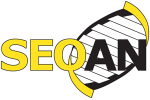 |
SeqAn3
3.0.2
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
79 template <
typename val
idator_type>
82 SEQAN3_CONCEPT
validator = std::copyable<std::remove_cvref_t<validator_type>> &&
125 if (!((cmp <= max) && (cmp >= min)))
126 throw validation_error{detail::to_string(
"Value ", cmp,
" is not in range [", min,
",", max,
"].")};
135 template <std::ranges::forward_range range_type>
141 std::for_each(range.begin(), range.end(), [&] (
auto cmp) { (*this)(cmp); });
147 return detail::to_string(
"Value must be in range [", min,
",", max,
"].");
175 template <
typename option_value_t>
197 template <std::ranges::forward_range range_type>
199 requires std::constructible_from<option_value_type, std::ranges::range_rvalue_reference_t<range_type>>
212 template <
typename ...option_types>
214 requires ((std::constructible_from<option_value_type, option_types> && ...))
218 (values.
emplace_back(std::forward<option_types>(opts)), ...);
229 throw validation_error{detail::to_string(
"Value ", cmp,
" is not one of ", std::views::all(values),
".")};
237 template <std::ranges::forward_range range_type>
239 requires std::convertible_to<std::ranges::range_value_t<range_type>,
option_value_type>
243 std::for_each(std::ranges::begin(range), std::ranges::end(range), [&] (
auto cmp) { (*this)(cmp); });
249 return detail::to_string(
"Value must be one of ", std::views::all(values),
".");
265 requires (!(detail::is_char_adaptation_v<option_types> || ...))
270 template <typename range_type>
272 requires
std::ranges::forward_range<
std::decay_t<range_type>> &&
274 (!
seqan3::detail::is_char_adaptation_v<
std::ranges::range_value_t<range_type>>)
279 template <typename ...option_types>
281 requires (
std::constructible_from<
std::
string,
std::decay_t<option_types>> && ...)
286 template <typename range_type>
288 requires (
std::ranges::forward_range<
std::decay_t<range_type>> &&
289 std::constructible_from<
std::
string,
std::ranges::range_value_t<range_type>>)
294 template <typename ...option_types>
298 template <typename range_type>
300 requires (
std::ranges::forward_range<
std::decay_t<range_type>>)
351 template <std::ranges::forward_range range_type>
357 std::for_each(v.begin(), v.end(), [&] (
auto cmp) { this->operator()(cmp); });
369 if (extensions.empty())
375 " has no extension. Expected one of the following valid"
376 " extensions:", extensions,
"!")};
382 auto case_insensitive_equal_to = [&] (
std::string const & ext)
384 return std::ranges::equal(ext, drop_less_ext, [] (
char const chr1,
char const chr2)
386 return std::tolower(chr1) == std::tolower(chr2);
393 throw validation_error{detail::to_string(
"Expected one of the following valid extensions: ",
394 extensions,
"! Got ", drop_less_ext,
" instead!")};
411 if (
static_cast<bool>(ec))
412 throw validation_error{detail::to_string(
"Cannot read the directory ", path ,
"!")};
418 throw validation_error{detail::to_string(
"Expected a regular file ", path,
"!")};
421 if (!file.is_open() || !file.good())
422 throw validation_error{detail::to_string(
"Cannot read the file ", path,
"!")};
435 detail::safe_filesystem_entry file_guard{path};
437 bool is_open = file.is_open();
438 bool is_good = file.good();
441 if (!is_good || !is_open)
450 if (extensions.empty())
453 return detail::to_string(
" Valid file extensions are: [", extensions | views::join(
std::string{
", "}),
"].");
482 template <
typename file_t =
void>
487 static_assert(std::same_as<file_t, void> || detail::has_type_valid_formats<file_t>,
488 "Expected either a template type with a static member called valid_formats (a file type) or void.");
507 if constexpr (!std::same_as<file_t, void>)
527 requires std::same_as<file_t, void>
539 using file_validator_base::operator();
551 throw validation_error{detail::to_string(
"The file ", file,
" does not exist!")};
572 return "The input file must exist and read permissions must be granted." +
599 template <
typename file_t =
void>
604 static_assert(std::same_as<file_t, void> || detail::has_type_valid_formats<file_t>,
605 "Expected either a template type with a static member called valid_formats (a file type) or void.");
617 if constexpr (!std::same_as<file_t, void>)
630 requires std::same_as<file_t, void>
642 using file_validator_base::operator();
654 throw validation_error{detail::to_string(
"The file ", file,
" already exists!")};
674 return "The output file must not exist already and write permissions must be granted." +
714 using file_validator_base::operator();
726 throw validation_error{detail::to_string(
