length count at the aligned sequence iterator position.
Definition: gap_decorator.hpp:174
friend gap_decorator_iterator operator+(difference_type const skip, gap_decorator_iterator const &it)
Returns an iterator copy advanced by skip many positions.
Definition: gap_decorator.hpp:749
The iterator type over a seqan3::gap_decorator.
Definition: gap_decorator.hpp:601
size_type size() const
Returns the total length of the aligned sequence.
Definition: gap_decorator.hpp:153
gapped< std::ranges::range_value_t< inner_type > > value_type
The variant type of the alphabet type and gap symbol type (see seqan3::gapped).
Definition: gap_decorator.hpp:104
reference operator[](size_type const i) const
Return the i-th element as a reference.
Definition: gap_decorator.hpp:392
gap_decorator_iterator & operator-=(difference_type const skip)
Advances iterator by skip many positions.
Definition: gap_decorator.hpp:795
std::ranges::range_difference_t< inner_type > difference_type
The difference type of the underlying sequence.
Definition: gap_decorator.hpp:113
friend void assign_unaligned(gap_decorator &dec, unaligned_seq_t &&unaligned)
Assigns a new sequence of type seqan3::gap_decorator::unaligned_seq_type to the decorator.
Definition: gap_decorator.hpp:293
gap_decorator_iterator operator++(int)
Returns an incremented iterator copy.
Definition: gap_decorator.hpp:728
A gap decorator allows the annotation of sequences with gap symbols while leaving the underlying sequ...
Definition: gap_decorator.hpp:85
gap_decorator & operator=(gap_decorator const &)=default
Defaulted.
typename gap_decorator::difference_type difference_type
The difference type.
Definition: gap_decorator.hpp:651
Provides the seqan3::detail::inherited_iterator_base template.
~gap_decorator()=default
Defaulted.
Core alphabet concept and free function/type trait wrappers.
constexpr ptrdiff_t count
Count the occurrences of a type in a pack.
Definition: traits.hpp:134
const_iterator cend() const noexcept
Returns an iterator pointing behind the last element of the decorator.
Definition: gap_decorator.hpp:345
std::ranges::range_size_t< inner_type > size_type
The size_type of the underlying sequence.
Definition: gap_decorator.hpp:111
gap_decorator_iterator()=default
Defaulted.
gap_decorator(urng_t &&range) -> gap_decorator< std::remove_reference_t< urng_t > const & >
Ranges (not views!) always deduce to const & range_type since they are access-only anyway.
gap_decorator_iterator(gap_decorator const &host_, typename gap_decorator::size_type const pos_)
Construct from seqan3::gap_decorator and explicit position.
Definition: gap_decorator.hpp:689
reference const_reference
const_reference type equals reference type equals value type because the underlying sequence must not...
Definition: gap_decorator.hpp:109