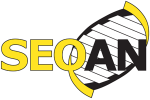 |
SeqAn3
3.0.1
The Modern C++ library for sequence analysis.
|
|
Go to the documentation of this file.
15 #include <type_traits>
30 namespace seqan3::detail
35 SEQAN3_CONCEPT has_value_type = requires {
typename value_type_t<remove_cvref_t<t>>; };
55 template <std::ranges::input_range rng_t>
57 requires !std::input_or_output_iterator<rng_t>
73 template <std::ranges::input_range rng_t>
75 requires !std::input_or_output_iterator<rng_t>
91 template <std::ranges::input_range rng_t>
93 requires !std::input_or_output_iterator<rng_t>
109 template <std::ranges::input_range rng_t>
111 requires !std::input_or_output_iterator<rng_t>
127 template <std::ranges::range rng_t>
129 requires !std::input_or_output_iterator<rng_t>
145 template <std::ranges::sized_range rng_t>
147 requires !std::input_or_output_iterator<rng_t>
152 using type = decltype(
size(std::declval<rng_t &>()));
169 template <
typename t>
171 requires detail::has_value_type<t>
180 template <
typename t>
181 requires detail::has_value_type<t> && detail::has_value_type<value_type_t<remove_cvref_t<t>>>
190 template <
typename t>
207 template <
typename t>
209 requires detail::has_value_type<t>
214 template <
typename t>
215 requires detail::has_value_type<t> && detail::has_value_type<value_type_t<remove_cvref_t<t>>>
216 constexpr
size_t dimension_v<t> = dimension_v<value_type_t<remove_cvref_t<t>>> + 1;
236 template <
typename t1,
typename t2>
240 requires (dimension_v<t1> == dimension_v<t2>);
typename difference_type< t >::type difference_type_t
Shortcut for seqan3::difference_type (transformation_trait shortcut).
Definition: pre.hpp:166
Provides various shortcuts for common std::ranges functions.
Provides various transformation trait base templates and shortcuts.
typename reference< t >::type reference_t
Shortcut for seqan3::reference (transformation_trait shortcut).
Definition: pre.hpp:77
Provides C++20 additions to the <iterator> header.
Provides various type traits on generic types.
rvalue_reference_t< std::ranges::iterator_t< rng_t > > type
Return the rvalue_reference member definition from the queried type's iterator.
Definition: range.hpp:98
Two types are "compatible" if their seqan3::dimension_v and their seqan3::innermost_value_type_t are ...
reference_t< std::ranges::iterator_t< rng_t const > > type
Resolves to the reference type of the const_iterator of t (not the const iterator!...
Definition: range.hpp:116
value_type_t< std::ranges::iterator_t< rng_t > > type
Return the value_type member definition from the queried type's iterator.
Definition: range.hpp:62
Exposes the value_type of another type.
Definition: pre.hpp:41
Recursively determines the value_type on containers and/or iterators.
Definition: range.hpp:173
typename value_type< t >::type value_type_t
Shortcut for seqan3::value_type (transformation_trait shortcut).
Definition: pre.hpp:48
Exposes the difference_type of another type.
Definition: pre.hpp:159
The main SeqAn3 namespace.
Definition: aligned_sequence_concept.hpp:36
value_type_t< remove_cvref_t< t > > type
The return type (recursion not shown).
Definition: range.hpp:176
Provides various transformation traits for use on iterators.
constexpr size_t size
The size of a type pack.
Definition: traits.hpp:116
Exposes the reference of another type.
Definition: pre.hpp:70
Exposes the size_type of another type.
Definition: pre.hpp:188
Exposes the const_reference of another type.
Definition: pre.hpp:130
Adaptations of concepts from the Ranges TS.
constexpr size_t dimension_v
Returns the number of times you can call seqan3::value_type_t recursively on t (type trait).
Definition: range.hpp:211
reference_t< std::ranges::iterator_t< rng_t > > type
Return the reference member definition from the queried type's iterator.
Definition: range.hpp:80
decltype(size(std::declval< rng_t & >())) type
Return the size_type as returned by the size function.
Definition: range.hpp:152
typename innermost_value_type< t >::type innermost_value_type_t
Shortcut for seqan3::innermost_value_type (transformation_trait shortcut).
Definition: range.hpp:191
typename rvalue_reference< t >::type rvalue_reference_t
Shortcut for seqan3::rvalue_reference (transformation_trait shortcut).
Definition: pre.hpp:106
difference_type_t< std::ranges::iterator_t< rng_t > > type
Return the difference_type member definition from the queried type's iterator.
Definition: range.hpp:134
Exposes the rvalue_reference of another type.
Definition: pre.hpp:99