"The directory ", dir,
" does not exists!")};
729 throw validation_error{detail::to_string(
"The path ", dir,
" is not a directory!")};
747 return detail::to_string(
"An existing, readable path for the input directory.");
786 using file_validator_base::operator();
800 if (
static_cast<bool>(ec))
801 throw validation_error{detail::to_string(
"Cannot create directory: ", dir,
"!")};
807 detail::safe_filesystem_entry dir_guard{dir};
809 dir_guard.remove_all();
829 return detail::to_string(
"A valid path for the output directory.");
871 throw validation_error{detail::to_string(
"Value ", cmp,
" did not match the pattern ", pattern,
".")};
880 template <std::ranges::forward_range range_type>
882 requires std::convertible_to<std::ranges::range_value_t<range_type>,
option_value_type const &>
886 std::for_each(v.begin(), v.end(), [&] (
auto cmp) { (*this)(cmp); });
892 return detail::to_string(
"Value must match the pattern '", pattern,
"'.");
913 template <
typename option_value_t>
914 struct default_validator
917 using option_value_type = option_value_t;
920 void operator()(option_value_t
const & )
const noexcept
941 template <val
idator val
idator1_type, val
idator val
idator2_type>
943 requires std::same_as<typename validator1_type::option_value_type, typename validator2_type::option_value_type>
945 class validator_chain_adaptor
954 validator_chain_adaptor() =
delete;
955 validator_chain_adaptor(validator_chain_adaptor
const & pf) =
default;
956 validator_chain_adaptor & operator=(validator_chain_adaptor
const & pf) =
default;
957 validator_chain_adaptor(validator_chain_adaptor &&) =
default;
958 validator_chain_adaptor & operator=(validator_chain_adaptor &&) =
default;
964 validator_chain_adaptor(validator1_type vali1_, validator2_type vali2_) :
969 ~validator_chain_adaptor() =
default;
980 template <
typename cmp_type>
982 requires std::invocable<validator1_type, cmp_type const> && std::invocable<validator2_type, cmp_type const>
993 return detail::to_string(vali1.get_help_page_message(),
" ", vali2.get_help_page_message());
998 validator1_type vali1;
1000 validator2_type vali2;
1032 template <val
idator val
idator1_type, val
idator val
idator2_type>
1034 requires std::same_as<typename std::remove_reference_t<validator1_type>::option_value_type,
1037 auto operator|(validator1_type && vali1, validator2_type && vali2)
1039 return detail::validator_chain_adaptor{std::forward<validator1_type>(vali1),
1040 std::forward<validator2_type>(vali2)};
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:247
virtual void operator()(std::filesystem::path const &file) const override
Tests whether path is does not already exists and is writable.
Definition: validators.hpp:649
Provides parser related exceptions.
std::string option_value_type
Type of values that are tested by validator.
Definition: validators.hpp:854
value_list_validator & operator=(value_list_validator &&)=default
Defaulted.
virtual void operator()(std::filesystem::path const &path) const =0
Tests if the given path is a valid input, respectively output, file or directory.
Provides seqan3::detail::safe_filesystem_entry.
output_file_validator & operator=(output_file_validator &&)=default
Defaulted.
void operator()(range_type const &range) const
Tests whether every element in range lies inside values.
Definition: validators.hpp:241
A validator that checks if a matches a regular expression pattern.
Definition: validators.hpp:851
value_list_validator(value_list_validator &&)=default
Defaulted.
virtual ~file_validator_base()=default
file_validator_base & operator=(file_validator_base &&)=default
Defaulted.
Provides various type traits on generic types.
Adaptations of concepts from the standard library.
output_directory_validator & operator=(output_directory_validator &&)=default
Defaulted.
This header includes C++17 filesystem support and imports it into namespace std::filesystem (independ...
T is_regular_file(T... args)
A validator that checks whether a number is inside a given range.
Definition: validators.hpp:106
regex_validator(std::string const &pattern_)
Constructing from a vector.
Definition: validators.hpp:859
A validator that checks if a given path is a valid output directory.
Definition: validators.hpp:766
Adaptations of algorithms from the Ranges TS.
auto operator|(validator1_type &&vali1, validator2_type &&vali2)
Enables the chaining of validators.
Definition: validators.hpp:1037
~value_list_validator()=default
Defaulted.
T current_exception(T... args)
std::string valid_extensions_help_page_message() const
Returns the information of valid file extensions.
