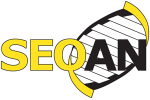 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
17 #include <meta/meta.hpp>
164 template <
field ...fs>
175 static constexpr
size_t index_of(
field f)
177 for (
size_t i = 0; i <
sizeof...(fs); ++i)
178 if (as_array[i] == f)
184 static constexpr
bool contains(
field f)
186 return index_of(f) != npos;
189 static_assert([] () constexpr
191 for (
size_t i = 0; i < as_array.
size(); ++i)
192 for (
size_t j = i + 1; j < as_array.
size(); ++j)
193 if (as_array[i] == as_array[j])
197 } (),
"You may not include a field twice into fields<>.");
224 template <
typename field_types,
typename field_
ids>
225 struct record : detail::transfer_template_args_onto_t<field_types, std::tuple>
229 template <
typename t>
231 requires requires (t & v) { v.
clear(); }
233 static constexpr
void clear_element(t & v) noexcept(noexcept(v.clear()))
239 template <
typename t>
240 static constexpr
void clear_element(t & v) noexcept(noexcept(std::declval<t &>() = t{}))
246 static constexpr
auto expander = [] (
auto & ...args) { (clear_element(args), ...); };
250 using base_type = detail::transfer_template_args_onto_t<field_types, std::tuple>;
263 using base_type::base_type;
267 "You must give as many IDs as types to seqan3::record.");
286 template <
typename field_types,
typename field_
ids>
287 struct tuple_size<
seqan3::record<field_types, field_ids>>
290 static constexpr
size_t value = tuple_size_v<typename seqan3::record<field_types, field_ids>::base_type>;
298 template <
size_t elem_no,
typename field_types,
typename field_
ids>
299 struct tuple_element<elem_no,
seqan3::record<field_types, field_ids>>
302 using type = std::tuple_element_t<elem_no, typename seqan3::record<field_types, field_ids>::base_type>;
316 template <field f,
typename field_types,
typename field_
ids>
320 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
321 return std::get<field_ids::index_of(f)>(r);
325 template <field f,
typename field_types,
typename field_
ids>
328 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
329 return std::get<field_ids::index_of(f)>(r);
333 template <field f,
typename field_types,
typename field_
ids>
336 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
337 return std::get<field_ids::index_of(f)>(
std::move(r));
341 template <field f,
typename field_types,
typename field_
ids>
344 static_assert(
field_ids::contains(f),
"The record does not contain the field you wish to retrieve.");
345 return std::get<field_ids::index_of(f)>(
std::move(r));
std::tuple_element_t< elem_no, typename seqan3::record< field_types, field_ids >::base_type > type
The member type. Delegates to same type on base_type.
Definition: record.hpp:302
record(record const &)=default
Defaulted.
record()=default
Defaulted.
@ seq
The "sequence", usually a range of nucleotides or amino acids.
@ offset
Sequence (SEQ) relative start position (0-based), unsigned value.
record & operator=(record &&)=default
Defaulted.
auto const & get(record< field_types, field_ids > const &r)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: record.hpp:326
@ id
The identifier, usually a string.
Provides seqan3::type_list and auxiliary type traits.
auto const && get(record< field_types, field_ids > const &&r)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: record.hpp:342
constexpr bool contains
Whether a type occurs in a pack or not.
Definition: traits.hpp:193
A class template that holds a choice of seqan3::field.
Definition: record.hpp:166
constexpr sequenced_policy seq
Global execution policy object for sequenced execution policy.
Definition: execution.hpp:54
@ cigar
The cigar vector (std::vector<seqan3::cigar>) representing the alignment in SAM/BAM format.
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
@ mapq
The mapping quality of the SEQ alignment, usually a ohred-scaled score.
auto & get(record< field_types, field_ids > &r)
Free function get() for seqan3::record based on seqan3::field.
Definition: record.hpp:318
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
record(record &&)=default
Defaulted.
record & operator=(record const &)=default
Defaulted.
auto && get(record< field_types, field_ids > &&r)
This is an overloaded member function, provided for convenience. It differs from the above function o...
Definition: record.hpp:334
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
SeqAn specific customisations in the standard namespace.
field
An enumerator for the fields used in file formats.
Definition: record.hpp:65
The class template that file records are based on; behaves like an std::tuple.
Definition: record.hpp:226
detail::transfer_template_args_onto_t< field_types, std::tuple > base_type
A specialisation of std::tuple.
Definition: record.hpp:250
@ flag
The alignment flag (bit information), uint16_t value.
~record()=default
Defaulted.
void clear() noexcept(noexcept(std::apply(expander, std::declval< record & >())))
Clears containers that provide .clear() and (re-)initialises all other elements with = {}.
Definition: record.hpp:270
@ header_ptr
A pointer to the seqan3::alignment_file_header object storing header information.
@ alignment
The (pairwise) alignment stored in an seqan3::alignment object.
Provides seqan3::type_list.