Definition: validators.hpp:448
output_file_validator(output_file_validator const &)=default
Defaulted.
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:890
std::vector< std::string > extensions
Stores the extensions.
Definition: validators.hpp:457
using option_value_type
The type of value on which the validator is called on.
A validator that checks whether a value is inside a list of valid values.
Definition: validators.hpp:177
value_list_validator()=default
Defaulted.
An abstract base class for the file and directory validators.
Definition: validators.hpp:318
auto const move
A view that turns lvalue-references into rvalue-references.
Definition: move.hpp:68
T has_extension(T... args)
T is_directory(T... args)
Provides concepts for core language types and relations that don't have concepts in C++20 (yet).
T throw_with_nested(T... args)
void operator()(option_value_type const &cmp) const
Tests whether cmp lies inside values.
Definition: validators.hpp:226
file_validator_base()=default
Defaulted.
value_list_validator & operator=(value_list_validator const &)=default
Defaulted.
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:29
arithmetic_range_validator(option_value_type const min_, option_value_type const max_)
The constructor.
Definition: validators.hpp:115
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
constexpr ptrdiff_t find_if
Get the index of the first type in a pack that satisfies the given predicate.
Definition: traits.hpp:175
virtual ~output_directory_validator()=default
Virtual Destructor.
typename decltype(detail::front< pack_t... >())::type front
Return the first type from the type pack.
Definition: traits.hpp:240
virtual void operator()(std::filesystem::path const &dir) const override
Tests whether path is writable.
Definition: validators.hpp:793
output_file_validator()
Default constructor.
Definition: validators.hpp:615
option_value_t option_value_type
Type of values that are tested by validator.
Definition: validators.hpp:180
Provides seqan3::views::join.
output_directory_validator()=default
Defaulted.
Adaptations of concepts from the Ranges TS.
T emplace_back(T... args)
Argument parser exception thrown when an argument could not be casted to the according type.
Definition: exceptions.hpp:141
output_directory_validator(output_directory_validator const &)=default
Defaulted.
void operator()(range_type const &v) const
Tests whether every filename in list v matches the pattern.
Definition: validators.hpp:884
virtual ~output_file_validator()=default
Virtual Destructor.
value_list_validator(value_list_validator const &)=default
Defaulted.
SeqAn specific customisations in the standard namespace.
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:145
output_directory_validator & operator=(output_directory_validator const &)=default
Defaulted.
The concept for option validators passed to add_option/positional_option.
output_directory_validator(output_directory_validator &&)=default
Defaulted.
T create_directory(T... args)
void operator()(option_value_type const &cmp) const
Tests whether cmp lies inside [min, max].
Definition: validators.hpp:123
Auxiliary for pretty printing of exception messages.
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:672
A type that satisfies std::is_arithmetic_v<t>.
void operator()(option_value_type const &cmp) const
Tests whether cmp lies inside values.
Definition: validators.hpp:867
void validate_readability(std::filesystem::path const &path) const
Checks if the given path is readable.
Definition: validators.hpp:404
file_validator_base & operator=(file_validator_base const &)=default
Defaulted.
output_file_validator & operator=(output_file_validator const &)=default
Defaulted.
void validate_filename(std::filesystem::path const &path) const
Validates the given filename path based on the specified extensions.
Definition: validators.hpp:366
void operator()(option_value_type const &cmp) const
Validates the value 'cmp' and throws a seqan3::validation_error on failure.
T rethrow_exception(T... args)
Provides various utility functions.
Provides traits for seqan3::type_list.
void operator()(range_type const &range) const
Tests whether every element in range lies inside [min, max].
Definition: validators.hpp:139
A validator that checks if a given path is a valid output file.
Definition: validators.hpp:601
output_file_validator(std::vector< std::string > extensions)
Constructs from a given collection of valid extensions.
Definition: validators.hpp:628
double option_value_type
The type of value that this validator invoked upon.
Definition: validators.hpp:109
std::string get_help_page_message() const
Returns a message that can be appended to the (positional) options help page info.
Definition: validators.hpp:827
void validate_writeability(std::filesystem::path const &path) const
Checks if the given path is writable.
Definition: validators.hpp:432
value_list_validator(range_type rng)
Constructing from a range.
Definition: validators.hpp:201
std::string option_value_type
Type of values that are tested by validator.
Definition: validators.hpp:322
output_file_validator(output_file_validator &&)=default
Defaulted.
void operator()(range_type const &v) const
Tests whether every path in list v passes validation. See operator()(option_value_type const & value)...
Definition: validators.hpp:355